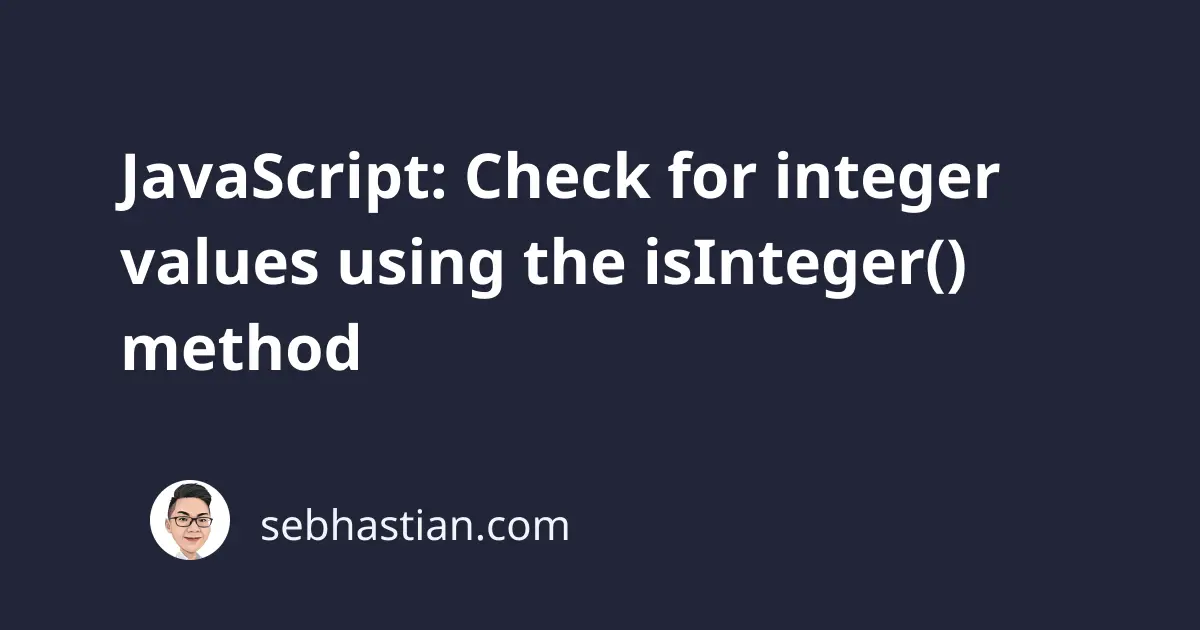
JavaScript Number
object comes with the isInteger()
method that you can use to check if the passed argument is a whole number (integer).
The method accepts one parameter: the value to be tested if it’s an integer.
If the argument is an integer, the method returns true
. Otherwise, it returns false
:
console.log(Number.isInteger(10)); // true
let name = "Nathan";
console.log(Number.isInteger(name)); // false
Any number you passed with floating points will return false
.
So do NaN
, Infinity
, and the boolean values:
let float = 6.873
console.log(Number.isInteger(float)); // false
console.log(Number.isInteger(0.12)); // false
console.log(Number.isInteger(NaN)); // false
console.log(Number.isInteger(Infinity)); // false
console.log(Number.isInteger(true)); // false
console.log(Number.isInteger(false)); // false
The method only returns true
when you pass a whole number as its argument.
Any floating numbers that can be represented as integers will also return true
, such as 1.0
, 2.0
, and so on:
console.log(Number.isInteger(1)); // true
console.log(Number.isInteger(2)); // true
console.log(Number.isInteger(2.0)); // true
console.log(Number.isInteger(59.0)); // true
console.log(Number.isInteger([1, 2, 4])); // false
And that’s how the Number.isInteger()
method works in JavaScript.
You can use this method whenever you need to check if a value is an integer or not.