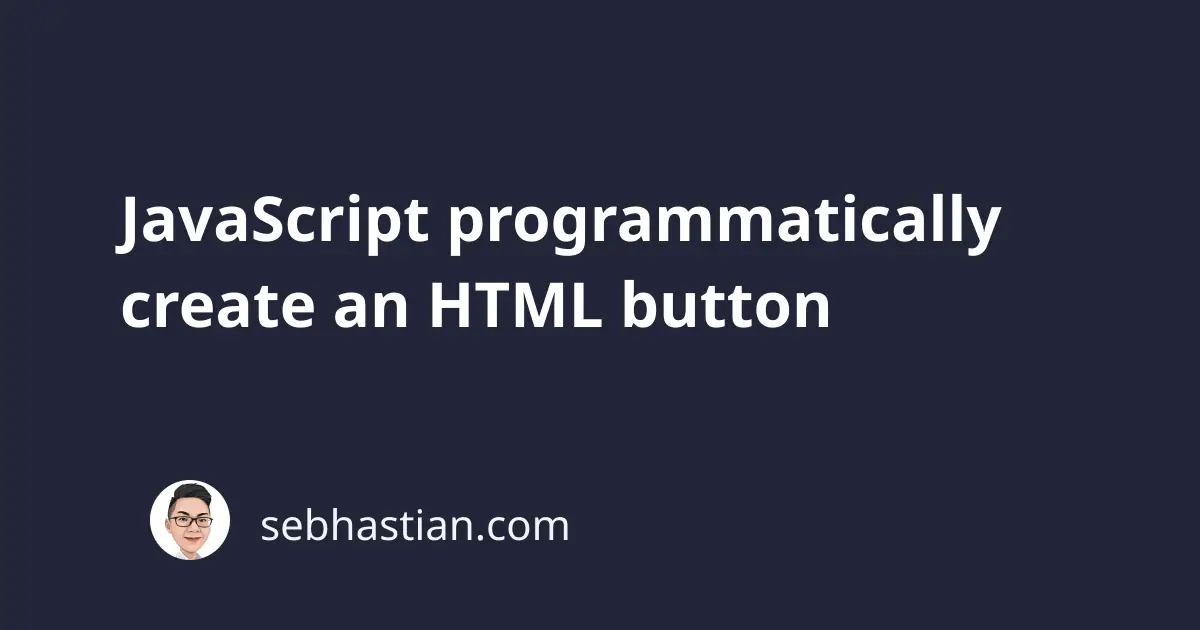
Sometimes you need to create an HTML button programmatically as a result of some code execution. You can easily create a button using JavaScript by calling on the document.createElement("button")
method.
For example, let’s create a <button>
that says "Click Me"
using JavaScript:
- First, you call the
document.createElement("button")
and assign the returned element to a variable namedbtn
. - Then, assign the
"Click Me"
string to thebtn.innerHTML
property - Finally, use
document.body.appendChild()
to append the button element to the<body>
tag
The code below shows how this can be done:
let btn = document.createElement("button");
btn.innerHTML = "Click Me";
document.body.appendChild(btn);
You can append the button element that you’ve created with JavaScript anywhere inside your HTML page by using the appendChild()
method.
You can also set the button’s name
, type
, or value
attributes as required by your project. Sometimes, you need to create a type='submit'
button for forms:
let btn = document.createElement("button");
btn.innerHTML = "Submit";
btn.type = "submit";
btn.name = "formBtn";
document.body.appendChild(btn);
The code above will create the following HTML <button>
tag:
<button type="submit" name="formBtn">
Submit
</button>
Finally, if you want to execute some code when the button is clicked, you can change the onclick
property to call a function as follows:
let btn = document.createElement("button");
btn.innerHTML = "Save";
btn.onclick = function () {
alert("Button is clicked");
};
document.body.appendChild(btn);
or you can also add an event listener as follows:
let btn = document.createElement("button");
btn.innerHTML = "Save";
btn.addEventListener("click", function () {
alert("Button is clicked");
});
document.body.appendChild(btn);
And that’s how you can create a button programmatically using JavaScript.