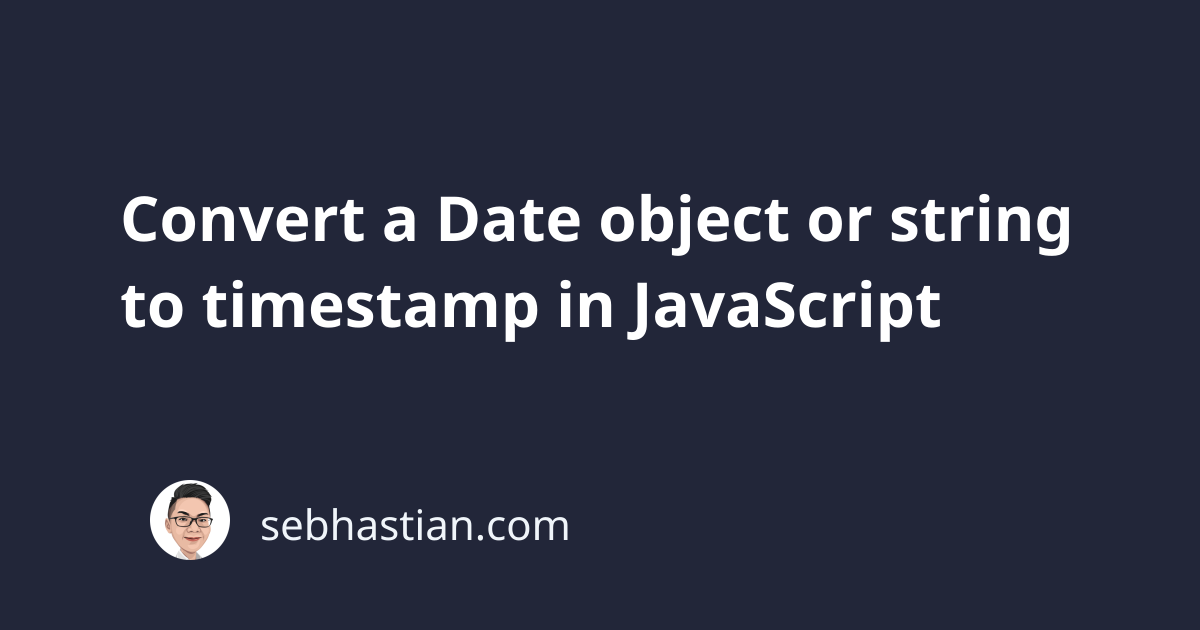
To convert a Date object or string to timestamp in JavaScript, you need to use the getTime()
method of the Date
object.
The get getTime()
method returns a Unix timestamp in milliseconds. You can divide the result by 1000 to get the epoch in seconds:
const myDate = new Date("July 20, 2009");
const timeMillis = myDate.getTime() / 1000;
console.log(timeMillis); // 1248022800
If you have a date string, then use the Date()
constructor to create a Date object from that string first before calling the getTime()
method.
But note that you need to pass the date in a specific format to make the Date()
constructor work.
If you have a date in dd/mm/yyyy
format for example, the Date()
constructor would return a NaN:
const myDate = new Date("25/01/2009").getTime();
console.log(myDate); // NaN
The Date()
constructor works most reliably on a string with yyyy-mm-dd
format, which is an ISO standard.
You need to split your string to an array, then pass each element into the Date()
constructor as follows:
let dateStr = "25/01/2009";
let dateArr = dateStr.split("/");
const myDate = new Date(`${dateArr[2]}-${dateArr[1]}-${dateArr[0]}`);
console.log(myDate.getTime() / 1000); // 1232841600
Whenever you need to use the Date()
constructor, always make sure that you’re passing a string in yyyy-mm-dd
format to avoid any issues.
If you use the forward slash /
as the date separator, change it to a dash -
also.
And that’s how you convert a date object or string into a timestamp in JavaScript. Until next time! 🙌