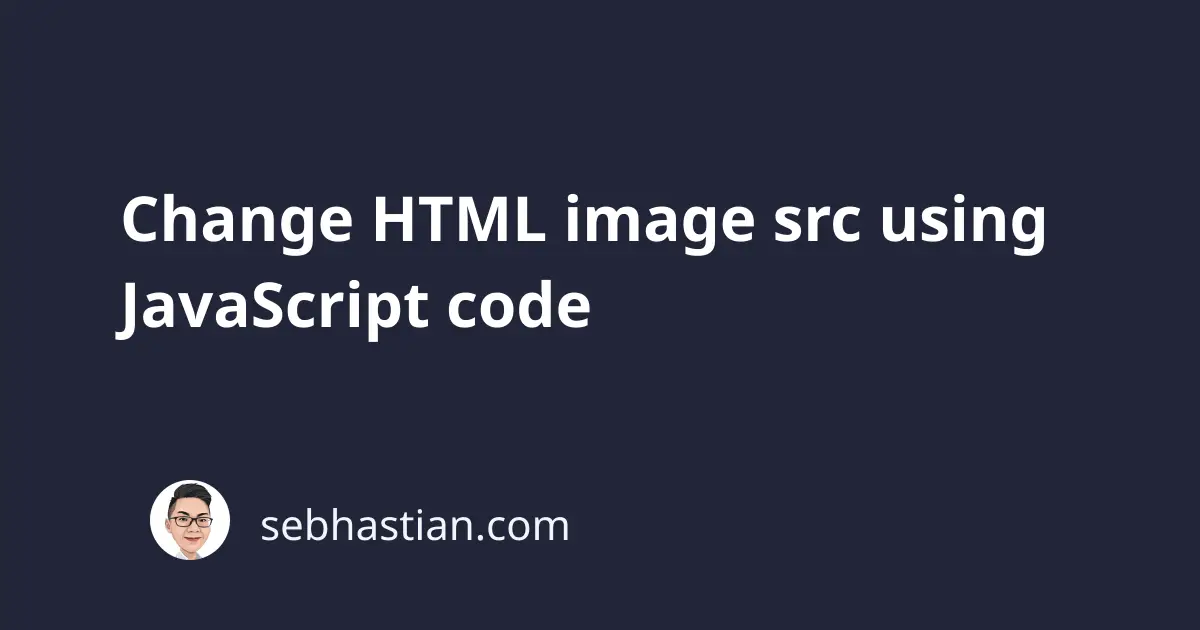
You can change an HTML image src
attribute programatically by using JavaScript. First, you need to grab the HTML element by using JavaScript element selector methods like getElementById()
or querySelector()
and assign the element to a variable.
After you have the element, you can assign a new string
value to its src
property as follows:
<body>
<img id="banner" src="image.jpg" />
<script>
const img = document.getElementById("banner");
img.src = "newImage.png";
</script>
</body>
When you have the image in another folder, than you need to add the image path to the src
attribute as well. The code below shows how to change the src
attribute to an image inside the assets/
folder:
img.src = "./assets/newImage.png";
Finally, if you need to run some JavaScript code after a new image has been loaded to the element, you can add an onload
event loader to your img
element before changing the src
attribute as follows:
const img = document.getElementById("banner");
img.onload = function () {
alert("New image has been loaded");
};
img.src = "newImage.png";
Using the above code, an alert will be triggered when the new image from the src
attribute has been loaded to the HTML page.