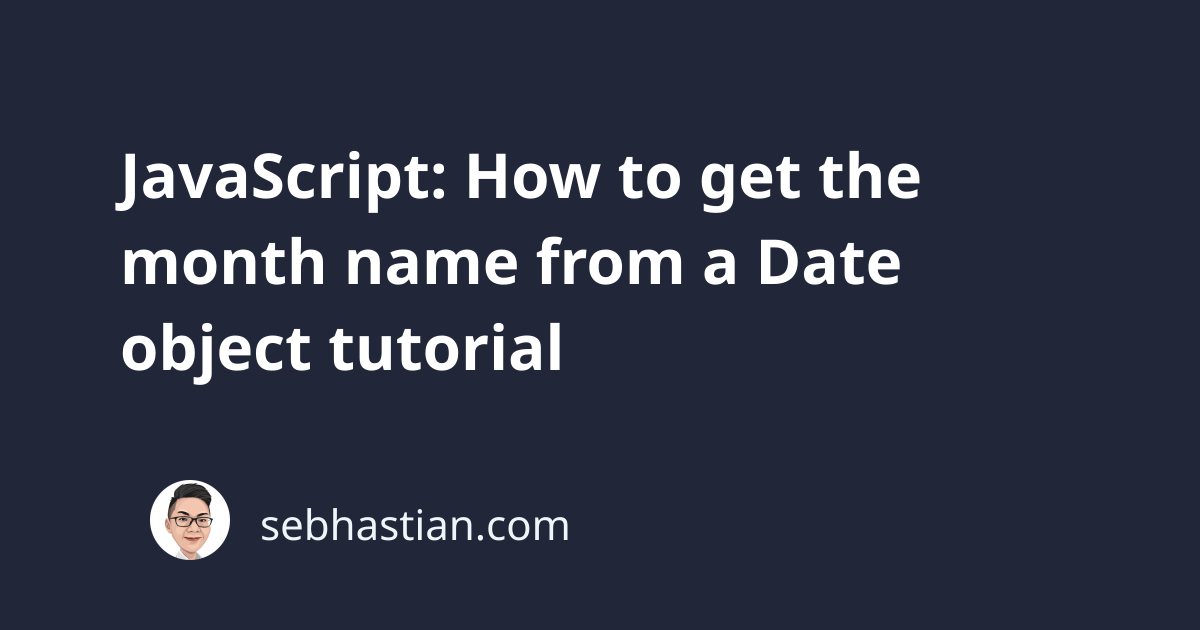
Sometimes, you want to get the month name from a JavaScript Date
object instead of the number representation. For example, you want to get the month name "January"
from the following date:
const date = new Date("01/20/2021"); // 20th January 2021
To get the string "January"
from the date above, you need to use a JavaScript Date
object method named toLocaleString()
which returns a locale-sensitive string
representing the value of Date
object.
The toLocaleString()
method accepts two parameters:
- The
locale
representing the locale-sensitive, could be astring
or anarray
- An
options
object, where you decide what the method will return
For example, here’s how to return the month of the date:
const date = new Date("01/20/2021"); // 20th January 2021
const month = date.toLocaleString('default', { month: 'long' });
console.log(month); // "January"
The 'default'
argument will use the locale currently used by your computer. You may need to use en-US
to get the right month in English. The option { month: 'long' }
will return the full month name. You can get the three letters representing the month using { month: 'short' }
option:
const date = new Date("01/20/2021"); // 20th January 2021
const month = date.toLocaleString('default', { month: 'short' });
console.log(month); // "Jan"
And that’s how you can get the month name from a JavaScript Date
object value.