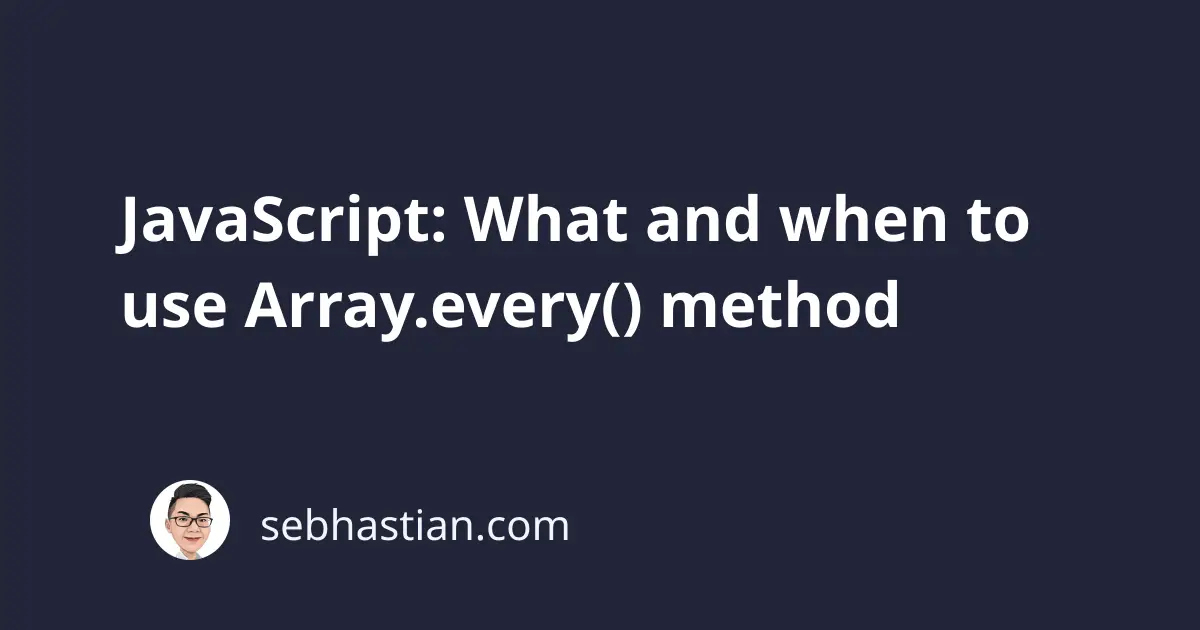
The JavaScript every()
method will check if each one of your Array
elements passes a certain test function that you can define as the callback function.
The every()
method will return either true
or false
as the result of the method call. When you have even one element that doesn’t pass the test, the method will return false
.
Let’s see an example of every()
in action: Suppose you want to make sure that an array of ages
are all above 20
.
Here’s how to find out with every()
:
let ages = [22, 29, 25];
let isAgesAboveTwenty = ages.every((element) => element > 20);
console.log(isAgesAboveTwenty); // true
Again, when you have an element that doesn’t pass the test, every()
will stop the execution and immediately returns false
:
let ages = [17, 29, 25];
let isAgesAboveTwenty = ages.every((element) => {
console.log(element);
element > 20;
});
console.log(isAgesAboveTwenty); // false
The above code will log only 17
and false
to the console. The rest of the elements are not checked again.
JavaScript every()
method is used only when you want to know whether all elements in your array pass a certain test. While you can certainly implement the same test using for
loop or forEach
method, the every()
method is a built-in method that’s readily available for all your Array
type objects.
By using every()
, you reduce the amount of code you need to write in your program.