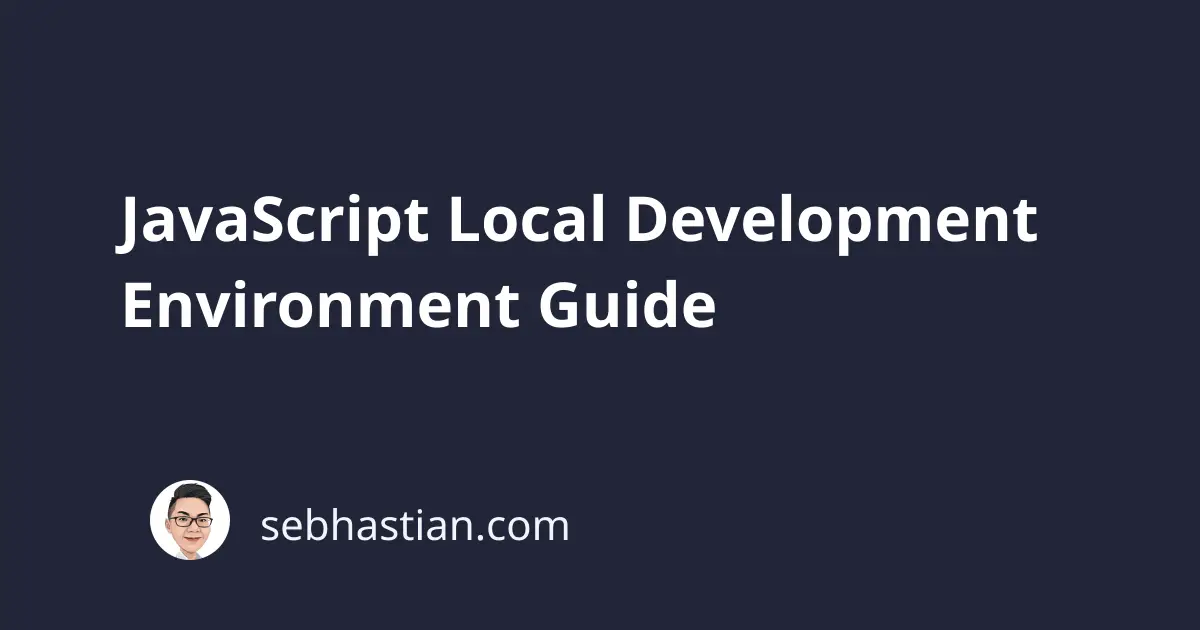
In order to code well, you need to code often. That means you have to be able to run your code in a notebook or PC anytime you wish.
This guide will help you in setting up an environment where you can practice coding in your computer.
Thankfully, JavaScript is a language that first and foremost used in the browser, which means it’s so easy for you to setup a place for practicing JS.
You can download any of your favorite modern browser. Here is the one I recommend ordered by popularity:
I personally only used the first three browser.
Chrome and firefox are core products of the company that make them, and they have the highest priority to improve and update their browser.
Safari is for testing in both Mac and iPhone, and its browser engine, WebKit is kind of lagging behind Chrome in developing new features like Progressive Web App and WebRTC.
My recommendation is simply to develop in Chrome and test in both Chrome and Safari if you use Mac. For Windows or Linux, just use the one you like the most.
Note: I’d still recommend Chrome though, since I used it everyday!
After you’ve downloaded the browser, let’s learn how to code JavaScript inside it’s console. This will be as easy as pressing F12 in your keyboard and click on console tab
Now copy and paste this code into your console:
let firstName = "Nathan",
lastName = "Sebhastian";
console.log (firstName);
console.log (lastName);
And that’s how you run JavaScript in the browser.
Installing Text Editor
A text editor is now an essential part of any development environment.
And lucky for us, text editors are mainly freeware or open source. Two of the most popular text editors are Sublime Text and VSCode.
You don’t need them to follow along the JS Basic tutorial, and if you wish to, you only need to download one of them. Installing text editors are very straightforward. Just follow download the right package for your OS
Installing Node.js
If you desire to be a JS developer, sooner or later you’ll need to install Node on your computer. Luckily installing node is very easy on Windows and MacOS.
Simply visit its website at https://nodejs.org and you’ll be guided to download the right version for your computer. For Linux users particularly Ubuntu, visit their installation guide via package manager at:
https://nodejs.org/en/download/package-manager/#debian-and-ubuntu-based-linux-distributions
After installation, check if node is properly installed in your computer using the terminal command:
$ node -v
v8.11.2 // return the version of installed Node.js
Let’s try to create our first HTTP server in Node.js inside a file named hello_world.js
var http = require('http');
var server = http.createServer();
server.on('request', function(req, res){
res.end('Hello World');
});
server.listen(3000);
You can tell Node to run the server like this:
$ node hello_world.js
Then open your browser and hit http://localhost:3000.
Now you’re ready developing your JavaScript application