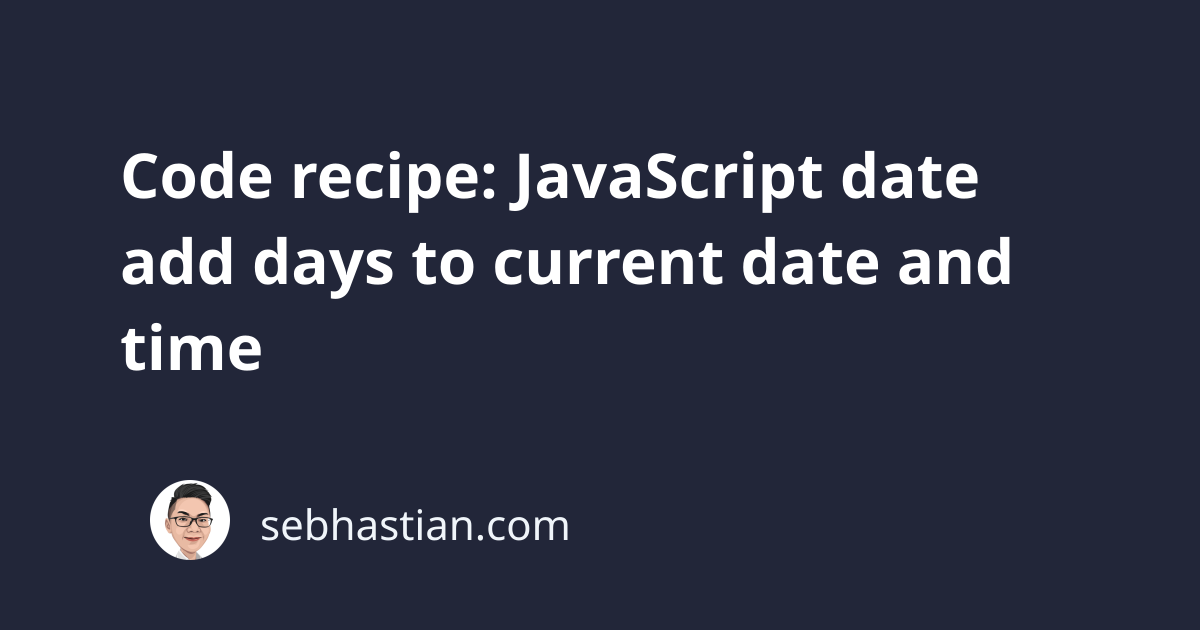
To increment a JavaScript date
object by one or more days, you can use the combination of setDate()
and getDate()
methods that are available for any JavaScript Date
object instance.
The setDate()
method allows you to change the date of the date
object by passing an integer representing the day of the month. On the other hand, the getDate()
method allows you to retrieve the day of the month.
Let’s see an example of their use.
First, create an appointment
constant that holds the value of 12 Feb 2021 00:00 AM
:
const appointment = new Date("February 12, 2021 00:00:00");
Then, you can retrieve the day using the getDate()
method. It will return 12
as the date is set manually when the appointment
object is created previously:
const appointment = new Date("February 12, 2021 00:00:00");
console.log(appointment.getDate()); // 12
To set the day of the month, you can use the setDate()
method:
const appointment = new Date("February 12, 2021 00:00:00");
appointment.setDate(22);
console.log(appointment.getDate()); // 22
Using what you’ve learned so far, you can call on the getDate()
method inside the setDate()
method and then increment it with as many days as you like.
Suppose that the appointment was postponed two days from the promised date. Here’s how you add two days to the appointment
variable:
const appointment = new Date("February 12, 2021 00:00:00");
appointment.setDate(appointment.getDate() + 2);
console.log(appointment.getDate()); // 14
And that’s how you add days to your current Date
object instance. The setDate()
method is also smart that if you pass a number outside the range of the current month, it will roll over into the next month:
const appointment = new Date("February 12, 2021 00:00:00");
appointment.setDate(appointment.getDate() + 30);
// The day exceeds Feb 28
// roll over into March
console.log(appointment); // Sun Mar 14 2021 00:00:00
Here’s a reusable function that you can use to add days to your Date
object. The function will create a copy of your Date
object by creating a new Date()
using Date.valueOf()
method. Then the setDate()
method will be called on that copy. This way, the original date won’t be modified by the function:
function addDays(originalDate, days){
cloneDate = new Date(originalDate.valueOf());
cloneDate.setDate(cloneDate.getDate() + days);
return cloneDate;
}
let appointment = new Date("February 12, 2021 00:00:00");
let newAppointment = addDays(appointment, 7);
console.log(appointment.getDate()); // 12
console.log(newAppointment.getDate()); // 19
You can modify the addDays()
function above to suit your requirements.