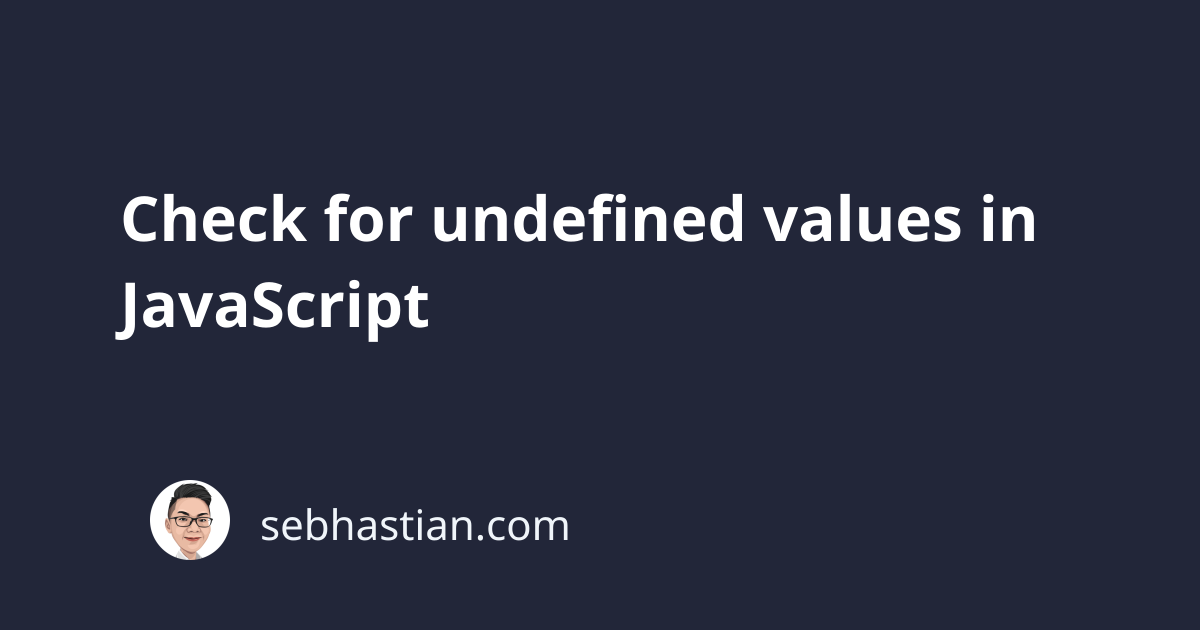
To check for undefined
variables or object properties in JavaScript, you need to use the typeof
operator.
The typeof
operator is used to find the type of the value you add as its operand. The operator returns a string
representing the type of the value.
You can use the operator to check for undefined
values as shown below:
console.log(typeof 42);
// output: "number"
console.log(typeof undeclaredVariable);
// output: "undefined"
let myObj = {
color: "red"
};
console.log(typeof myObj.color);
// output: "string"
console.log(typeof myObj.id);
// output: "undefined"
As you can see, you can check for undefined values by using the typeof
operator.
By combining the operator with an if
statement, you can create a conditional code that will be executed only when a value is undefined
:
let myObj = {
color: "red"
};
if (typeof myObj.id === "undefined") {
// id is undefined
console.log("The id property is undefined");
} else {
// id is defined
console.log("The id property is available");
}
In some cases where you have the variable or object property explicitly declared as undefined
, you can also use a strict equal operator to undefined
value directly.
Consider the following code example:
let undefinedVar = undefined;
if (undefinedVar === undefined) {
console.log("Variable has undefined value");
}
In the above example, the undefinedVar
variable will hit the if
statement because the value is equal to undefined
. You don’t need the typeof
operator when the variable is initialized.
But if you never declare the variable at all, then using the ===
operator without the typeof
operator will produce an error:
console.log(newVar === undefined);
// ReferenceError: newVar is not defined
This is why it’s better to use the typeof
operator to really check if a value is undefined
.
The typeof
operator won’t throw an error when you haven’t declared the variable during the checking, but a direct comparison such as y === undefined
will break your JavaScript application.
When using the typeof
operator, compare the variable or object property with the string "undefined"
instead of the undefined
value:
typeof y === undefined; // false
typeof y === "undefined"; // true
Now you’ve learned how to check for undefined
values using the typeof
operator. Nice work! 😉