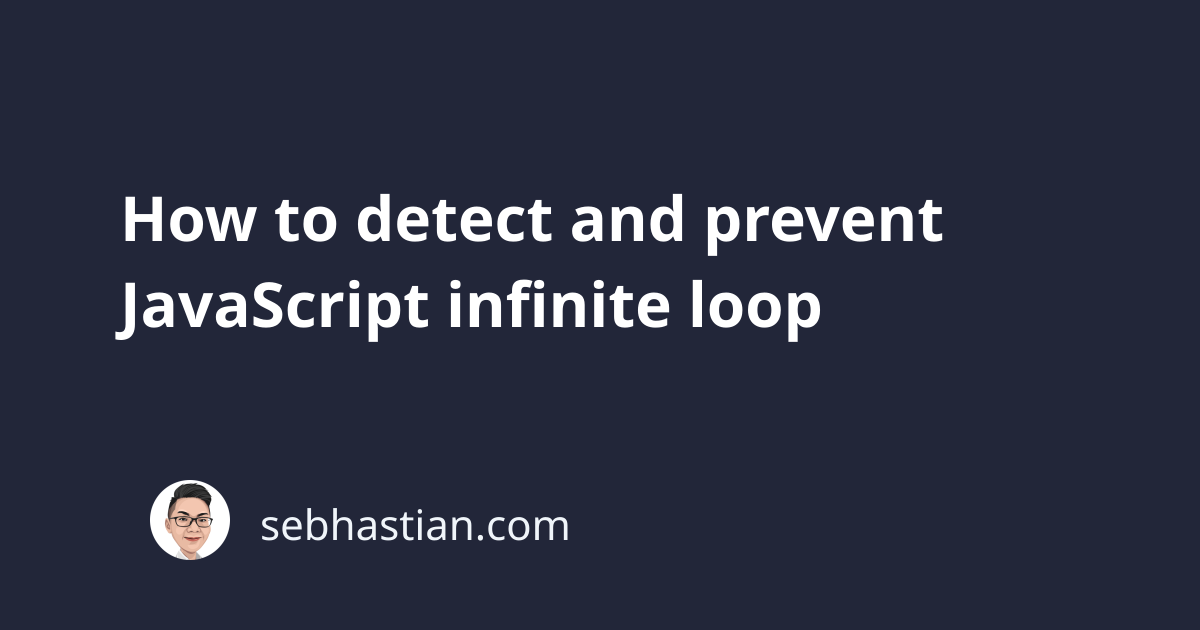
An infinite loop is a condition where a piece of your JavaScript code runs forever caused by a fault in your code that prevents the loop from getting terminated.
An infinite loop is dangerous because it can crash the environment where you run the code (browser or NodeJS server) or freeze your computer, causing it to stop responding.
The for
and while
statements are the common cause of an infinite loop, so this tutorial will help you learn how to detect and fix the infinite loop caused by the statements.
Let’s start with fixing the infinite loop in a for
statement.
Fixing infinite loop in a for statement
A for
statement can cause an infinite loop when you mistakenly put an assignment operator instead of a comparison operator in the second expression (the condition expression)
Here’s an example of a for
statement that will cause an infinite loop. Notice how the second expression is i = 10
:
for (let i = 0; i = 10; i++) {
console.log("Infinite loop");
}
The for
statement requires the second expression evaluates to false
to terminate the loop. In the above example, an assignment operator i = 10
is placed as the condition expression instead of a comparison operator like i < 10
or i > 10
.
Because an assignment operator always evaluates to true
, the for
statement won’t stop printing "Infinite loop"
to the console, which can cause your computer to freeze.
To fix the for
statement above, you need to replace the second expression with something that the for
statement can reach. One example is by using comparison operators (=<
, <
, >
, >=
)
// the second expression is replaced with <
for (let i = 0; i < 10; i++) {
console.log("Infinite loop");
}
There’s also another version of the same mistake. This time, the first expression and the second expression causes the infinite loop:
for (let i = 5; i > 0; i++) {
console.log("Infinite loop");
}
As you can see from the code above, the loop will continue as long as the variable i
is bigger than 0
.
Since the value of i
is already bigger than 0
from the initialization, the second expression will always evaluate to true
, causing an infinite loop.
To fix the code above, the third expression must decrement the value of i
instead of incrementing it:
for (let i = 5; i > 0; i--) {
console.log("Infinite loop");
}
Another example of a for
statement that can cause infinite loop is to omit all three expressions inside the parentheses as follows:
for (;;) {
console.log("Infinite loop");
}
But the above code can only be written intentionally, so you shouldn’t have it in your project unless you want your computer to crash.
While the for
statements you wrote will certainly be more complex than the examples above, you can still use the same principles to find and fix the fault in your statements.
First, you need to make sure that the second expression you put inside your for
statement can actually evaluate as false
.
If the second expression is already correct, start checking on the first and the third expression. Does the first expression initialize a value that always evaluates to true
when the second expression is executed?
Finally, does the third expression correctly increment or decrement the value of the variable initialized in the first expression?
To sum it up: check your second expression first, then check the first and the third expression, in that order.
Next, let’s learn how to fix infinite loop cause by a while
statement.
Fixing infinite loop in a while statement
A while
statement can cause an infinite loop when the condition expression put inside the parentheses always evaluates to true
:
while (true) {
console.log("Infinite loop");
}
To prevent an infinite loop, the condition expression of the while statement must be able to evaluates to false
.
One of the most common mistakes in writing a while
statement is to forget modifying the value of the variable used for the condition expression.
Notice how the value of i
never changes in the example below:
let i = 0;
while (i < 6) {
console.log("Infinite loop");
}
You need to increment the value of i
inside the while
statement body so that the condition expression evaluates to false
as the loop is executed:
let i = 0;
while (i < 6) {
console.log("Infinite loop");
i++;
}
No matter how complex your while
statement is, you need to make sure that the condition expression written inside the parentheses while()
can evaluate to false
.
If you still can’t find what’s causing the infinite loop, then you can use the console.log()
statement to print the value of the variable used by the condition expression:
let i = 0;
while (i < 6) {
console.log("Infinite loop");
i++;
console.log(i);
}
The console log may help you to pinpoint the faulty code line and fix it.