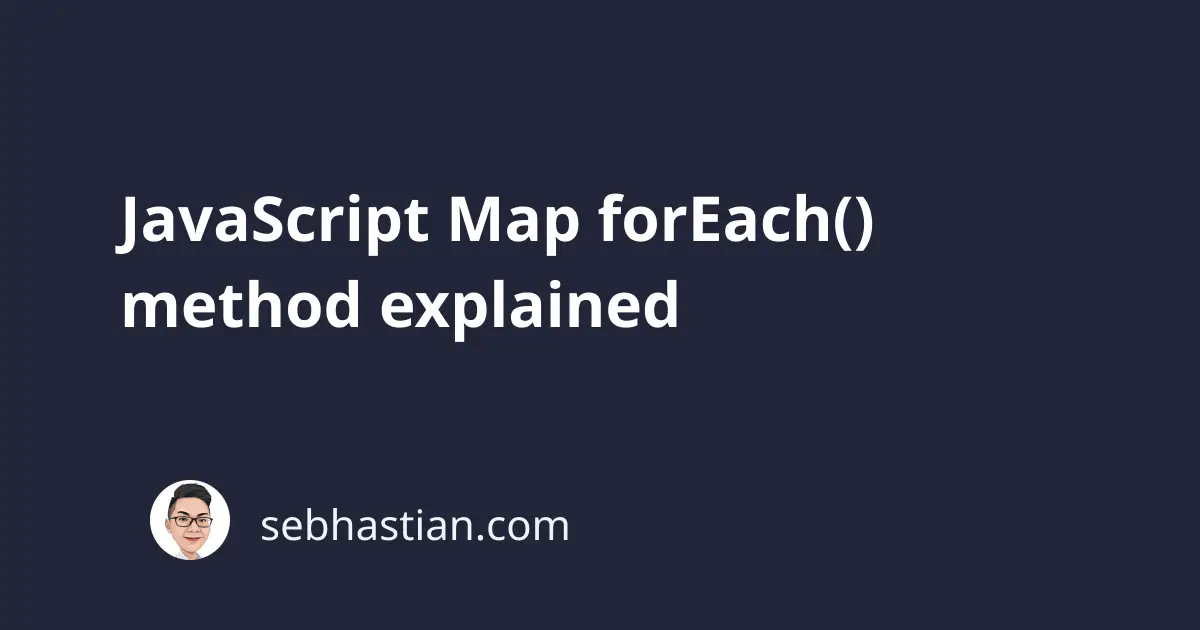
The forEach()
method is used to iterate over a Map
object and executes a callback function once for each pair.
The forEach()
method of the Map
object is similar to the one in the Array
object.
The code below shows how the forEach()
method works:
let map = new Map([
[1, "apple"],
[2, "banana"],
[3, "orange"],
]);
map.forEach(function (value, key, map) {
console.log(`Key: ${key} | Value: ${value}`);
});
/*
Output:
> "Key: 1 | Value: apple"
> "Key: 2 | Value: banana"
> "Key: 3 | Value: orange"
*/
The forEach()
method iterates over each key/value pair in your Map
object in the same order the pair was inserted.
The full syntax of the method is as shown below:
forEach(function (value, key, map) {
/* function body */
}, thisArg);
The method will pass the 3 arguments to the callback function:
value
- The value of the current pair in the iterationkey
- The key of the current pair in the iterationmap
- TheMap
object where you call the method
Additionally, you can also pass a thisArg
argument after the callback function.
The thisArg
argument will be used as the context of the this
keyword inside the callback function body:
const map = new Map([
['a', 'car']
]);
map.forEach(function (value, key, map) {
console.log(this); // John
}, "John");
map.forEach(function (value, key, map) {
console.log(this); // Global object
});
Without passing the thisArg
value, the Global object of the JavaScript environment will be used as the this
keyword context (Window
object for browsers and Global
object for Node. Could also be undefined
in other environments)
You can manipulate the Map
object in each iteration to fulfill the requirement of your code.
And that’s how the forEach()
method of the Map
object works. 👍