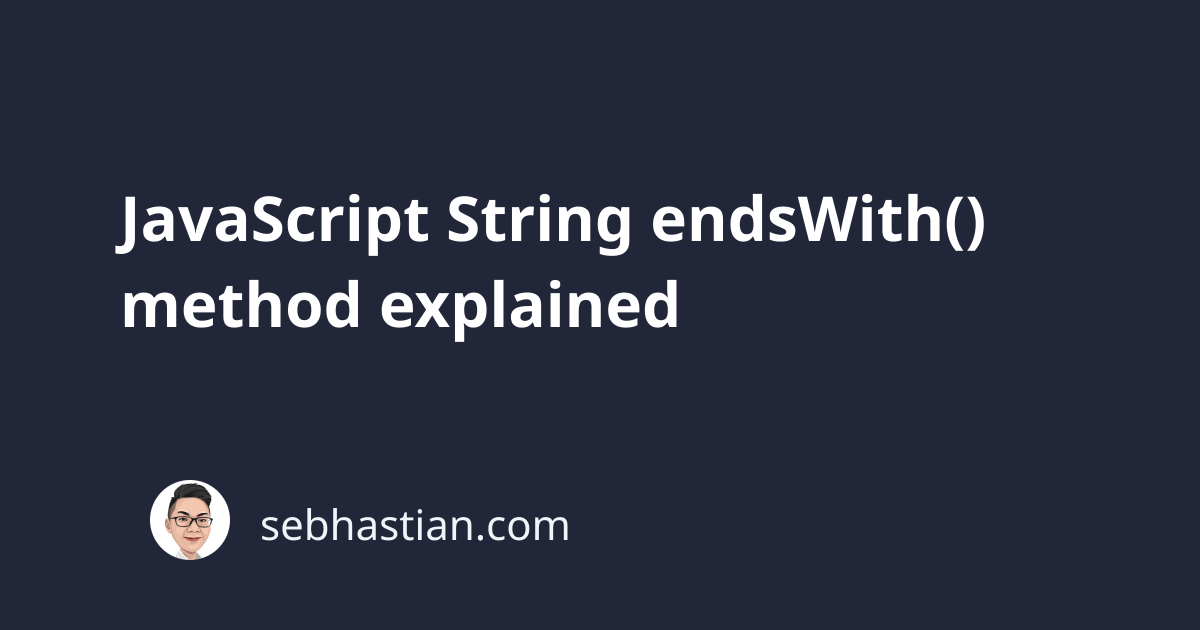
The endsWith()
method is available for JavaScript string to help you check whether a string ends with certain characters. The method accepts two parameters:
- The
string
to search for - required - And the
length
of the string - optional - when specified, the length of the string will be adjusted only for the method operation
endsWith(string, length);
Here’s an example of looking for exclamation mark !
at the end of the string:
let str = "Let's go and have some fun!"
console.log(str.endsWith("!")); // true
You can reduce the length of the string using the second parameter. For example, by passing the number 12
to the code above, the value of str
will be Let's go and
only under the endsWith()
method execution:
let str = "Let's go and have some fun!"
console.log(str.endsWith("and", 12)); // true
Keep in mind that you don’t need to include the complete word for the search. You can also pass only some characters as long as it finishes at the end of the string:
let str = "Let's go and have some fun!"
console.log(str.endsWith("un!")); // true
console.log(str.endsWith("fun")); // false
The search performed is also case sensitive, so make sure that you matched the case correctly:
"Ruby and Emerald".endsWith("emerald"); // false
"Ruby and Emerald".endsWith("Emerald"); // true