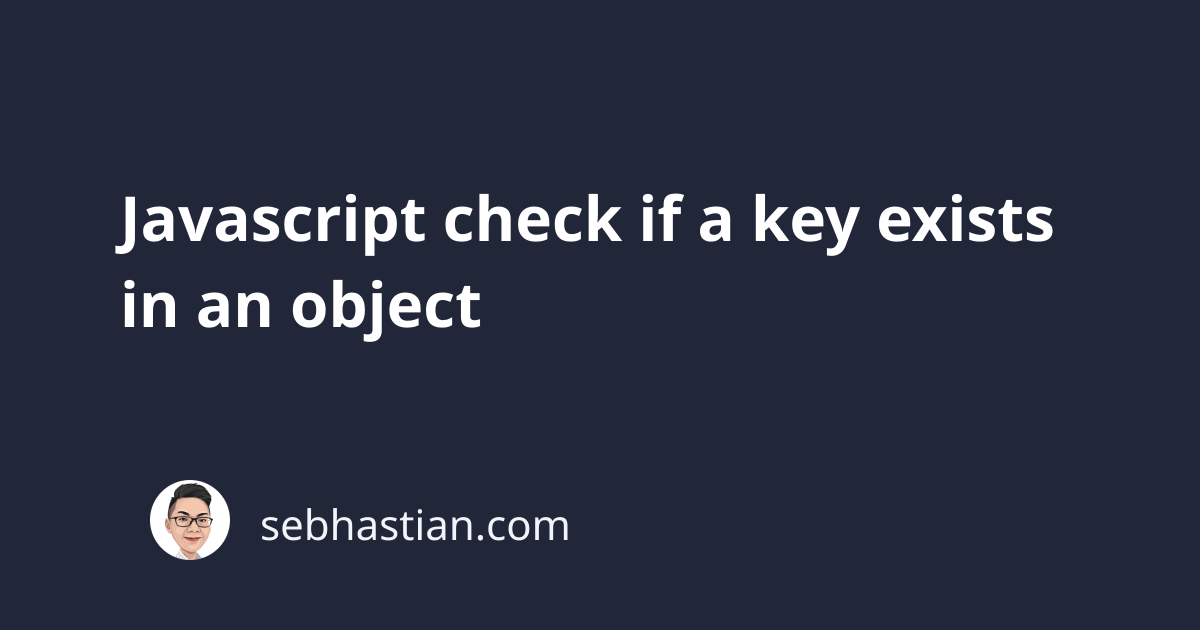
A JavaScript object is a special data structure that contains data as a key-value pair.
The object key is also known as the object property, while the value is called property value.
There are two ways you can check if a key exists in an object:
- Use the
in
operator - Use the
hasOwnProperty()
method
Both solutions work in a similar way. They will check for the existence of a key or property in your object.
Here’s the code snippet to perform the check in case you’re in a hurry:
"key" in object;
object.hasOwnProperty("key");
Let’s see an example of how to use these solutions next.
1. Check if a key exists using the in
operator
The in
operator checks if a specified key exists in an object. You need to specify the key as the left operand, and the object as the right operand. The key should be specified as a string.
The operator returns true
when the key exists, otherwise it returns false
.
The following example checks if an object named user
has a property named age
and address
:
let user = {
name: "John",
age: 28,
};
console.log("age" in user); // true
console.log("address" in user); // false
Here, you can see that the age
key exists, so the operator returns true
.
2. Check if a key exists using the hasOwnProperty()
method
The hasOwnProperty()
method is a built-in method of the Object data type that allows you to check if a specific property exists in an object.
Below you can see how to use the method to check if a property named ‘age’ and ‘address’ exists in the user
object:
let user = {
name: "John",
age: 28,
};
console.log(user.hasOwnProperty("age")); // true
console.log(user.hasOwnProperty("address")); // false
When using the hasOwnProperty()
method, you need to specify the property name or key you want to check as a string type argument to the method.
The method returns true
if the key exists, or false
if not.
The hasOwnProperty()
method ignores built-in properties provided by the Object data type, while the in operator doesn’t.
You can check for the hasOwnProperty
method itself to verify this difference:
let user = {
name: "John",
age: 28,
};
console.log("hasOwnProperty" in user); // true
console.log(user.hasOwnProperty("hasOwnProperty")); // false
console.log("toString" in user); // true
console.log(user.hasOwnProperty("toString")); // false
Here, you can see that built-in methods like hasOwnProperty
and toString
are ignored when using the hasOwnProperty()
method, while the in
operator takes them.
As a developer, you’re most likely going to check for a key that you added to the object, so it’s not going to be a problem whichever solution you use
Conclusion
In this article, you’ve learned how to check if an object has a key using the two standard solutions: the hasOwnProperty()
method and the in
operator.
I hope this tutorial is useful. Thanks for reading and Happy coding!