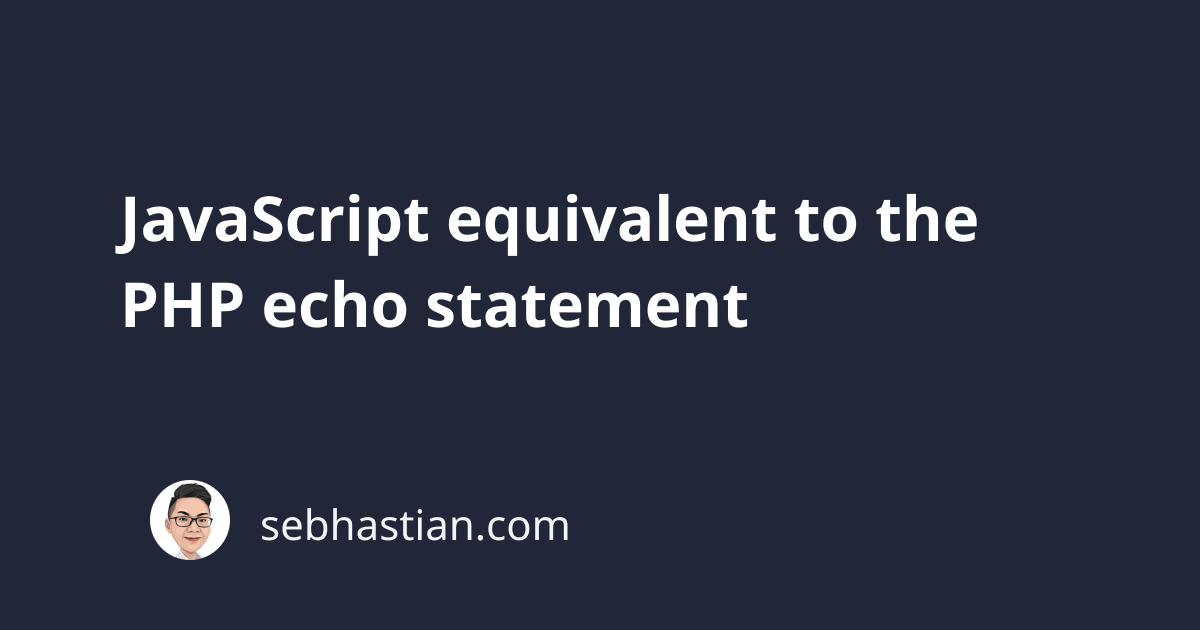
PHP developers tend to look for an equivalent to the echo
statement when they need to display an output to the screen using JavaScript.
Although JavaScript doesn’t have an echo
statement, you can use the document.write()
and the Element.appendChild()
methods to achieve the same result.
This tutorial will show you how to use both methods. Let’s start with learning document.write()
method
Replacing echo with document.write()
One JavaScript method that’s very close to the echo
statement is the document.write()
function, which allows you to display some output to your HTML page during the first render.
Take a look at the JavaScript code below:
<body>
<div>Hello</div>
<script>
document.write("<div>Let's print an additional DIV here</div>");
</script>
<div>World</div>
</body>
The code above will produce similar output to the following PHP code:
<body>
<div>Hello</div>
<?php
echo "<div>Let's print an additional DIV here</div>";
?>
<div>World</div>
</body>
Both examples above will produce the following output:
<body>
<div>Hello</div>
<div>Let's print an additional DIV here</div>
<div>World</div>
</body>
But keep in mind that the document.write()
method will overwrite any content you may have inside the <body>
element after the initial render.
For example, the following code will run the document.write()
method when a button is clicked:
<body>
<div>Hello</div>
<div>World</div>
<button onclick="writeElement()">Echo</button>
<script>
function writeElement() {
document.write("<div>Let's print an additional DIV here</div>");
}
</script>
</body>
When you click on the button, the <body>
element content will be replaced with the value you passed as an argument to the document.write()
method.
The resulting HTML from the button click will a single <div>
element as shown below:
<body>
<div>Let's print an additional DIV here</div>
</body>
To avoid overwriting any element already present inside the <body>
tag, you can use the document.body.appendChild()
method to add an element by the end of the <body>
tag.
Replacing echo with the appendChild() method
The Element.appendChild
method is a built-in method available for all HTML elements that you access using JavaScript. It allows you to add a child element by the end of the element where you call the method.
If you call the appendChild()
method on the <body>
element, then the new element will be placed by the end of the <body>
tag:
// add an element right above the closing </body> tag
document.body.appendChild(newElement);
The method requires you to pass an Element
object instead of a string, so you need to use the document.createElement()
method to create your <div>
element.
The code to add a new element by the end of the <body>
tag is as follows:
let newDiv = document.createElement("div");
newDiv.innerText = "Let's print an additional DIV here";
document.body.appendChild(newDiv);
Now place the code above inside the writeElement()
function:
<body>
<div>Hello</div>
<div>World</div>
<button onclick="writeElement()">Echo</button>
<script>
function writeElement() {
let newDiv = document.createElement("div");
newDiv.innerText = "Let's print an additional DIV here";
document.body.appendChild(newDiv);
}
</script>
</body>
When you click on the button, the newDiv
element will be appended below the <script>
tag as follows:
<body>
<div>Hello</div>
<div>World</div>
<button onclick="echo()">Echo</button>
<script>...</script>
<div>Let's print an additional DIV here</div>
</body>
The two methods above are the closest JavaScript methods you can get to the PHP echo
statement.