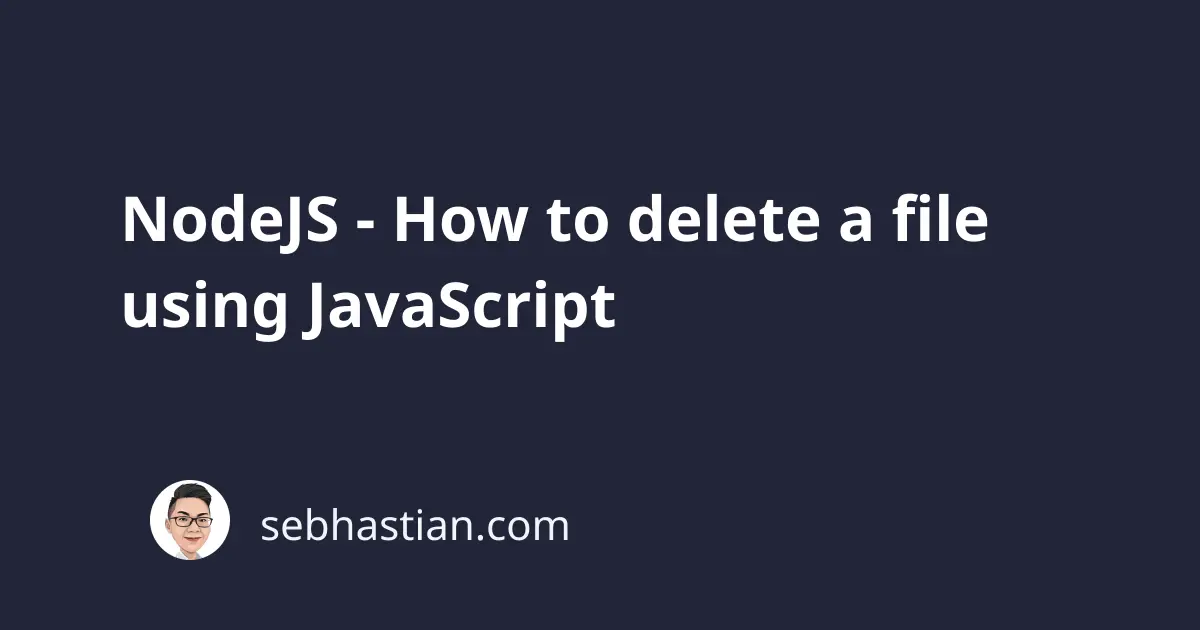
You can’t delete a file when running JavaScript from the browser, but you can do it when running JavaScript from a server environment like NodeJS.
When you need to delete a file using NodeJS, You can use the fs.unlink()
or fs.unlinkSync()
method. This tutorial will show you how to use both methods to delete a file in NodeJS.
The unlink()
and unlinkSync()
method is provided by fs
module, which is short for the file system. First, you need to import fs
module with require()
as follows:
const fs = require("fs");
Then you can start using the methods to delete your file. Let’s start with learning unlinkSync()
method.
Delete a file using unlinkSync() method
The unlinkSync()
method will delete a file synchronously, which means JavaScript code execution will stop until the method finished running.
Suppose you have a JavaScript project folder as follows:
project
├── picture.jpg
└── index.js
You can delete the picture.jpg
file by using the following JavaScript code:
const fs = require("fs");
const path = "./picture.jpg";
try {
fs.unlinkSync(path);
console.log("File removed:", path);
} catch (err) {
console.error(err);
}
Put the code above inside index.js
file, then run the file with node index.js
command.
The code above will delete the picture.jpg
file and log to the console that the file has been removed.
The code is surrounded with a try..catch
block so that a log will be printed when the file can’t be deleted for any reason (No delete permission, file not found, etc)
Next, let’s look at the unlink()
method.
Delete a file using unlink() method
The fs.unlink()
method will delete a file in your filesystem asynchronously, which means JavaScript code execution will continue without waiting for the method to finish.
To use the method you need to pass two parameters:
- The
path
string which contains the path to the file that you want to delete - The
callback
function that will be executed once the function finished running. Theerror
object will be passed to this function by the method.
For example, the code below will delete a picture.jpg
file in the same folder where the script is called from:
const fs = require("fs");
const path = "./picture.jpg";
fs.unlink(path, function (err) {
if (err) {
console.error(err);
} else {
console.log("File removed:", path);
}
});
The unlink()
method doesn’t need to be wrapped in a try..catch
block because whenever the method encounters an error, that error will be caught and passed to the callback
function, as shown above.
And that’s how you can use JavaScript to delete a file. Keep in mind that deleting files is only possible when using JavaScript in a server environment like NodeJS. You can’t delete a file when running JavaScript from the browser.