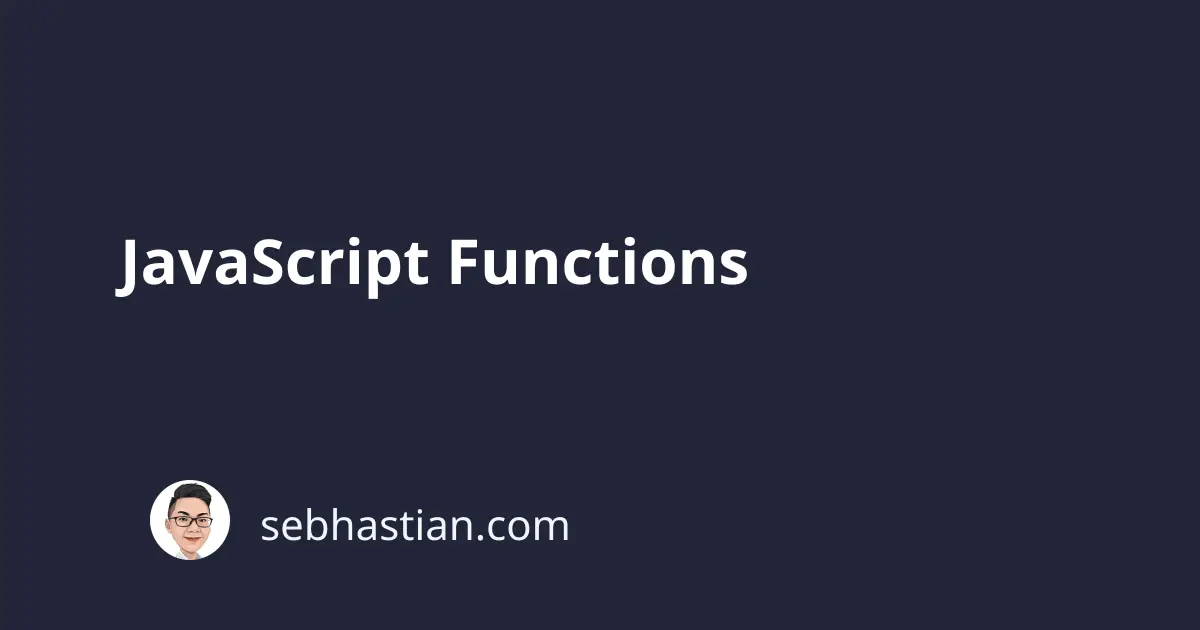
Functions are the main thing you will use very often when coding with JavaScript. They give us a way to structure code in a large program into groups of useful subprograms.
Think of functions as a single unit of code that performs a specific task inside a large code base. Let’s learn all about functions in this article.
Introduction to functions
A function
in programming language creates a new block of code that is defined and self contained. A single function can be run inside your program anytime you want by calling its definition. Functions can also accept parameters that will affect the outcome of its process.
A function declared with the function keyword creates a Function
object that inherits all the properties, methods and behavior of Function objects.
A function without return
statement will return undefined
value. You can put in any type of value into a function return statement. JavaScript’s functions are also first-class functions, which means they can be passed as arguments, assigned to a value and returned as value from another function.
Function Syntax
The syntax to declare a regular function in JS is as follows:
function myFunctionName(firstParameter secondParameter) {
// statement as function body here
}
You can also assign a function into a variable (called function expression)
const myFunctionVariable = function (firstParameter, secondParameter) {
// statement as function body here
};
This is another example where the new ES2015 variable shines.
A const
variable is more suited than let or var for function expression, since a function will not be changed once declared (at least in my 5 years of coding, I’ve seen zero case of it.)
ES2015 also introduced arrow functions, a new way to declare function that has a shorter syntax and especially useful for creating inline functions.
const myFunctionName = (firstParameter) => {
// statement as function body here
};
Parameters or Arguments
You’ve seen some examples of parameter syntax from above, so let me explain a bit. Parameters are dynamic values that must be passed into a function, and the function can then use those parameters in its statement.
There really is no limit to how many parameters a function can have, but I’ve rarely used more than 5 in a single function.
There is definitely something wrong with the way you code if you ever have a dozen parameters in a single function anyway!
const myFunctionName = () => {
// This function has no parameter
};
const myFunctionName = (firstParameter) => {
//This function has one parameter
};
const myFunctionName = (firstParameter, secondParameter) => {
//This function has two parameter
};
If you called a function without assigning values into its parameters, the parameter values will be undefined.
const myFunctionName = (firstParameter, secondParameter) => {
// print the parameters out
console.log(firstParameter);
console.log(secondParameter);
};
myFunctionName("hola", "viva");
myFunctionName("hola");
myFunctionName();
Since ES2015, functions can have default parameters to avoid undefined values.
const myFunctionName = (
firstParameter = "Yo fill this",
secondParameter = "This is empty"
) => {
// print the parameters out
console.log(firstParameter);
console.log(secondParameter);
};
myFunctionName();
Return Values
Every function in JavaScript returns a value, and by default it is undefined. A functions ends when its final line of statement is executed or when a return keyword is run. The return keyword will exit the function execution and return control into its caller.
const myFunctionName = (
firstParameter = "Yo fill this",
secondParameter = "This is empty"
) => {
// print the parameters out
console.log(firstParameter);
return 5; // stop the function and return to its caller, the let number.
console.log(secondParameter);
};
let number = myFunctionName();
console.log(number); // will print the returned 5.
A function can only return a single value. So if you need to return multiple values, you can use an array or object.
const myFunctionName = (
firstParameter = "Yo fill this",
secondParameter = "This is empty"
) => {
let value = [firstParameter, secondParameter];
return value; // return [firstParameter, secondParameter]; also works
console.log(secondParameter);
};
let number = myFunctionName();
console.log(number); // ["Yo fill this", "This is empty"]
Nested Functions
A nested function is blocked from outside of its parent function, thus can’t be called from outside. You can just use inline function for this kind of operation.
const myFunctionName = (
firstParameter = "Yo fill this",
secondParameter = "This is empty"
) => {
const anotherFunction = () => {
console.log("This is from nested function");
};
anotherFunction();
};
myFunctionName();
Function as Object Method
A function can be used as an object property. When it does, it’s called as object method.
const car = {
brand: "Tesla",
type: "Electric",
start: function () {
console.log("Engine started");
},
shutdown: function () {
console.log("Hope you enjoy your ride!");
},
};
car.start();
car.shutdown();
Some Tricky Stuff With JS Functions
The IIFE functions
IIFE stands for Immediately Invoked Function Expression, which means the function will be executed immediately without the need to call it.
(function () {
console.log("Executed. Yeah!");
})();
// assign the result of IIFE into a variable
let theValue = (function () {
return 10;
})();
console.log(theValue);
Hoisting in JavaScript
JavaScript put function and variable declaration into memory before its use, which enabled us as developers to call a function before it’s initialized.
myFunction();
function myFunction() {
console.log("run the function");
}
This kind of code would usually cause error in other programming language, simply because you can’t call a function before you declare that function. But in JavaScript we can, and that is because of hoisting.
Basically, JS will hoist all declaration of functions and variables into memory before the execution began. You can learn more of hoisting here