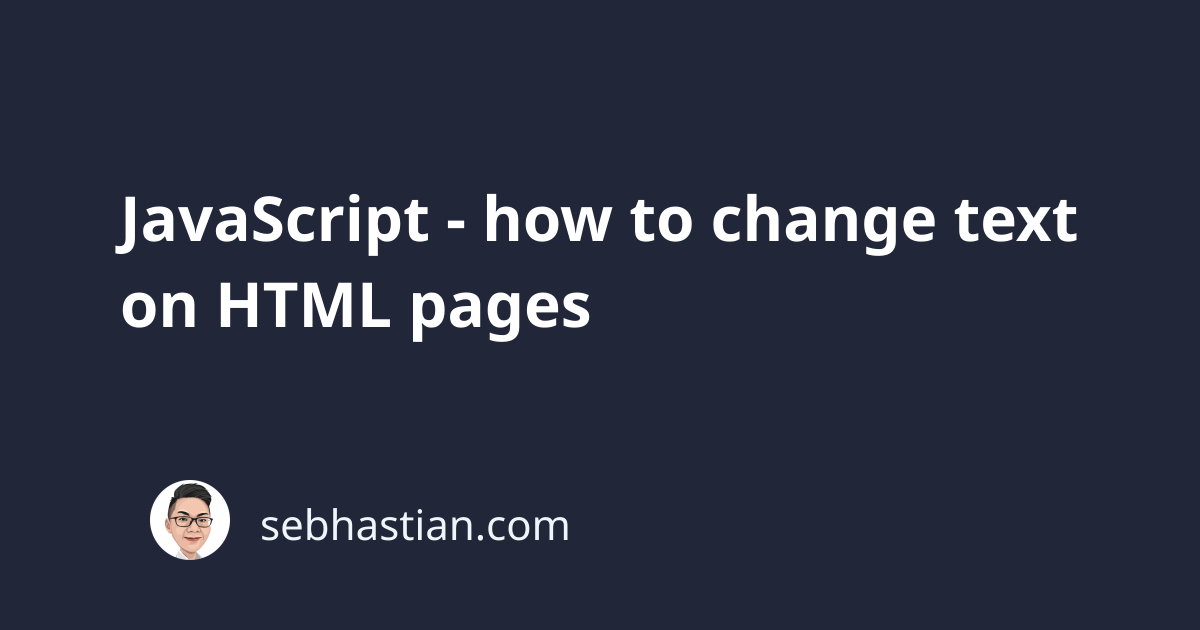
The easiest way to change the text content of HTML pages is to manipulate the textContent
property of the specific element using JavaScript.
First, you need to select the element you want to change the text. It may be a <span>
, a <div>
, a <p>
or any other tag. All HTML elements have the textContent
property from which you can set the text for that element.
For example, suppose you have the following HTML page:
<body>
<h1 id="header">Hello, World!</h1>
<p id="content">It's a very good weather we are having today.</p>
</body>
Here’s how to change the text inside the <h1>
element into “Greetings from Mark”:
First, you need to select the element <h1>
using JavaScript’s element selector. The browser provides you with 4 methods to select an HTML element:
getElementById()
- fetch a single element with matchingid
attributegetElementsByClassName()
- fetch the elements with matchingclass
attributegetElementsByName()
- fetch the elements with matchingname
attributegetElementsByTagName()
- fetch the elements with matching tag name like"li"
for<li>
,"body"
for<body>
The most recommended approach is to use getElementById()
method because it’s the most specific of these methods.
Out of the four methods, only getElementById()
will retrieve a single element as an object. The other methods will fetch the elements as an array even when the page has only one matching element.
Since the <h1>
tag above already has an id
attribute, you can use that to get the element as follows:
const header = document.getElementById("header");
If your element doesn’t have an id
attribute, then you can add it first. Please make sure that no two elements or more have the same id
attribute value. JavaScript will return only the first element it found if you do.
Next, you just need to replace the textContent
property as in the code below:
header.textContent = "Greetings from Mark";
And just like that, you’ll have the text inside the <h1>
tag changed.
Keep in mind that the textContent
property is used only to change the text inside the element. If you add an HTML tag inside the text, it will be displayed on the page literally.
For example, suppose you want to add an italic styled text with <i>
tag. You may try to add it like this:
header.textContent = "<i>Greetings from Mark</i>";
What would happen is the text "<i>Greetings from Mark</i>"
will be displayed on the HTML page.
To properly add HTML tags to your new text, you need to use the innerHTML
property instead of the textContent
property. Here’s an example:
header.innerHTML = "<i>Greetings from Mark</i>";
Now the <h1>
tag content will be styled properly.
The innerHTML
property allows you to set the HTML content of the element, not just plain text as in textContent
property. Feel free to use the method that meets your need 😉
You can use the same methods to change other HTML elements like a <div>
, a <span>
, or a <p>
tag.