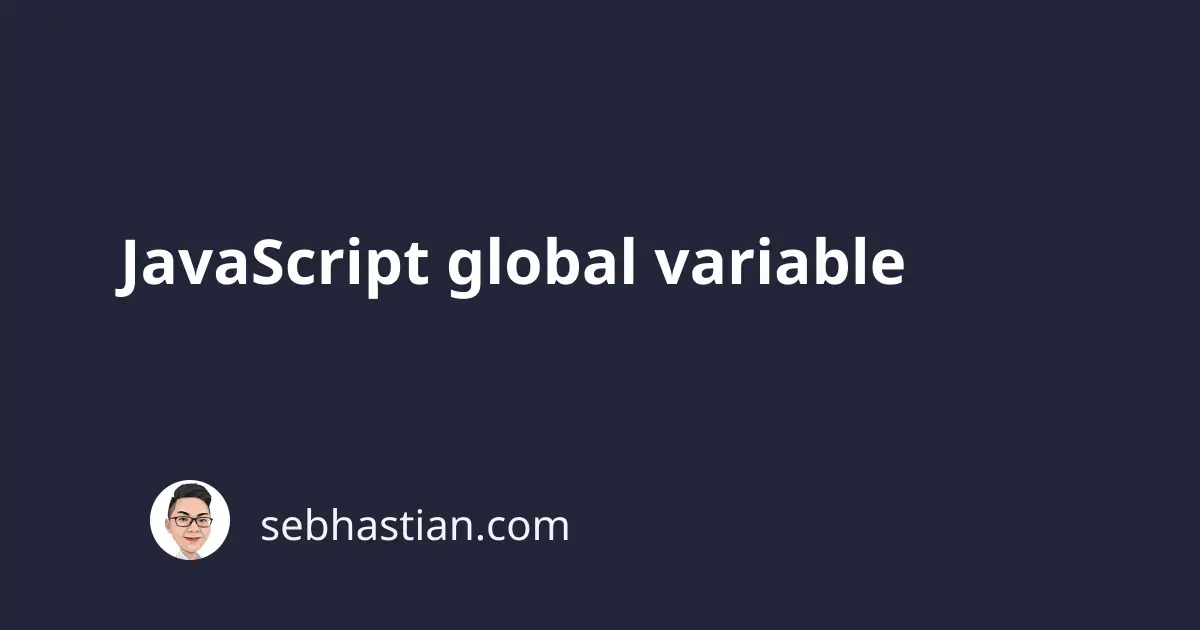
JavaScript global variables are variables that can be accessed from anywhere throughout the lifetime of a JavaScript program.
A variable declared inside a function will be undefined
when called from outside the function:
function getUsername() {
var username = "Jack";
}
getUsername();
console.log(`Outside function : ${username}`);
When you run the code above, JavaScript will throw the ReferenceError: username is not defined
on the console.log()
line. This is because the username
variable scope is limited locally inside the getUsername
function, so it can’t be accessed outside the function.
But when you declare the variable outside of the function, you can log the variable both inside and outside the function:
var username = "Jack";
function getUsername() {
console.log(`Inside function : ${username}`);
}
getUsername();
console.log(`Outside function : ${username}`);
You will see both console.log
can access the username
variable because it’s considered a global variable:
> Inside function : Jack
> Outside function : Jack
Global variables that you declared are kept inside a global object. In the browser, your global variables are automatically assigned as a property of the window
object. You can access the username
variable using username
or window.username
as in the following example:
var username = "Jack";
console.log(`window.username: ${window.username}`);
But this kind of behavior is only triggered when you create a variable using the var
keyword. Variables created with either let
or const
keyword won’t be assigned as a property of the window
object.
let username = "Jack";
console.log(`window.username: ${window.username}`);
// window.username: undefined
Now that you know how to create global variables, let’s talk a bit about whether you should use them or not.
Should you use global variables?
Global variables do make things easier because you can call them from anywhere. But creating global variables are “globally” considered bad by software developers for a reason. So before you set off and create global variables in your code, let me tell you about these reasons.
First, because the variable can be accessed from anywhere, that means any part of your code that calls the variable can change its value. This means it will be hard for you or any other developer to reason about the role played by the variable in your code, which also makes it hard to debug when you find errors in your program.
Global variables also make it difficult for you to test your code separately for obvious reasons. If the variable you need is at line 7 on a different file and your function is at line 2000 of another file, you still need to run these files together, even if they have nothing else in common.
A global variable will exist through the lifetime of your application, occupying memory resources as long as the application is running. If you have thousands of code lines and that global variable is used only in two or three lines, then your global variable will keep that memory occupied when it should be released for other tasks.
If global variables are bad, why do they exist?
Global variables are actually needed by JavaScript so that you can build applications easier. Some global variables are built into JavaScript engines because they are properties and values that are commonly needed almost in every single JavaScript project.
The window
and the global
objects are actually global variables. So does the Math
object.
To conclude, global variables are good only when it’s built into the JavaScript engine. Your code will be easier to understand, debug and control when the scope of the declared variables are limited to the part of code that actually uses it.