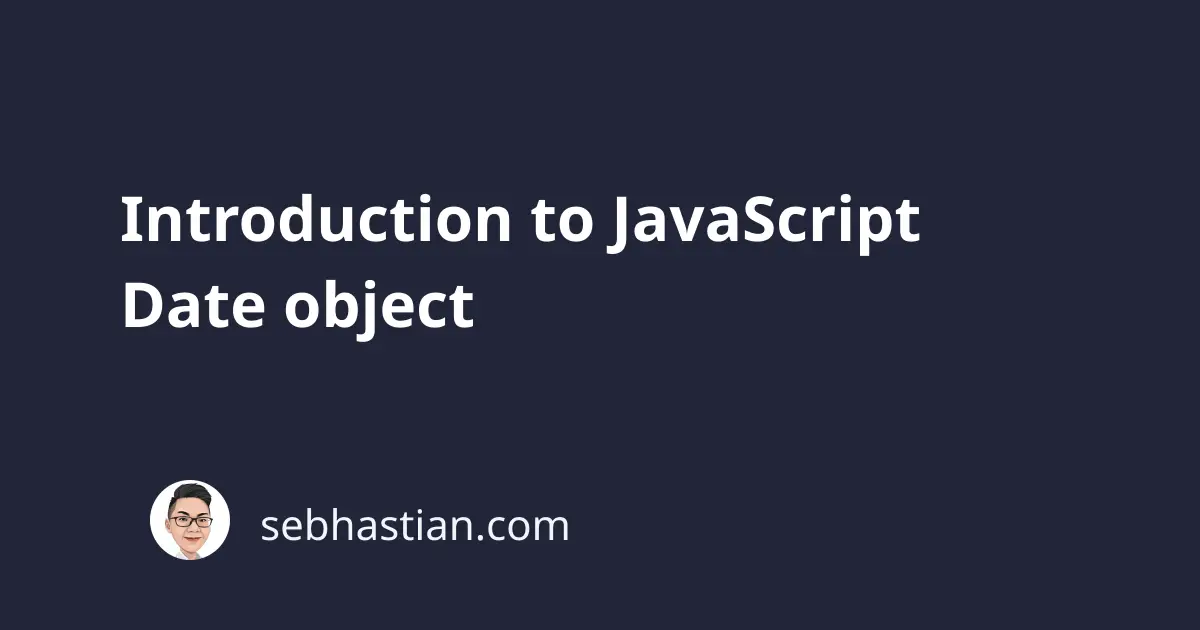
JavaScript’s built-in Date
object enables you to create and store the date and time. It also provides you with methods to manipulate created date and time objects.
You can use the new Date()
construct to create a new Date object showing the current date and time:
let nowDate = new Date(); // date and time in your local timezone
console.log(nowDate); // shows current date and time
The date and time created by new Date()
will always follow the timezone used by the JavaScript engine. Usually, it follows your computer’s timezone.
JavaScript Date
object works by internally calculating the amount of milliseconds that have passed since Epoch time (January 1, 1970 00:00:00 in the UTC timezone).
You can see this when you use the Date.now()
method:
let nowInMs = Date.now();
console.log(nowInMs); // current milliseconds passed since January 1 UTC
Any JavaScript environment knows how to interpret these Date
objects, so you can pass it around from Node.js to the browser and conversely from the browser to Node.js.
Aside from creating the current date and time with new Date()
, there are three ways you can create a specific date and time in JavaScript:
- Using the date
string
- Using milliseconds
- Using the 7 parameters of date and time object
First, using a date string
as follows:
let newDate = new Date("January 17, 2021");
console.log(newDate); // Sun Jan 17 2021 00:00:00 in your timezone
let dateWithTime = new Date("January 17, 2021 09:15:30");
console.log(dateWithTime); // Sun Jan 17 2021 09:15:30 in your timezone
Second, using the milliseconds as follows:
let ms = 1613542151911; // Wed Feb 17 2021 06:09:11 UTC+0
let dateFromMs = new Date(ms);
console.log(dateFromMs); // time will be adjusted to your timezone
When using milliseconds, the time format will be in UTC+0, so your new Date
object will be adjusted forward or backward according to your timezone.
Third, you can use the 7 parameters of the Date
construct in the following order:
new Date(year, month, day, hour, minute, second, millisecond);
Keep in mind that Date
counts the month from zero. That means you need to use 0
for January, 1
for February, and so on. For example:
let date = new Date(2021, 1, 10, 7, 30, 5, 2);
console.log(date); // Wed Feb 10 2021 07:30:05 in your timezone
When you pass only one argument, JavaScript will consider that to be milliseconds
:
let date = new Date(2021);
console.log(date); // Thu Jan 01 1970 07:00:02
You need to at least pass two arguments for the year
and month
to create the proper Date
object:
let date = new Date(2021, 01);
console.log(date); // Mon Feb 01 2021 00:00:00
You can omit the parameters from day
to the millisecond
and use only the year
and month
parameters.
Manipulating date and time
JavaScript Date
object provides you with methods to get and set your date in a certain format.
JavaScript methods you can use to retrieve your date includes:
getFullYear()
returns the year in 4 digits formatgetMonth()
returns the month from0
to11
, with0
representing JanuarygetDate()
returns the date from1
to31
getDay()
returns the day of the week, from0
to6
, with0
representing SundaygetHours()
returns the hour from0
to23
getMinutes()
returns the minute from0
to59
getSeconds()
returns the second from0
to59
getMilliseconds()
returns the second from0
to999
getTime()
returns the milliseconds since Epoch time, likeDate.now()
JavaScript also has the corresponding set methods for Date
object as follows:
setFullYear()
returns the year in 4 digits formatsetMonth()
returns the month from0
to11
, with0
representing JanuarysetDate()
returns the date from1
to31
setDay()
returns the day of the week, from0
to6
, with0
representing SundaysetHours()
returns the hour from0
to23
setMinutes()
returns the minute from0
to59
setSeconds()
returns the second from0
to59
setMilliseconds()
returns the second from0
to999
setTime()
returns the milliseconds since Epoch time, likeDate.now()
Date.parse() method
The Date.parse() method allows you to return the milliseconds from a date string, similar to the Date.now()
method.
The standard format for the date string is YYYY-MM-DDTHH:mm:ss.sssZ
which broken down as follows:
YYYY-MM-DD
– is for the date format: year in 4 digits, while month and date in 2 digits- The character
T
separates the date from time. HH:mm:ss.sss
– is for the time format: hours, minutes, seconds and milliseconds.- The optional
Z
part denotes the time zone for the date. A single letter Z would mean UTC+0. You can use the+00:00
or-00:00
for the timezone
Here’s an example:
let dateA = Date.parse("2019-01-01T00:00:00.000Z");
let dateB = Date.parse("2019-01-01T00:00:00.000+03:00");
console.log(dateA); // 1546300800000
console.log(dateB); // 1546290000000
When you pass an invalid date format, the method will return NaN
:
let dateA = Date.parse("2021-25-23'");
console.log(dateA); // NaN
That will be all about JavaScript Date
object.