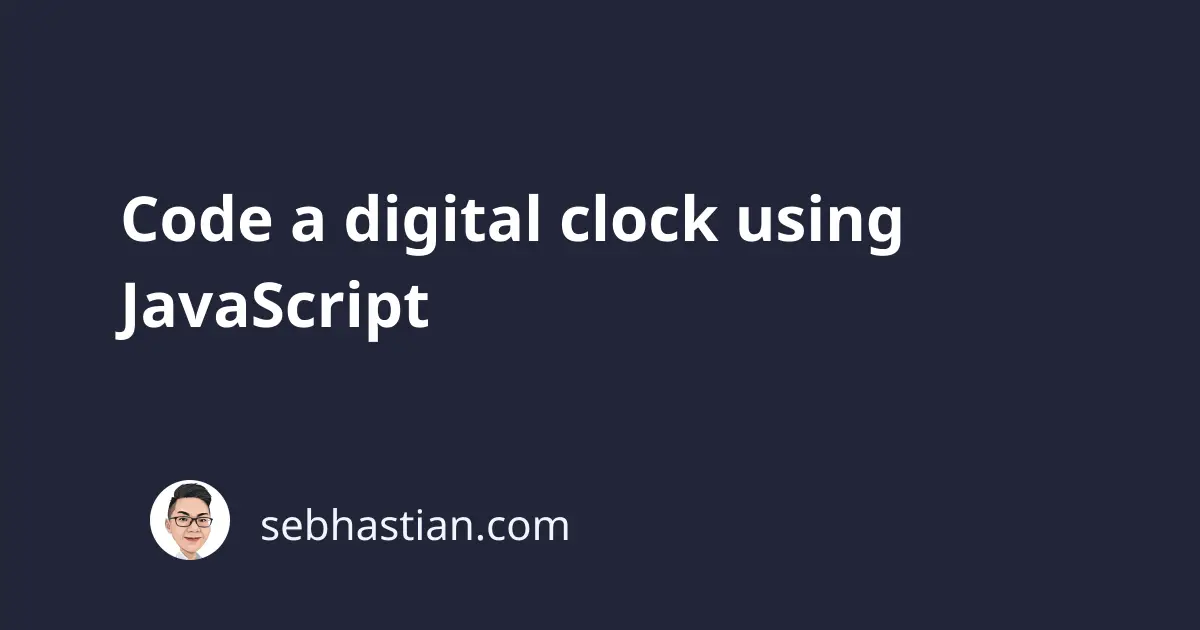
This tutorial will help you learn how to code a digital clock using JavaScript. Here’s a live demo of the result:
See the Pen JavasScript clock by Nathan Sebhastian (@nathansebhastian) on CodePen.
First, you need to have an HTML element where you can display the current clock value. You can create either a <div>
or a <span>
element.
I will use a <div>
with the id
value of clockDiv
:
<div id="clockDiv"></div>
Next, you need to write the script that will get the current hour, minute, and second values from a JavaScript Date
object.
You can get the current time value using the new Date()
method:
function getClockTime() {
let date = new Date();
}
Next, get the hour, minute, and second values using the getHours()
, getMinutes()
, getSeconds()
methods:
function getClockTime() {
let date = new Date();
let hr = date.getHours();
let min = date.getMinutes();
let sec = date.getSeconds();
}
The getHours()
method will return the current hour in number
format between 0 - 23
while the getMinutes()
and getSeconds()
will return the minute and second between 0 - 59
A digital clock requires the values to be in 2-digit format, so when the value is single-digit, you need to add a 0
before the value.
The obvious way would be as follows:
function getClockTime() {
let date = new Date();
let hr = date.getHours();
let min = date.getMinutes();
let sec = date.getSeconds();
hr = hr < 10 ? "0" + hr : hr;
min = min < 10 ? "0" + min : min;
sec = sec < 10 ? "0" + sec : sec;
}
But actually, you can make a more smart code by always adding 0
before the values and use the slice()
method to grab the last two characters.
Take a look at the code below:
function getClockTime() {
let date = new Date();
let hr = date.getHours();
let min = date.getMinutes();
let sec = date.getSeconds();
hr = hr < 10 ? "0" + hr : hr;
min = min < 10 ? "0" + min : min;
sec = sec < 10 ? "0" + sec : sec;
hr = ("0" + hr).slice(-2);
min = ("0" + min).slice(-2);
sec = ("0" + sec).slice(-2);
}
With that, you have the code to get the hour, minute, and second values. For the last step, you need to assign the values to the innerHTML
property as follows:
let clockEl = document.getElementById("clockDiv");
function getClockTime() {
let date = new Date();
let hr = date.getHours();
let min = date.getMinutes();
let sec = date.getSeconds();
hr = ("0" + hr).slice(-2);
min = ("0" + min).slice(-2);
sec = ("0" + sec).slice(-2);
clockEl.innerHTML = `${hr}:${min}:${sec}`;
}
With that, the time values are now displayed inside the HTML <div>
tag. The last thing you need to do is to call the getClockTime()
function every second. You can do this using the setInterval()
method as shown below:
setInterval(getClockTime, 1000);
The full HTML code will be as follows:
<body>
<div id="clockDiv"></div>
<script>
let clockEl = document.getElementById("clockDiv");
function getClockTime() {
let date = new Date();
let hr = date.getHours();
let min = date.getMinutes();
let sec = date.getSeconds();
hr = ("0" + hr).slice(-2);
min = ("0" + min).slice(-2);
sec = ("0" + sec).slice(-2);
clockEl.innerHTML = `${hr}:${min}:${sec}`;
}
setInterval(getClockTime, 1000);
</script>
</body>
Now you’ve learned how to create a clock using JavaScript. Let’s style the clock a bit so it looks better.
Add the following <style>
to your <head>
tag:
<style>
body {
background-color: black;
}
#clockDiv {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
color: white;
font-size: 52px;
font-family: monospace;
}
</style>
Now you have a big clock at the center with a dark background.
Next, let’s figure out how to add AM/PM
to make a 12-hour format clock.
JavaScript clock with AM/PM format
To make a 12-hour format clock with JavaScript, you need to add a period
variable which defaults to "AM"
.
When hr
value is greater than 12
, then you need to decrement hr
value by 12
and change the period
value from "AM"
to "PM"
.
Take a look at the following code and notice how the two if
blocks change the clock format:
let clockEl = document.getElementById("clockDiv");
function getClock() {
let date = new Date();
let hr = date.getHours();
let min = date.getMinutes();
let sec = date.getSeconds();
let period = "AM";
if (hr == 0) {
hr = 12;
}
if (hr > 12) {
hr = hr - 12;
period = "PM";
}
hr = ("0" + hr).slice(-2);
min = ("0" + min).slice(-2);
sec = ("0" + sec).slice(-2);
clockEl.innerHTML = `${hr}:${min}:${sec} ${period}`;
}
setInterval(getClock, 1000);
You can try the code above in the same <div>
element.
Now you’ve learned how to create a digital clock using JavaScript in both 24-hour and 12-hour format. Great job 😉