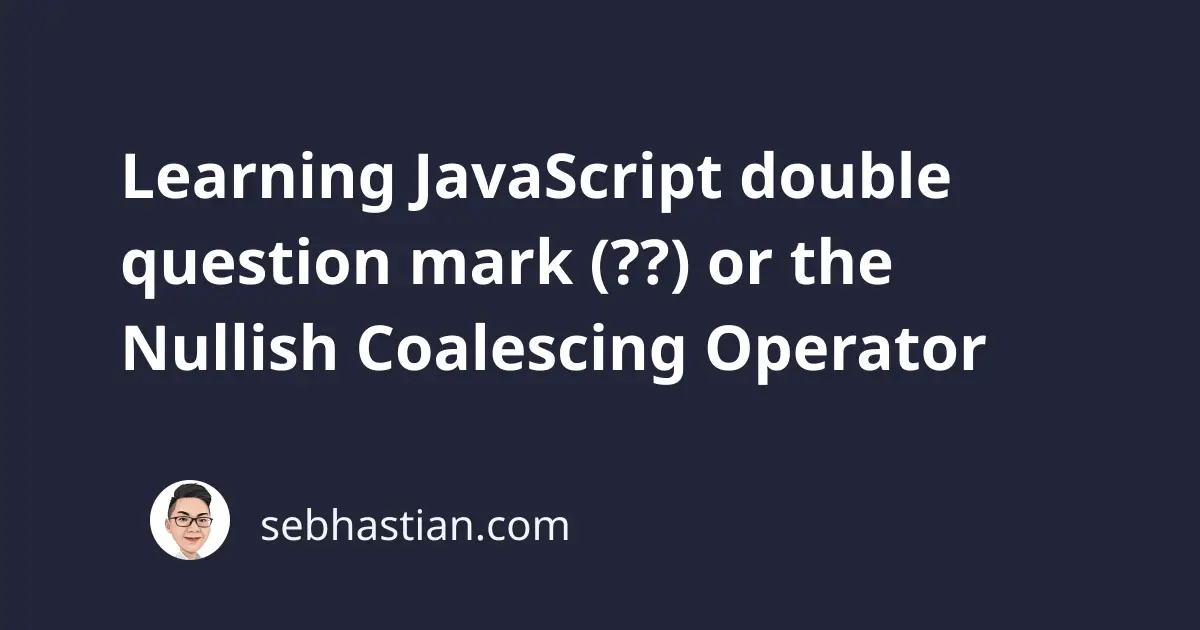
When you’re inspecting JavaScript code, you may find an expression using a double question mark (??
) as in the code below:
alert(username ?? "Guest");
The double question mark is a logical operator that returns the expression on the right-hand of the mark when the expression on the left-hand is null
or undefined
This operator is also known as the Nullish Coalescing Operator. It’s a new feature introduced in JavaScript ES2020 that allows you to check for null
or undefined
values in a more concise way.
Nullish Coalescing Operator syntax
The syntax for the Nullish Coalescing Operator is very simple. It consists of two question marks ??
placed between two expressions.
Here’s an example:
let firstName = null;
let username = firstName ?? "Guest";
console.log(username); // "Guest"
The code above assigns the firstName
variable value as the value of the username
variable.
When the firstName
value is null
or undefined
, then the value Guest
will be assigned instead:
You can also write it this way:
let username = undefined ?? "Guest";
console.log(username); // "Guest"
As you can see, you don’t need an if-else
statement to check for null
or undefined
values.
Why JavaScript needed this operator?
The Nullish Coalescing Operator was created as an improved alternative to the OR operator ||
.
The OR operator was originally created to provide a default or fallback value when the left-hand expression is falsy, or evaluates to false
.
But after some real-world uses, it’s clear that there are times when developers want to return values that are considered falsy, such as 0
and an empty string (""
)
The use of OR operator will prevent you from returning any falsy values at all. Consider the following example:
// empty string evaluates to false in JavaScript:
let firstName = "";
let username = firstName ?? "Guest";
console.log(username); // ""
// When you use OR operator:
username = firstName || "Guest";
console.log(username); // "Guest"
By using the Nullish Coalescing Operator, you will only replace exactly null
and undefined
values with the right-hand value.
The Nullish Coalescing Operator can be used with any type of value, including numbers, strings, and objects.
Several use cases for the Nullish Coalescing Operator
The Nullish Coalescing Operator is useful in a variety of situations where you need to check for null
or undefined
values and provide a default value.
Here are several examples of common use cases:
Handling missing function arguments
When a function is called, it’s possible that some of the arguments may be omitted.
The Nullish Coalescing Operator can be used to provide default values for a missing argument as follows:
function greet(name) {
console.log(`Hello, ${name ?? "Guest"}!`);
}
greet(); // 'Hello, Guest!'
greet("John"); // 'Hello, John!'
Accessing object properties
When working with objects, it’s possible that a property may not exist or is undefined
.
The Nullish Coalescing Operator can be used to safely access object properties and provide a default value when the property is missing:
let user = { name: "John Doe" };
let email = user.email ?? "N/A";
console.log(email); // 'N/A'
Choosing between a variable and a constant
You may want to select a value from a variable or a constant if the variable is null
or undefined
:
let value = null;
const defaultValue = 'Default';
let result = value ?? defaultValue;
console.log(result); // 'Default'
As you can see, the Nullish Coalescing Operator is a great feature that can make your code more concise and reliable.
Using ?? with || and && operators
For safety reasons, the double question mark can’t be used together with JavaScript OR (||
) and AND (&&
) operators without parentheses ()
separating the operators.
For example, the following code tries to see if either firstName
or lastName
variable can be used as the value of username
before using "Guest"
as its value:
let firstName = "John";
let lastName = "Stone";
let username = firstName || lastName ?? "Guest";
// Error: Unexpected token '??'
console.log(username);
This is because JavaScript won’t be able to determine which operator it needs to evaluate first. You need to use parentheses to clearly indicate the priority of the evaluations.
The following code will first evaluate the expressions inside the parentheses:
let firstName = null;
let lastName = undefined;
let username = (firstName || lastName) ?? "Guest";
console.log(username); // "Guest"
And that’s how you combine the Nullish Coalescing Operator with either AND or OR operator.
Conclusion
The JavaScript double question mark is also known as the Nullish Coalescing Operator. It’s a logical operator that simply returns the right-hand expression when the left-hand expression is either null
or undefined
.
The Nullish Coalescing Operator is an improved OR operator that allows you to return 0
and an empty string ""
as a valid value for your application.
This article also shows you a few examples where the Nullish Coalescing Operator is useful.