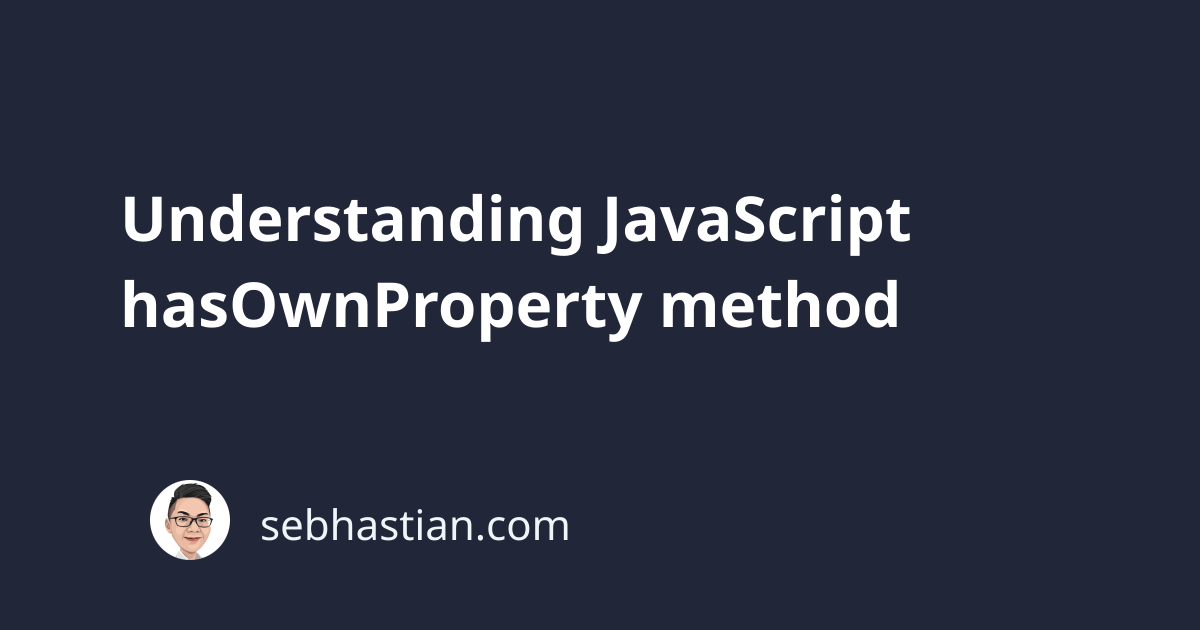
JavaScript can help you to check whether an object actually has a specific property with the hasOwnProperty
method. You can pass a string representing the name of the property as the argument to this method:
let user = {
name: "Jack",
};
user.hasOwnProperty("name"); // true
But then again, can’t you just use the in
operator for checking if an object has a property like this?
let user = {
name: "Jack",
};
console.log("name" in user); // true
While you can do so, the hasOwnProperty
is better than using the in
operator because it helps you to check if the property is actually defined by you instead of being inherited from the prototype.
For example, try to log the user
object defined above into the console:
let user = {
name: "Jack",
};
console.log(user);
You’ll see on the console that there is a list of methods under the __proto__
property, such as toString
and hasOwnProperty
methods:
These methods are inherited from the object prototype, which is inherited by any object you declared in your code. The hasOwnProperty
method will ignore these methods and only check for those that you defined yourself:
let user = {
name: "Jack",
};
console.log(user.hasOwnProperty("hasOwnProperty")); // false
As you see from the code above, the hasOwnProperty
method returns false
when checking on hasOwnProeprty
method even though you are calling on the method itself. The in
operator can’t distinguish between the user-defined method and the inherited method like the above:
let user = {
name: "Jack",
};
console.log("hasOwnProperty" in user); // true
This is why when you need to check whether an object has a specific property, it’s better to use hasOwnProperty
method.