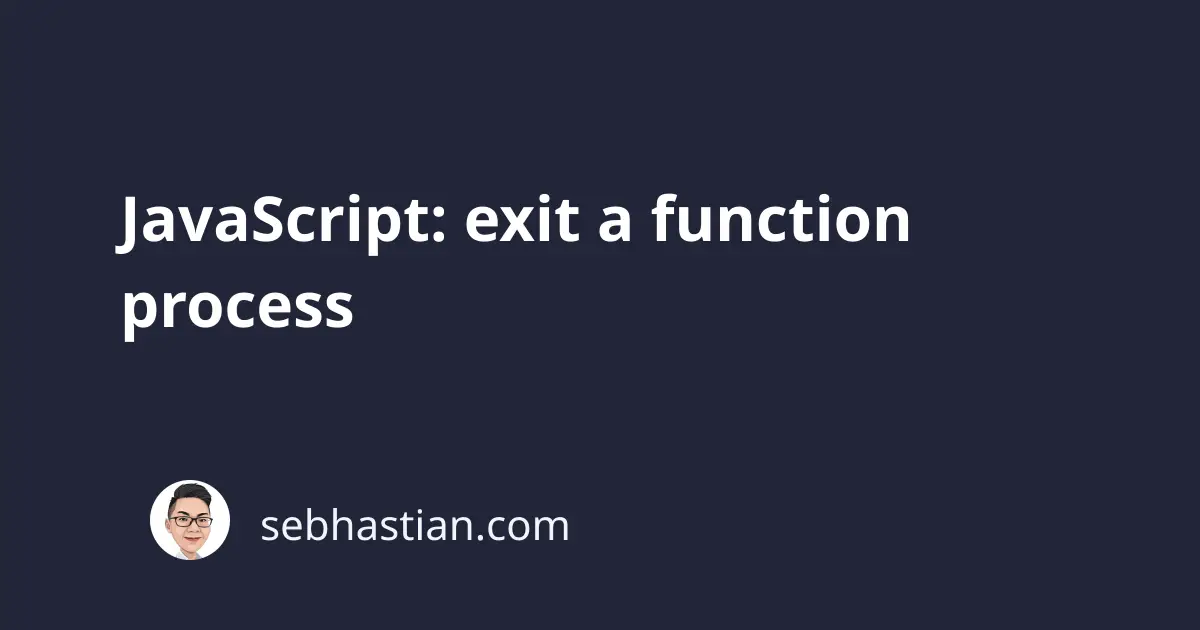
You can use the return
statement to stop and exit a JavaScript function
execution before it reaches the end of the function code block.
Here’s an example:
function hello() {
console.log("Loading...");
return;
console.log("Hello World!");
}
When you run the code above, JavaScript will print "Loading..."
to the console and then return
to the caller context. This will result in "Hello World!"
not being printed to the console.
A JavaScript function
always returns a value. When you don’t explicitly define the return
statement, JavaScript acts there is one at the end of the code block.
When you write a function like this:
function hello() {
console.log("Hello World!");
}
JavaScript will interpret it like the following:
function hello() {
console.log("Hello World!");
return; // becomes undefined
}
Without any value actually returned, JavaScript simply returns undefined to the caller context:
function hello() {
console.log("Hello World!");
}
let value = hello();
console.log(value); // undefined
Specifying exit conditions
Now that you know how to exit a JavaScript function anytime with the return
statement, you can actually specify the exit condition by using an if
statement.
Let’s say that you have a function with a parameter. When the parameter is present, you will print a different sentence and return
function fnTest(param) {
if (param) {
console.log(`Parameter: ${param}`);
return;
}
console.log("You have no parameter");
}
fnTest("a"); // "Parameter: a"
By using the return
statement, you don’t have to put an else
statement to run a different code block.
When to use return statement instead of throw
Now the return
statement is commonly used to exit a function
when you want to run a different code block depending on the passed arguments. It’s not recommended to use return
when you have an exception on your code.
For example, suppose you have a function that requires an x
of number
type. You may write the function like this:
function doubleNumber(x) {
if (typeof x !== "Number" || isNaN(x)) {
console.error ("sorry, x is not a number");
return;
}
console.log((x *= 2));
}
The function above will double the number x
when x
is a number, but logs an error and exit the function earlier without returning any value.
There’s nothing wrong with the code, but the function will simply return undefined
and your code execution will continue down the line. If your code execution relies on the value returned by the function, it’s better for you to stop the execution of your entire script by using the throw
statement.
Take a close look at the if
code block below:
function doubleNumber(x) {
if (typeof x !== "Number" || isNaN(x)) {
throw "sorry, x is not a number";
}
console.log((x *= 2));
}
When you use the throw
statement, the execution of your script will stop because of the error thrown by it. This way, you ensure that code execution only proceeds when there is no exception.
Learn more about throw statement here: JavaScript throw statement guide