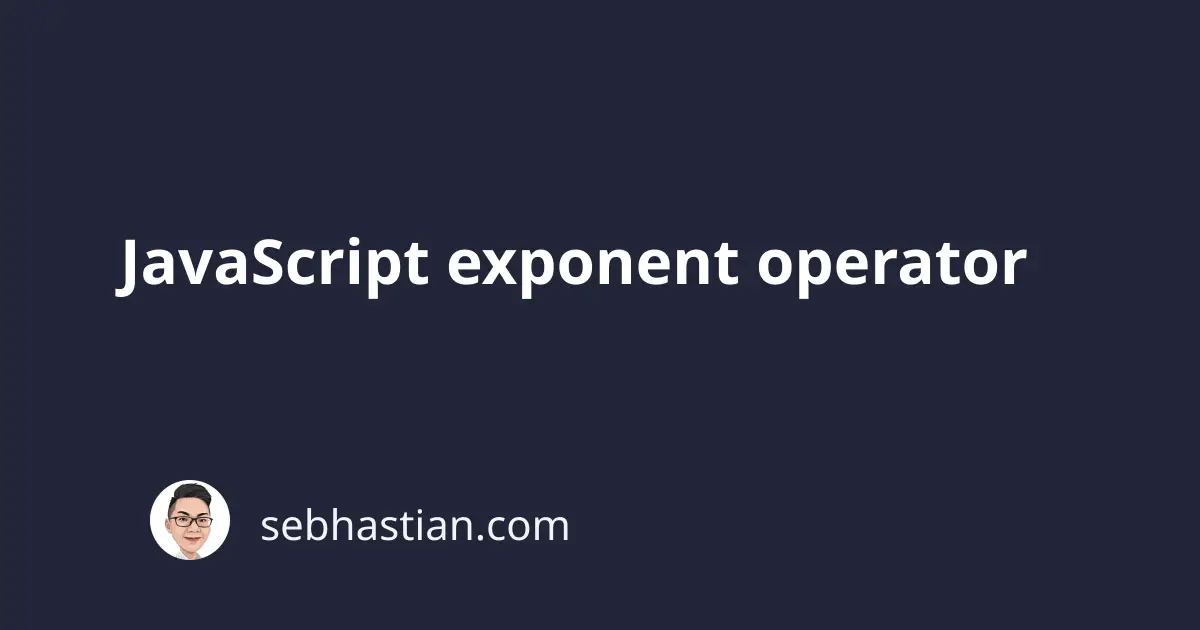
JavaScript comes with an exponentiation operator marked with the double asterisk symbol (**
)
You can add the exponentiation operator after the base number and before the exponent number:
Consider the following code example:
console.log(2 ** 3); // 8
console.log(3 ** 4); // 81
console.log(3 ** -2); // 0.111
The base number is placed on the left side of the operator, while the exponent number is placed on the right side.
The exponentiation operator is equal to the Math.pow()
method, except that it also can run bigint
values.
BigInt("3") ** BigInt("2"); // 9n
Math.pow(BigInt("3"), BigInt("2"));
// Uncaught TypeError:
// Cannot convert a BigInt value to a number
BigInt("3") ** 2
// Uncaught TypeError:
// Cannot mix BigInt and other types, use explicit conversions
The exponentiation operator is right-associative, meaning that without parentheses, JavaScript will work on the right side of the equation first:
2 ** 3 ** 1 // equal to 2 ** (3 ** 1)
2 ** 3 ** 1 ** 4 // equal to 2 ** (3 ** (1 ** 4))
The calculation will proceed from right to left unless you add parentheses as shown below:
(2 ** 3) ** 1 // 2 ** 3 will be calculated first
2 ** (3 ** 1) ** 4 // equal to 2 ** ((3 ** 1) ** 4)
Now you’ve learned how the exponentiation operator works in JavaScript. Good work! 👍