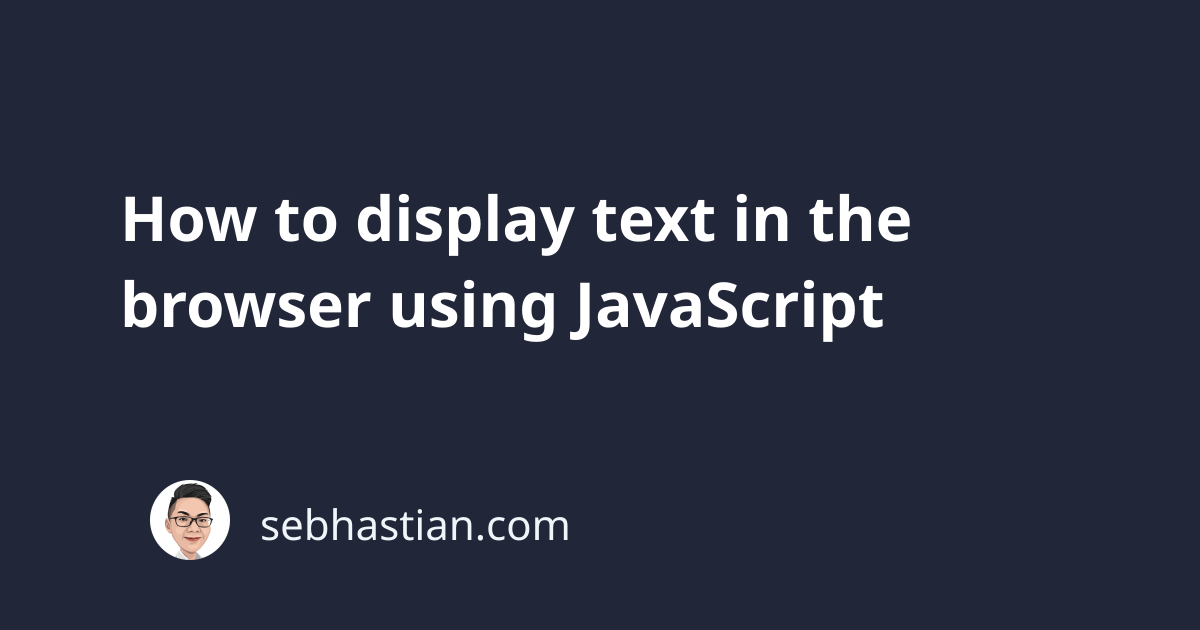
There are four ways to display text in the browser using JavaScript:
- Using the
document.write()
method to write inside the<body>
tag - Using the
document.querySelector()
method to replace the content of a specific element - Using the
console.log()
method to write text output to the browser console - Using the
alert()
method to write text output to the popup box
This tutorial will help you learn them all.
Let’s start with using the document.write()
method.
JavaScript display text using document.write() method
You can display texts in the browser programmatically using JavaScript by manipulating the Document Object Model (DOM)
The Document Object Model is a programming interface that allows you to manipulate the output rendered by the browser. The object itself can be accessed from the document
variable.
For example, the document.write()
method allows you to replace everything inside the HTML <body>
element.
Suppose you have the following HTML <body>
element content:
<body>
<h1>JavaScript Display Text</h1>
<h2>Learn how to display JavaScript text in the browser</h2>
<p>Hello World</p>
</body>
You can call the document.write()
method and pass the message you want to be displayed in the browser as its argument:
document.write("My message");
The HTML <body>
will be replaced as follows:
<body>
My message
</body>
The document.write()
method rewrites everything inside the <body>
tag.
Alternatively, you can use the document.querySelector()
method to select an HTML tag that’s present on your browser page.
JavaScript display text using document.querySelector() method
The querySelector()
method allows you to retrieve a single element that match the query parameter passed to the method.
For example, to replace the <h1>
element content, you can select the element and assign a new value to its innerText
or innerHTML
property as follows:
let header = document.querySelector("h1");
header.innerText = "My message";
// or
header.innerHTML = "My message";
The resulting HTML <body>
content is as follows:
<body>
<h1>My message</h1>
<h2>Learn how to display JavaScript text in the browser</h2>
<p>Hello World</p>
</body>
You can learn more about the querySelector()
method here: JavaScript querySelector() method explained
Aside from in the browser page, you can also display text output in the browser console using JavaScript. Let’s learn about that next.
JavaScript display text using console.log() method
Every web browser has a developer console panel that you can use to write JavaScript code and manipulate the DOM API.
For example, you can access Chrome browser’s console panel, Press Command+Option+J
(Mac) or Control+Shift+J
(Windows, Linux, Chrome OS).
The console looks like this:
From there, you can write the following code:
console.log("Hello World!");
And see the console logs the text as shown below:
The console.log()
method allows you to write output to the console by passing the text as its argument.
JavaScript display text using alert() method
The alert()
method is a method from the browser’s Window
object that allows you to create a popup box with a message.
You can call the alert()
method, and pass the text you want to display as its argument. Since you’ve learned how to open the developer console panel from the previous section, let’s test the alert()
method from the console:
alert("Hi, this is an alert box");
The output will be as shown below:
And those are the four ways that you can use to display a text programmatically using JavaScript.