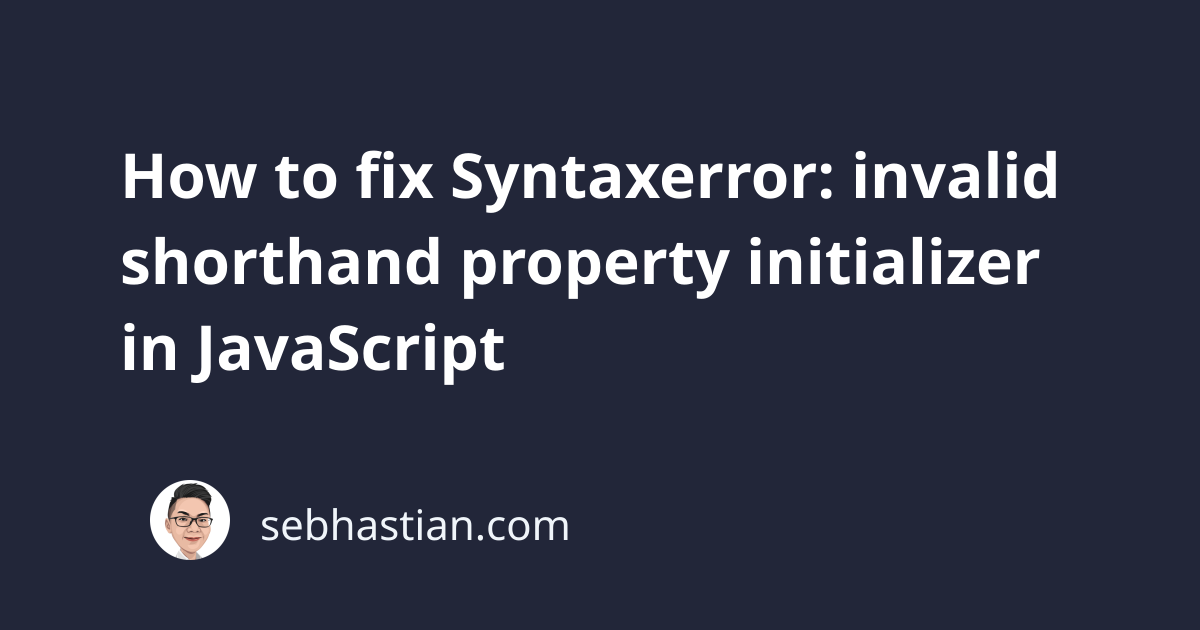
One common JavaScript error that you might encounter is:
Syntaxerror: invalid shorthand property initializer
This error occurs when you use the assignment =
operator to assign a value to a property in your object.
To resolve this error, you need to replace the =
operator with the colon :
operator. Let’s see an example that causes this error and how you can quickly fix it.
Suppose you need to create an object named person
that has two properties: first_name
and last_name
. You then create the object as follows:
const person = {
first_name = 'John',
last_name = 'Wick'
};
When you run the code above, you get this error:
Uncaught SyntaxError: Invalid shorthand property initializer
You can’t use the single equal =
operator when assigning values to a property object. You need to use the colon :
operator instead like this:
const person = {
first_name: 'John',
last_name: 'Wick'
};
console.log(person.first_name); // John
console.log(person.last_name); // Wick
As you can see, the colon :
operator serves as a delimiter between key-value pair in a JavaScript object.
Once you replaced the single equal =
operator with the colon :
operator inside all objects you have in your code, the Syntaxerror: invalid shorthand property initializer
should be resolved.
I hope this tutorial helps. Happy coding and stay awesome! 🙌