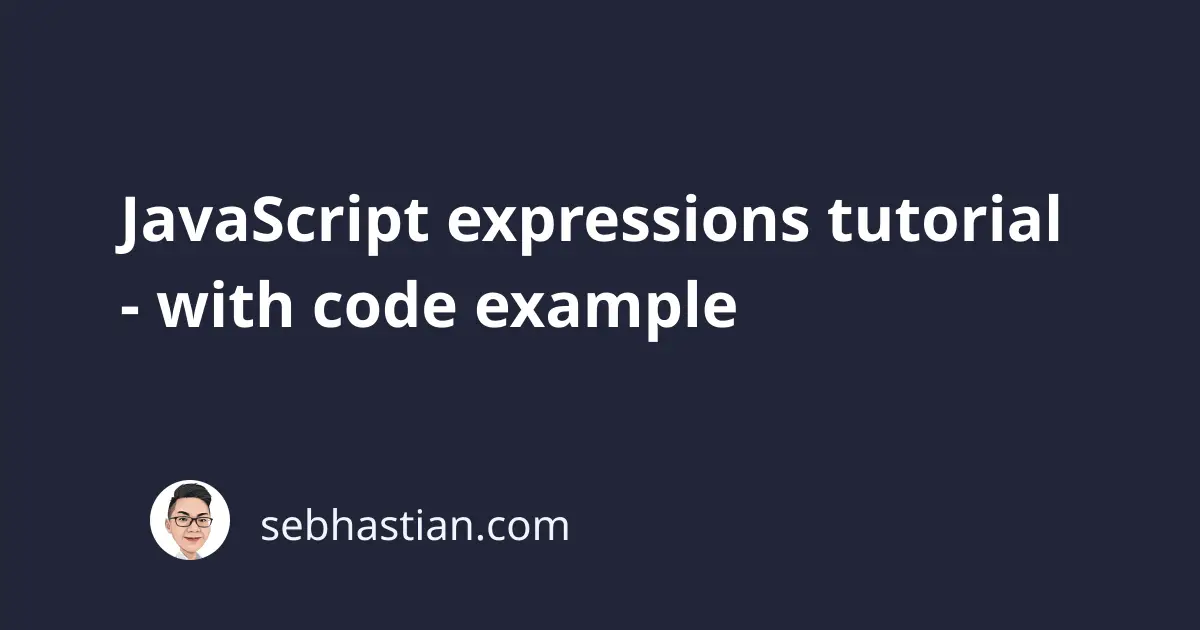
JavaScript expressions are units of code that return a value after execution or computation.
A JavaScript expression can be a combination of literal values, variables, and operators.
The process of computing a JavaScript expression is called evaluation.
For example, here’s how you send a string
expression to the JavaScript console:
> "Hello"
'Hello'
> "This is JavaScript string expression"
'This is JavaScript string expression'
The highlighted lines above are the expression sent to the JavaScript console. The literal string
expressions are evaluated to string
type values returned by the next line.
You can also compose a JavaScript expression using values and operators:
> "Red " + "and" + " Blue"
'Red and Blue'
> 15 + 20
35
> true && false
false
Next, you can also add variables to create more complex expressions.
The code below shows how you can assign a string
value to a variable, then use the variable in another variable:
> let name = "Nathan"
undefined
> let greetings = `Hello! I'm ${name}`
undefined
> greetings
"Hello! I'm Nathan"
As you can see above, variable assignments using var
, let
, and const
keywords evaluate to undefined
.
This is because variable, function, or class definitions are statements. They just declare the definitions for you to use later.
Function statements can return an expression as shown below:
> function sayHi(name) {
return `Hi, ${name}!`;
}
undefined
> sayHi("Jane");
'Hi, Jane!'
You can also create a function expression by assigning a function to a variable.
The following code defines a function expression using the arrow function syntax:
> let sayHi = (name) => `Hi, ${name}!`;
undefined
> sayHi("Lou");
'Hi, Lou!'
When you have a short function, writing the function as an expression can be more convenient than writing it the traditional way using the function
keyword.
Because an expression always evaluates to a value, you can identify a JavaScript expression by looking at the value returned by your code.
Now you’ve learned about JavaScript expressions. I hope this tutorial has been useful for you. 🙏