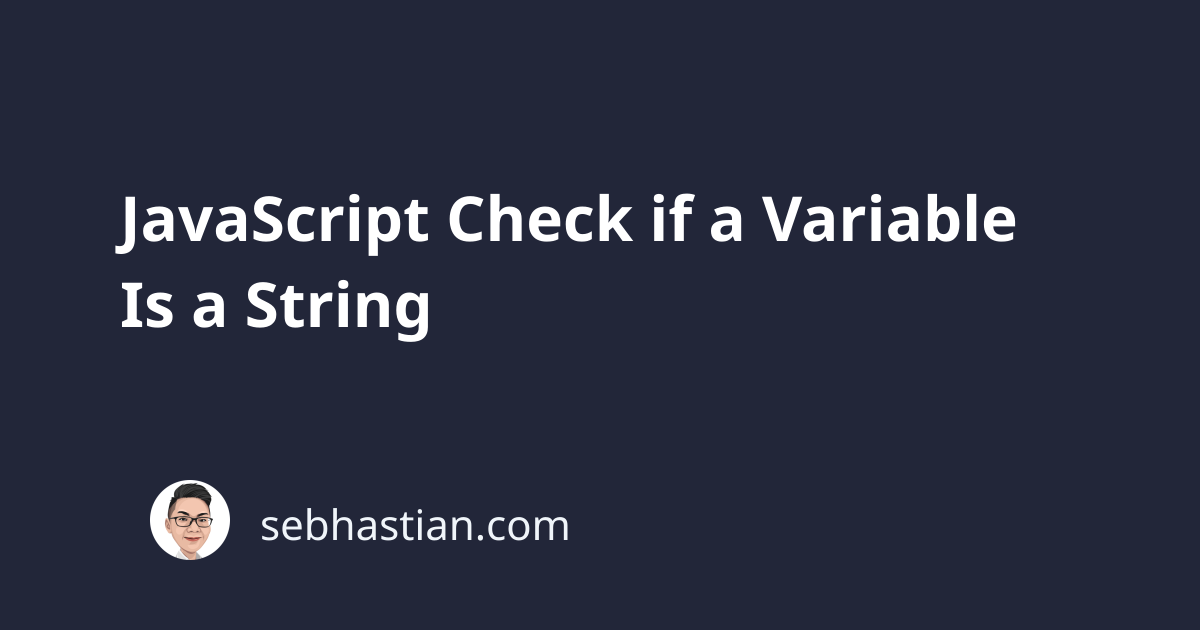
There are two simple ways you can check if a variable is a string in JavaScript:
- Using the
typeof
operator - Using the
instanceof
operator
Both are used in different ways, so let’s see a quick example of how to use these.
Using the typeof operator
The typeof
operator is used to check the type of a variable. You can add an equality comparison operator ===
to check if the variable is of ‘string’ type:
let myVariable = 'Hello';
console.log(typeof myVariable === 'string'); // true
The comparison returns true
when the variable is indeed a string. You can use an if-else
statement to let JavaScript know what to do when the variable is a string or not:
let myVariable = 'Hello';
if (typeof myVariable === 'string') {
console.log('The variable is a string');
} else {
console.log('The variable is NOT a string');
}
But keep in mind that this comparison will return false
when you have a String
object and not a string primitive. Consider the following example:
let myVariable = new String('Hello');
if (typeof myVariable === 'string') {
console.log('The variable is a string');
} else {
console.log('The variable is NOT a string'); // ✅
}
This code will trigger the else
statement because the String()
constructor creates an object type value.
To check for this kind of string, you need to use the instanceof
operator.
Using the instanceof
operator
The instanceof
operator is used to test whether a specific constructor appears or is used to create the object you test with this operator.
Because we’re testing a String
object, you can use the operator like this:
let myVariable = new String('Hello');
if (myVariable instanceof String) {
console.log('The variable is a string'); // ✅
} else {
console.log('The variable is NOT a string');
}
Because the String()
constructor is used to create the object, the operator returns true
.
But if you test a primitive string value, then the instanceof
operator would return false
. You need to use the solution appropriate for your case.
And that’s how you check if a variable is a string in JavaScript. Hope that helps and happy coding! 👍