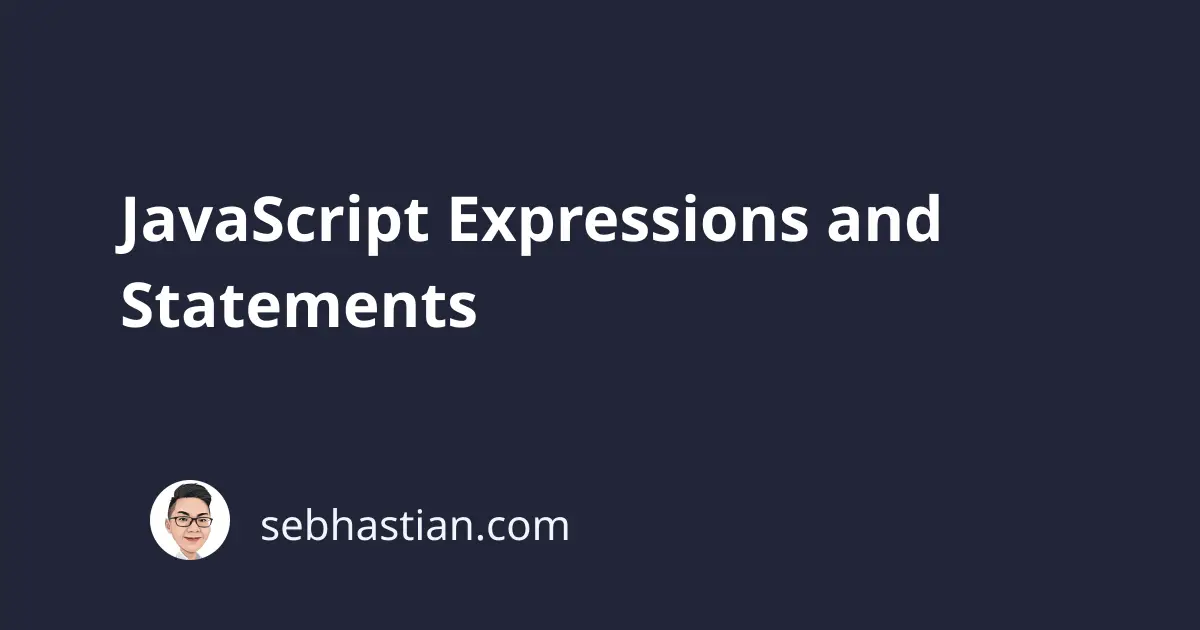
The word statement and expressions confuse me a lot when I first learned programming.
Perhaps it’s because they are the bread and butter of a program’s code structure — just like SOV sentence structure in English — hence makes it difficult to see clearly which is which.
I will try my best to explain what expressions and statements are for you.
Expressions are parts of code that will be evaluate by the programming and returns a new value as a result of that evaluation. It is similar to arithmetical operation, in which we can do addition or subtraction between two numbers or more to create a new number as the result. An easy trick to spot expressions is to look for the operator sign in the code.
A statement, on the other hand, is a complete line of code that performs some action, like print a variable’s value or checking for conditionals. Consider the following piece of code
// first example
let name = "Nathan" + "Sebhastian";
console.log(name);
// second example
let age = 10 + 6;
console.log(age);
// third example
function myFunction(a, b) {
if (a == "Johnny" && b == "English") {
console.log("Welcome, Britain's greatest agent!");
} else {
console.log("Identification failed.");
}
}
myFunction("Johnny", "English");
Here we will do exercise to identify which part of code is a declaration, which is an expression, and which is a statement. Together with declarations, expressions and statements became the building block of code that will be used again and again. Declarations are easy to spot, since the name speaks for itself. We have seen three declarations in the previous code:
// first example
let name = "Nathan" + "Sebhastian"; // this is declaration of variable name
console.log(name);
// second example
let age = 10 + 6; // we declare variable age here
console.log(age);
// third example
function myFunction(a, b) {
// here we declare a function with two parameters
if (a == "Johnny" && b == "English") {
console.log("Welcome, Britain's greatest agent!");
} else {
console.log("Identification failed.");
}
}
myFunction("Johnny", "English");
Now it’s time to identify expressions. Can you spot the part of our code that returns value?
// first example
let name = "Nathan" + "Sebhastian"; // this is declaration of variable name with string concatenation expression
console.log(name);
// second example
let age = 10 + 6; // we declare variable age here with integer addition expression
console.log(age);
// third example
function myFunction(a, b) {
// here we declare a function with two parameters, this is also a function definition expressions
if (a == "Johnny" && b == "English") {
// logical expressions comparing the values of a and b variables
console.log("Welcome, Britain's greatest agent!");
} else {
console.log("Identification failed.");
}
}
myFunction("Johnny", "English"); // this function call is an expression
In this example we see three expressions. The first one is a string expression, the second is arithmetic expression, the third one is a function definition expression, the fourth is a logical expression and the fifth is a function call. Now you began to see why defining which part of code is declaration, expression or statement. Don’t worry, I will provide a cheat sheet at the end for your reference. For now, let’s determine which part of the code are statements.
// first example
let name = "Nathan" + "Sebhastian";
console.log(name); // statement
// second example
let age = 10 + 6;
console.log(age); // statement
// third example
function myFunction(a, b) {
// this entire if else block is a statement
if (a == "Johnny" && b == "English") {
console.log("Welcome, Britain's greatest agent!");
} else {
console.log("Identification failed.");
}
}
myFunction("Johnny", "English");
The easiest way to figure out statements in JavaScript is to look for the semicolon sign ;
Now I know what you think.. “Isn’t that means the entire sample code are statements?” The answer is, unfortunately, yes! Statements are complete lines of code that can have declarations and expressions. You can think of it this way:
// first example
let name = "Nathan" + "Sebhastian"; // the code states that variable name's value is "Nathan" + "Sebhastian"
console.log(name); // statement
// second example
let age = 10 + 6; // this code states that variable age's value is 10+6
console.log(age); // statement
// third example
function myFunction(a, b) {
// this entire if else block is a statement
if (a == "Johnny" && b == "English") {
console.log("Welcome, Britain's greatest agent!");
} else {
console.log("Identification failed.");
}
}
myFunction("Johnny", "English"); // statement that contain function call expression
Statements in a programming language can contain expression. That kind of statement is called expression statement. The reverse however, does not hold: you cannot write a statement where JavaScript expects an expression. For example, an if statement cannot become the argument of a function:
myFunction ("Johnny", if (true){return "English"} ); // unexpected token error
Learning how to identify code parts as declaration, statement or expression takes time and practice. You’ll develop an instinct to identify them as you go along. Now as promised, here is an expression cheat sheet you can use as reference.
Arithmetic Expressions
Expressions that evaluate to a number:
10 / 5;
i++;
i -= 1;
i * 8;
String Expressions
Expressions that evaluate to a string:
"Nathan " + "Sebhastian";
name += "Developer"; // equal to name = name + "Developer";
Primary Expressions
Expressions that evaluate to variable reference, literal value:
10 // integer value
'this is a string' // string value
true // boolean value
this // the current object
undefined // undefined value
name // variable reference
// also keywords to create expressions
function
class
function* //the generator function
yield //the generator pauser/resumer
yield* //delegate to another generator or iterator
async function* //async function expression
await //async function pause/resume/wait for completion
/pattern/i //regex
() // grouping
Array and Object Expressions
These expressions return array or object data type:
[]
{}
[1,2,3]
{a: "Software", b: "Hardware"}
Logical Expressions
These expressions return boolean data type
a && b;
a || !b;
!a;
Logical Expressions
These expressions return boolean data type
a && b;
a || !b;
!a;
Object Creation Expressions
These expressions return an object:
new object();
new myObject(1);
new mySecondObject('name', 2, {a: 4});
Object Property Expressions
These expressions return an object property:
object.property; //reference object property
object[property];
object["property"];
Function Definition Expressions
These expressions return a function:
function() {}
function(a, b) { return a * b }
(a, b) => a + b
a => a / 2