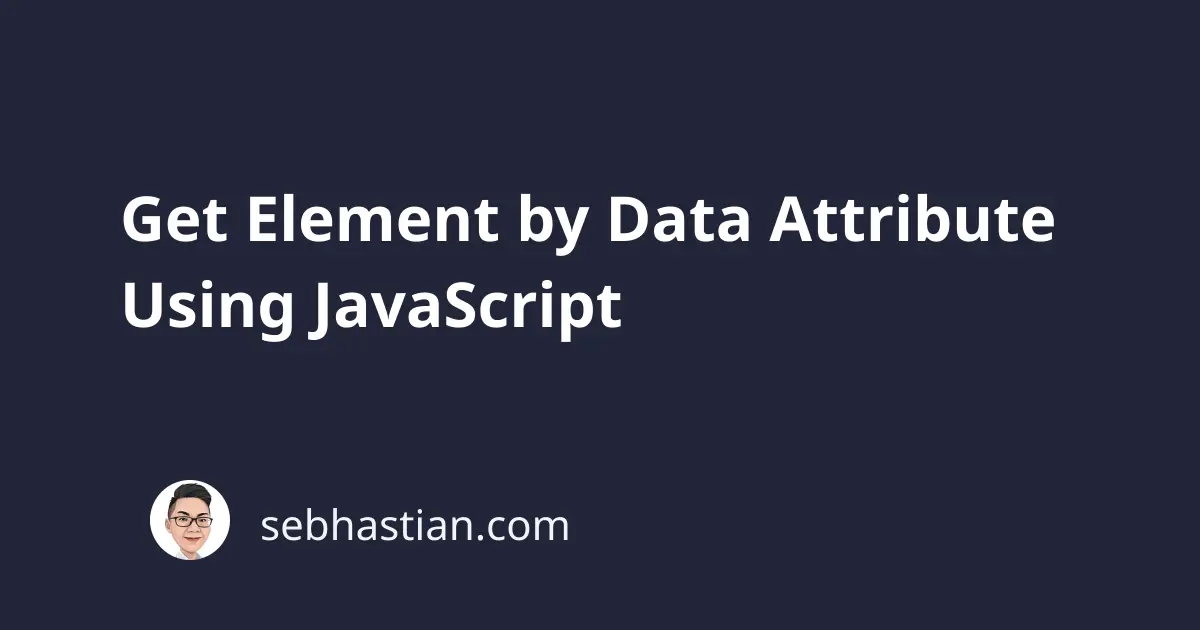
Hello again! In this article, I’m going to show you how to select an element by the value of its data attribute using JavaScript.
To get an element that has a certain data attribute, you need to use the document.querySelector()
method and enter the attribute selector, which is [data-x=""]
For example, suppose you have <div>
elements as shown below:
<div data-value='1'>Hello!</div>
<div data-value='2'>Morning!</div>
To select the <div>
with data-value
of 1, you run the following code:
const myElement = document.querySelector('[data-value="1"]');
console.log(myElement.innerText); // Hello!
If you want to get multiple elements that have the same data attribute, you need to use the document.querySelectorAll()
method instead.
Suppose you have the following elements in your website:
<div data-session='123'>Hello!</div>
<div data-session='123'>Morning!</div>
<div data-session='333'>Night!</div>
Then you can grab the first two elements by using the document.querySelectorAll()
method like this:
const elements = document.querySelectorAll('[data-session="123"]');
Now if you want to select all elements that has the data-session
attribute without filtering the value the attribute has, then you can omit the value in the selector entirely.
The following code will select all three <div>
elements:
const elements = document.querySelectorAll('[data-session]');
The document query selector is very flexible, so you can select any element with any custom data attribute using it.
And that will be all for today. See you in other articles!