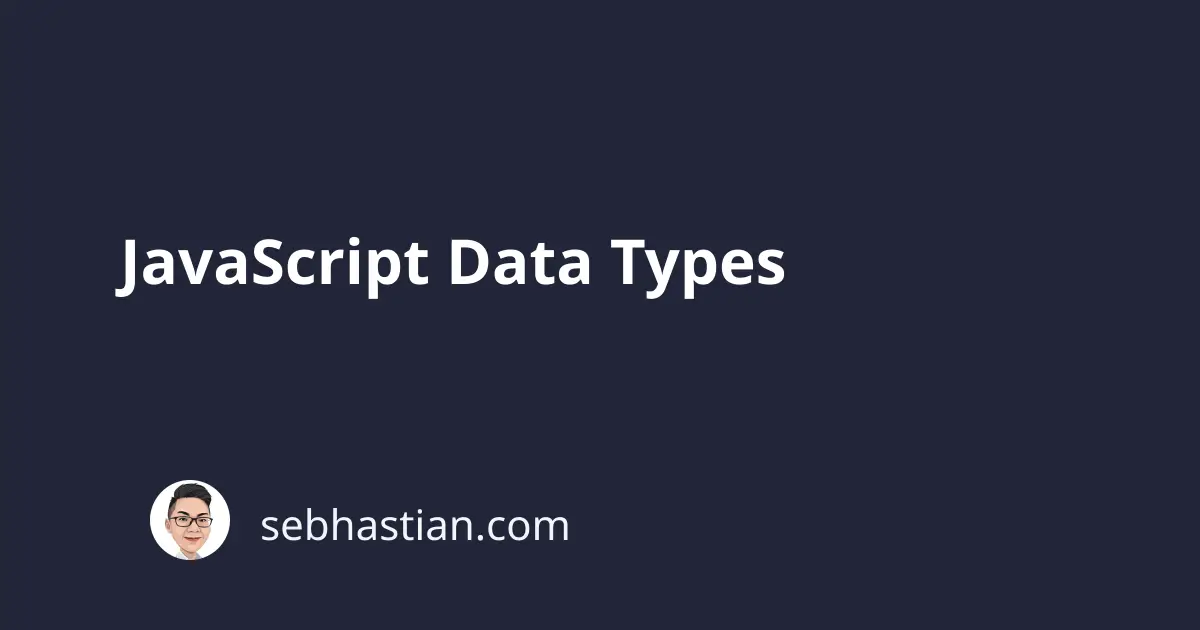
All programming languages have built-in data types, and JavaScript is no exception. The implementation of data types might differ from language to language, and although you might hear that JavaScript is an untyped language, it doesn’t mean that JavaScript has no type. We will discuss about JS data types here.
The ECMAScript standard defines seven different data types: Primitive Types
- Numbers
- Strings
- Booleans
- Null
- Undefined
- Symbols
.. and Object types
What is Primitive Data Type
They are essentially a data type that is not an Object and has no methods. The primitive values are immutable, meaning their value can’t be changed after declaration.
Number data type
In JavaScript, there is only one number data type, and that is double float format. This means you can have float numbers in an arithmetic equation without the need to convert data types.
console.log(5/2);
// returns 2.5
JavaScript will represent number as integer or float depending on your code
// Integer literal
10
100
1235845621
//Float literal
10.278
8.3e4
.564
String data type
JavaScript uses string data type to represent textual data. It is marked by enclosing in quotes or double quotes. Each element in the String occupies a position index.
The first element is at index 0, the next at index 1, and so on. The length of a String is the number of elements in it.
'This is a string'
"Another string here"
Sometimes you need to put quotation mark inside a string. JavaScript provides escape sequences in the form of backslash \
to handle this kind of operation
'I\'m a Software Developer and I work with \'JavaScript\''
// I'm a Software Developer and I work with 'JavaScript'
Other forms of backslash escape sequence includes: ' single quote " double quote \ backslash \n new line \r carriage return \t tab \b backspace You might use them when you deal with string data types
// Multi line string code with backslash
"This is \
a \
string"
// Multi line string interpretation
"This is \na \nstring"
String template literal
Template literals are string literals that allows developer to embed expressions in a string. They are marked by the enclosing back-tick mark ``` that surrounds them.
// template literals can create multi line string interpretation without \n
`This is
a
string`
Template literals can use variables or expressions as part of the string. This is done by using the ${}
to enclose the expression. Examples:
let name = "John";
`Your name is ${name} Snow, from House Stark.`
// normal string equivalent
'Your name is ' + name + ' Snow, from House Stark.'
// Using expressions
`The result of 10 + 19 is ${10+19}`
String also has an Object in JavaScript, which we will discuss later.
Boolean data type
In almost all programming language, Boolean data type consists of two values : true
and false
.
JavaScript is no different in this case. You can use many comparison operators like ==
or !=
to receive a Boolean value. You can learn more in Boolean operation post here.
8 == 8
7 != 10
9 >= 20 // ..is bigger or equal to..
"Nathan" != "Sebhastian" // will compare unicode values
JavaScript also has the notion of falsy values. They are special values that will always return false when evaluated in a Boolean context.
//They are alwasy falsy!
false
0
'' or "" //empty string
null
undefined
NaN //e.g. the result of 1/0
The rest of values are all truthy values
'0' //a string containing a single zero
'false' //a string containing the text "false"
[] //an empty array
{} //an empty object)
function(){} // an empty function
Null
This is a special value to denote the absence of value.
let name = null;
Undefined
This is a value returned by variables or functions that have not been defined yet.
let name;
console.log(name);
Object data type
They are data type for any values that are not primitive. The most distinguishing features that separate object from primitive type is that they have properties and methods and that they are written with Capital-first letter.
Some examples include Array and String. “Hold on, isn’t string a primitive data type?” That’s very attentive of you.
Yes, string is a data type and its primitive, but JavaScript has a set of built-in objects that can be used out of the box. Fortunately, one of those built-in objects is String
.
In runtime, JavaScript compiler automatically converts string primitives to String objects, so that it’s possible to use String object methods for primitive strings. Which means you can do this:
"This is a string".length
"Another string here".charAt(4)
For the full list of String object capabilites, you can visit Mozzila String object documentation