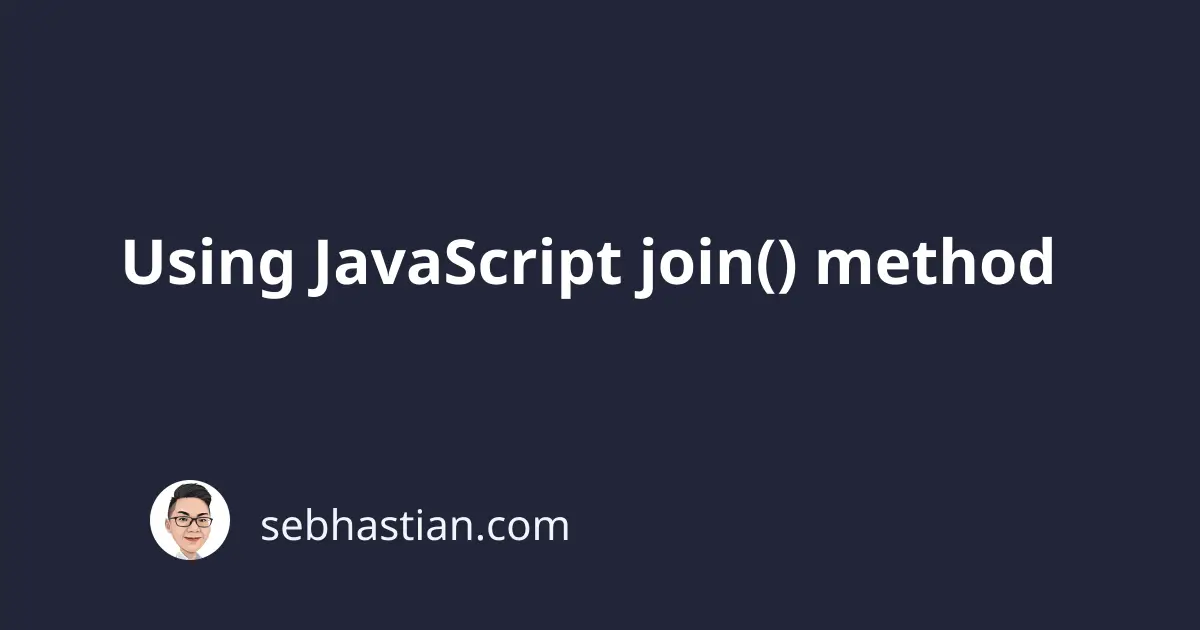
The JavaScript join()
method allows you to concatenate elements of an array into one string
. For example, the following Array
of strings will be joined as a string:
let arr = ["Hello", "World"];
let str = arr.join(" ");
console.log(str); // "Hello World"
The join()
method accepts one string
parameter, that will be used as the separator. Commonly you can use a space character (" "
) or a comma (,
):
let arr = ["Grape", "Banana", "Melon", "Orange"];
let str = arr.join(", ");
console.log(str); // "Grape, Banana, Melon, Orange"
When your array contains numbers, the join()
method will coerce the numbers into a string and concatenate them together:
let arr = [1, 2, 3, 4];
let str = arr.join(", ");
console.log(str); // "1, 2, 3, 4"
Any undefined
or null
value will be transformed into an empty array:
let arr = ["Hello", "World", undefined, null, "!"];
let str = arr.join(" ");
console.log(str); // "Hello World !"