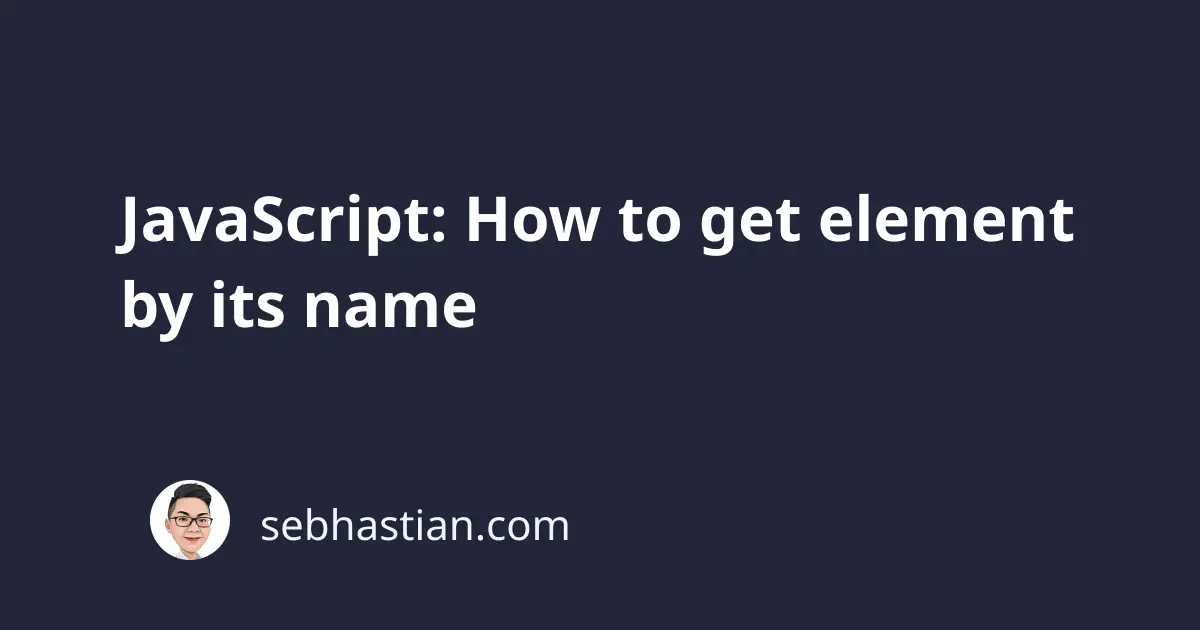
You can use JavaScript to retrieve an HTML element by using its name with the getElementsByName()
method.
This method always return an array of elements with the name
attribute that you specified as its argument.
For example, suppose you have the following HTML <body>
tag:
<body>
<p name="paragraph">Hello world</p>
<p name="paragraph">Bonjour le monde</p>
<p name="paragraph">Olá Mundo</p>
</body>
You can fetch all three <p>
elements using its name as follows:
const paragraphs = document.getElementsByName("paragraph");
console.log(paragraphs); // NodeList [p, p, p]
console.log(paragraphs[0].innerHTML); // Hello world
console.log(paragraphs[1].innerHTML); // Bonjour le monde
console.log(paragraphs[2].innerHTML); // Olá Mundo
When there is no element with the specified name
attribute, the method will simply return an empty array []
.