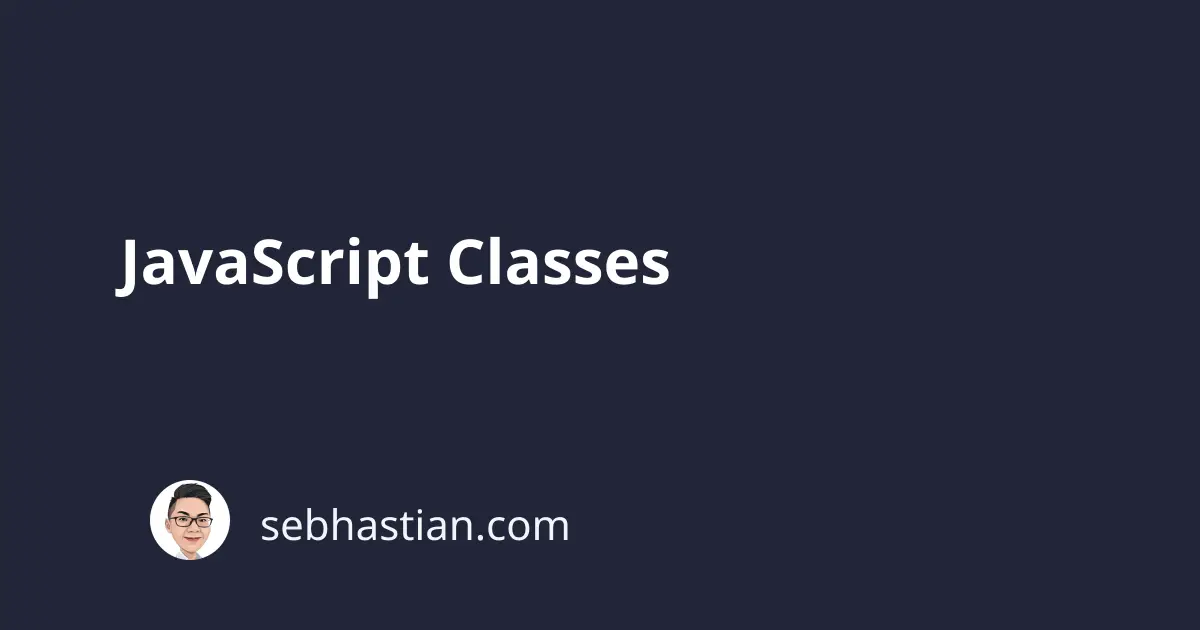
Before the addition of class
keyword into ECMAScript specification, JavaScript had a little known way to implement inheritance through prototypal inheritance.
It was a good implementation of inheritance, but since it was different from other programming languages.
In order to make it easier for developers, the ECMAScript committee introduced the concept of class in ES2015, which totally resembles classes from popular programming languages such as Python or Ruby.
It’s important to note that the class syntax does not introduce a new object-oriented inheritance model to JavaScript, since it’s merely a syntactic sugar on top of the existing prototype based inheritance.
Defining a Class
One way to define a class is through the use of class declarations, which looks like this
class Car {
constructor(model, name) {
this.model = model;
this.name = name;
}
start() {
console.log(name + " engine started");
}
}
A class declared with a name which acts as its identifier. We can use the name to create new objects using the keyword new.
The arguments we put into the new object will be passed on to the constructor method of the class, which will run immediately on initialization.
A class also can have as many methods as we define in it. In the example below, we called the method start()
after we initialize a new object.
class Car {
constructor(model, name) {
this.model = model;
this.name = name;
}
start() {
console.log(`${this.model} ${this.name} engine started.`);
}
}
let honda = new Car("Honda", "Brio");
honda.start();
Inheritance
A class can be extended by another class, and the new class will inherit all of its parent class methods. The object that initialize the child class will then be able to use methods from both classes.
If two or more methods with the same name are declared in the classes, the method closest to the object will be executed while the rest will be ignored.
class ElectricCar extends Car {
start() {
return super.start() + " Battery at 95%.";
}
}
let tesla = new ElectricCar("Tesla", "Model 3");
tesla.start();
You can reference the parent class method by using the super
keyword.
Getters and Setters
The get and set is a set of keywords to define methods that will be used for accessing variable and modifying its value.
class Car {
constructor(name) {
this._name = name;
}
set name(value) {
this._name = value;
}
get name() {
return this._name;
}
}
let honda = new Car("Honda");
console.log(honda.name);
honda.name = "NotHonda";
console.log(honda.name);
Note the use of _
prefix in the variable name to do the double duty of marking the variable as private and avoiding infinite call into the setter method.
You also can omit one of the methods and the code will still run. Only remember that without a setter, the property cannot be set, and any attempt to do so will be ignored. The same applies to getter methods.