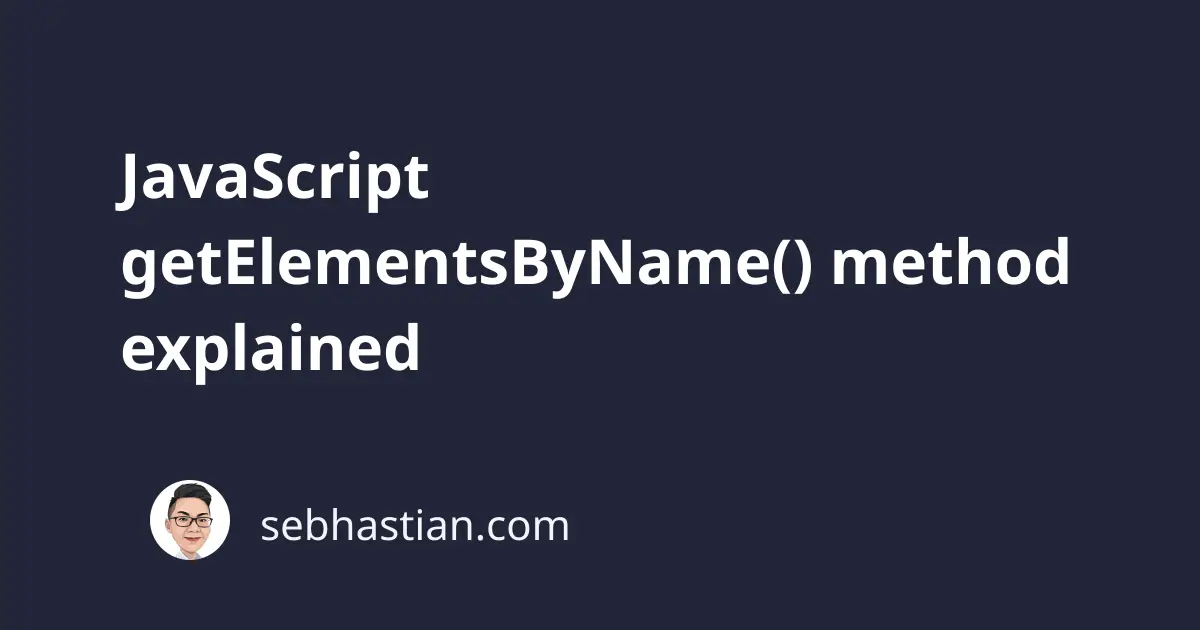
The document.getElementsByName()
method is a built-in method of the DOM API that allows you to select HTML elements by the value of its name
attribute.
The method requires you to pass the name
attribute that you want to search for on your current HTML page.
For example, suppose you have the following HTML page:
<body>
<p name="opening">Opening paragraph</p>
<p name="closing">Closing paragraph</p>
</body>
Here’s how you can select the <p>
element with the name
attribute value of "opening"
:
let elements = document.getElementsByName("opening");
console.log(elements); // [p]
console.log(elements[0]); // <p name="opening">Opening paragraph</p>
The getElementsByName()
method returns an array-like object called HTMLCollection
which stores all elements that matches the value passed as the method’s argument.
This means you can get multiple elements that have the same name
attribute in your HTML page.
Take a look at the following HTML <body>
tag content:
<body>
<p>Web technologies</p>
<ul>
<li name="list">HTML</li>
<li name="list">CSS</li>
<li name="list">JavaScript</li>
<li>PHP</li>
</ul>
</body>
When you use getElementsByName()
method to retrieve the name=list
elements above, the first three <li>
tag will be retrieved by the method and returned as an HTMLCollection
:
let elements = document.getElementsByName("list");
console.log(elements); // [li, li, li]
console.log(elements[0]); // <li name="list">HTML</li>
console.log(elements[1]); // <li name="list">CSS</li>
console.log(elements[2]); // <li name="list">JavaScript</li>
The elements will be ordered from the first element found at the top to the last one at the bottom.
Conversely, the method will return an empty array []
when there is no matching element found in the HTML page.
Keep in mind that even though you can access HTMLCollection
object’s value like an array, the HTMLCollection
object does not inherit methods from JavaScript Array
prototype object.
This means JavaScript Array
methods like forEach()
, map()
, or filter()
can’t be called from an HTMLCollection
object.
If you want to do something with all elements that match your selection, you need to use the document.querySelectorAll()
method.
See Also: querySelectorAll()
method explained
The querySelectorAll()
method returns a NodeList
object that implements the forEach()
method.