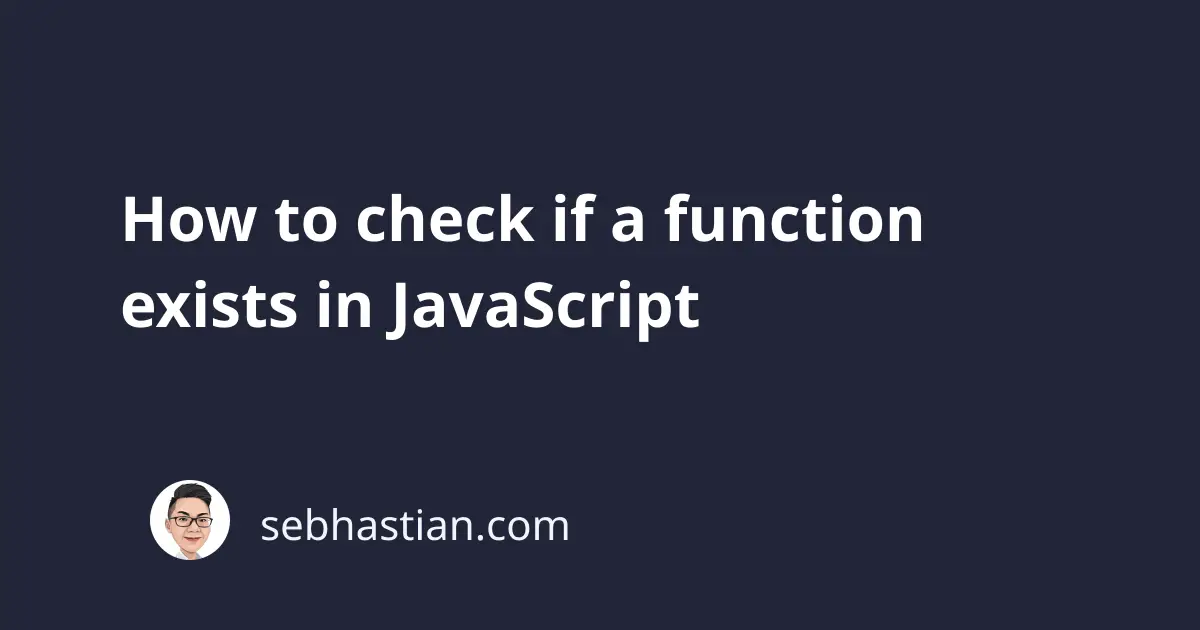
An error caused by calling a non-existent function will cause JavaScript to throw an undefined
error and stop running your code. To prevent the error, you can first check if a function exists in your current JavaScript environment by using a combination of an if
statement and the typeof
operator on the function you want to call.
Only when the typeof
returns "function"
will you call that function to execute it. Let’s look at the following example:
function printMessage(){
console.log("Hello World!");
}
if(typeof printMessage === "function"){
printMessage();
}
When the type of printMessage
is anything other than "function"
, you don’t call the function.
Handling non-existent function with try..catch block
Alternatively, you can also use a try..catch
block to handle the error when a non-existent function is called:
try {
printMessage();
} catch (err) {
console.log(err); // Error: "printMessage is not defined"
}
// code below won't be executed without try..catch block
console.log("abc...");
console.log("...The script execution continues...");
console.log("...even when there's an error");
Without the try..catch
block, the console logs above won’t be executed by JavaScript. You can use the techniques above to check if a JavaScript function exists before calling it.