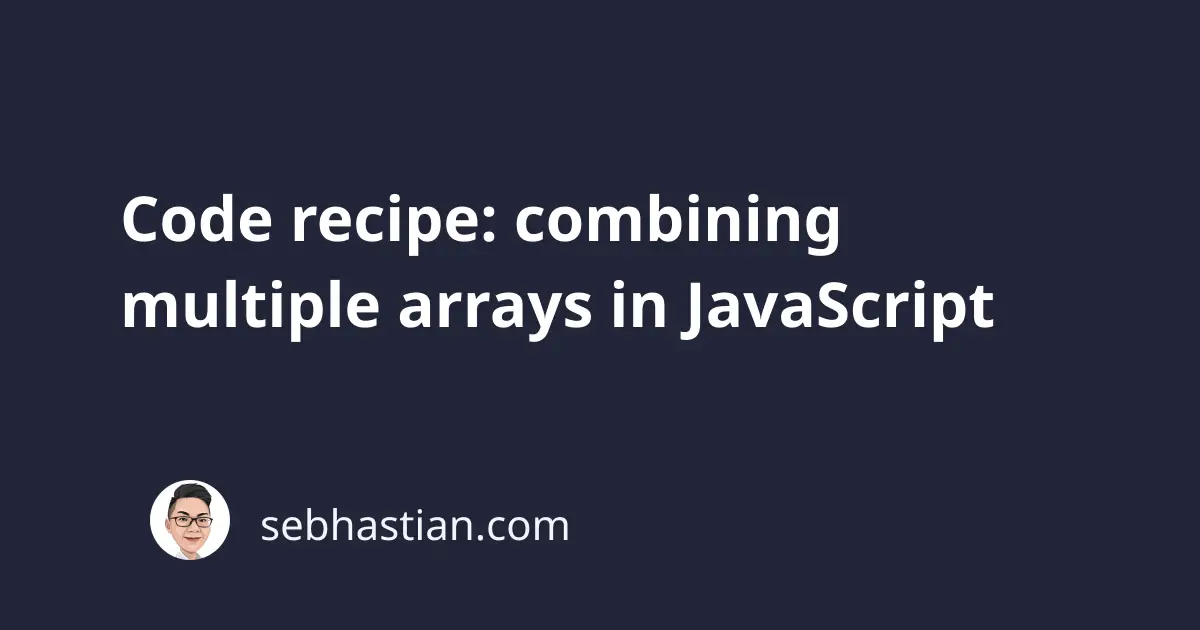
When you need to combine two or more JavaScript arrays, you can use either the Array.concat()
method or the spread operator.
This tutorial will show you how to combine arrays using both technique. Let’s start with the Array.concat()
method.
Combining arrays with concat() method
The concat()
method is a built-in method of JavaScript Array object. It returns a new array with elements combined from two or more arrays.
Here’s an example:
let felidae = ["lion", "tiger", "leopard"];
let canidae = ["coyote", "wolf", "fox"];
let animals = felidae.concat(canidae);
console.log(animals); // ["lion", "tiger", "leopard", "coyote", "wolf", "fox"]
console.log(felidae); // ["lion", "tiger", "leopard"]
console.log(canidae); // ["coyote", "wolf", "fox"]
The syntax of concat()
method above looks like the canidae
array is combined into felidae
array, but as you can see from the console logs, the felidae
array is actually unchanged.
To make the code more obvious, you can replace the call to concat()
using an empty array and place all arrays you want to merge as the parameters.
let felidae = ["lion", "tiger", "leopard"];
let canidae = ["coyote", "wolf", "fox"];
let animals = [].concat(felidae, canidae);
console.log(animals); // ["lion", "tiger", "leopard", "coyote", "wolf", "fox"]
The concat()
method allows you to combine as many array as you need.
let felidae = ["lion", "tiger"];
let canidae = ["fox", "wolf"];
let bovidae = ["cow", "goat"];
let animals = [].concat(felidae, canidae, bovidae);
console.log(animals); // ["lion", "tiger", "fox", "wolf", "cow", "goat"]
You’ve now learned how to combine arrays using the concat()
method. Let’s look at how you can combine arrays with the spread operator next.
Combining arrays with spread operator
The spread operator allows you to extract the elements of an array and pass it as elements of a new array.
Here’s an example of combining two arrays with spread operator:
let felidae = ["lion", "tiger"];
let canidae = ["fox", "wolf"];
let animals = [...felidae, ...canidae];
console.log(animals); // ["lion", "tiger", "fox", "wolf"]
Both concat()
method and spread operator can be used to combine multiple arrays just fine.
Reusable function to combine arrays
Finally, here’s a reusable function that you can use in your project to combine two or more arrays. The function makes use Array.reduce()
method and the spread operator:
function mergeArr(...arr) {
return arr.reduce((acc, val) => {
return [...acc, ...val];
}, []);
}
const felidae = ["lion", "tiger"];
const canidae = ["fox", "wolf"];
const bovidae = ["cow", "goat"];
const animals = mergeArr(felidae, canidae, bovidae);
console.log(animals); // ["lion", "tiger", "fox", "wolf", "cow", "goat"]
Feel free to use the function mergeArr()
in your projects.