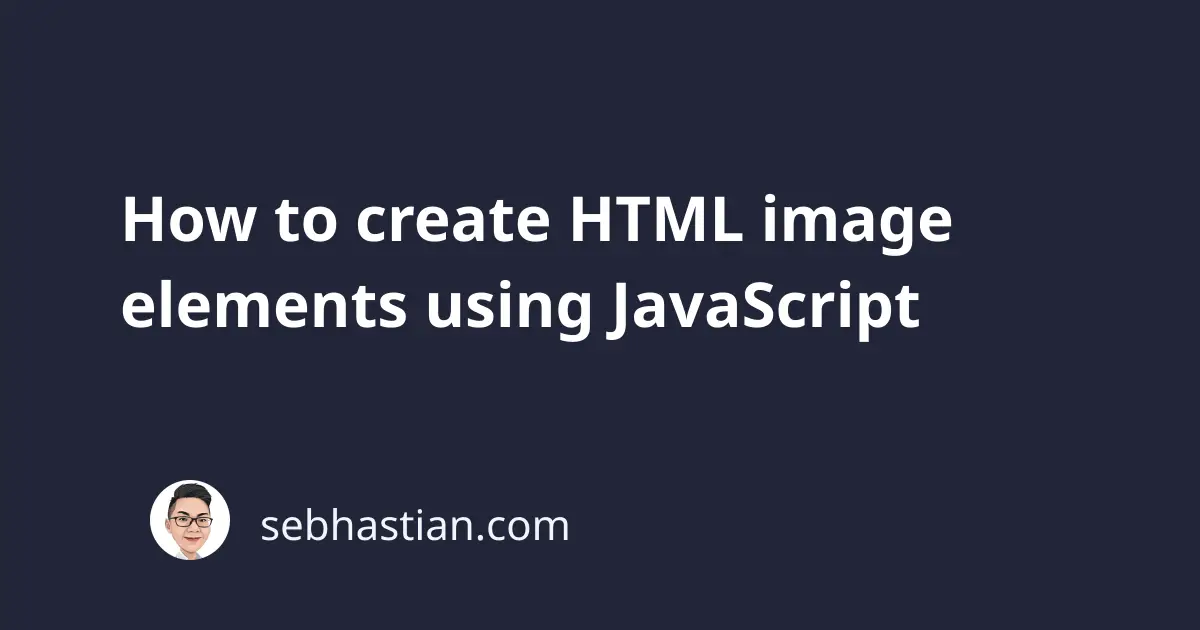
There are two ways you can create an HTML image element using JavaScript:
- Using the
document.createElement('img')
syntax - Using the
Image()
constructor
Let’s learn how to use these two methods to create an HTML image element.
Create image using JavaScript createElement API
The document.createElement()
method is a method of the document
object that allows you to create an instance of HTML elements.
You need to pass the HTML element tag name as an argument to the createElement()
method to create one:
let paragraph = document.createElement("p");
let image = document.createElement("img");
Once you’ve created the element, you can modify its properties like any other HTML element.
After that, you need to append the image to the HTML <body>
tag to make it visible:
let image = document.createElement("img");
image.src = "image.png";
image.width = 300;
image.height = 200;
document.body.appendChild(image);
By appending the image using the document.body.appendChild()
method, you will have the following HTML document structure:
<body>
<img src="image.png" width="300" height="200" />
</body>
And that’s how you create an HTML element using the document.createElement()
method.
Create image using JavaScript Image() constructor
The JavaScript Image()
constructor is used to create an HTML image element, similar to how the document.createElement('img')
syntax works.
To create an image using the constructor, you need to call the constructor as follows:
let image = new Image();
The constructor accepts two optional arguments:
width
for the image’swidth
attributeheight
for the image’sheight
attribute
Here’s an example of creating an image using the Image()
constructor:
let image = new Image(300, 200);
image.src = "image.png";
// <img width="300" height="200" src="image.png">
document.body.appendChild(image);
And that’s how you create an HTML image element using the Image()
constructor.
Keep in mind that the document
object and the Image()
constructor are parts of the Web API. They are not available in other JavaScript environments like NodeJS.
Good work in learning how to create images using JavaScript. 👍