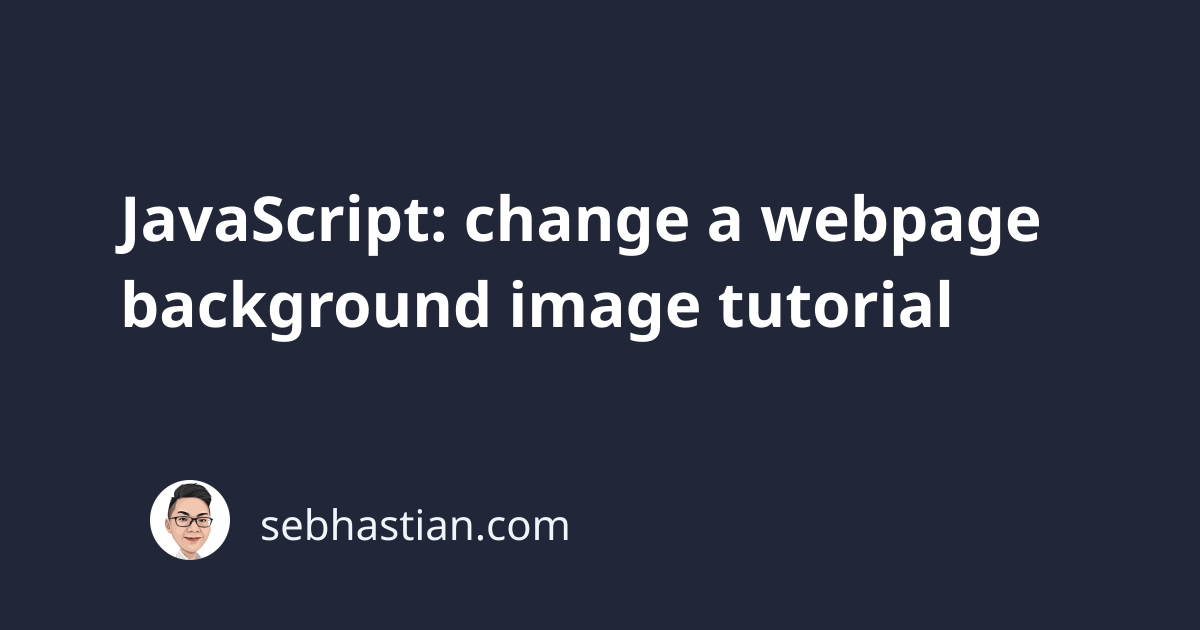
When you want to change a webpage background image using JavaScript, you can do so by setting the background image of the document object model (DOM) property.
The property you need to manipulate for changing the background image of the whole page is document.body.style.backgroundImage
:
document.body.style.backgroundImage = "url('image.png')";
You need to put the relative path to the image from your web page’s folder. If the image is located in the same folder, then you can reference it directly inside the url()
function as in the code above.
When the image is one folder down, add the correct relative path as follows:
document.body.style.backgroundImage = "url('./assets/image.png')";
Or go up one folder with the following relative path:
document.body.style.backgroundImage = "url('../image.png')";
If you have the image URL available publicly on the internet, you can pass the full url into the property as in the following example:
document.body.style.backgroundImage =
"url('https://sebhastian.com/img/default.png')";
The code above will change the background image into an image that I have created for my website. You can test the code out by changing the image of Google’s homepage. First, open your browser’s developer console. Then in the Console tab next to Elements tab, write the following code:
document.body.style.backgroundImage =
"url('https://sebhastian.com/img/default.png')";
The background image of Google’s homepage will change to the specified image, just like in the screenshot below:
By default, the image you selected as a background will be repeated to cover the whole page. You may want to style the background image further by setting the background-repeat
style to "no-repeat"
and the background-size
style to "cover"
.
To add the extra styles using JavaScript, you need to set the style
property, which contains the same styling property but with a camelCase
instead of kebab-case
. For example, background-repeat
becomes backgroundRepeat
:
document.body.style.backgroundRepeat = "no-repeat";
document.body.style.backgroundSize = "cover";
Inside your web application, you may want to trigger the background image change when the visitors do something. For example, you can change the background image when a <button>
is clicked:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript change background image</title>
<script>
// The function below will change the background image
function changeBackgroundImage() {
document.body.style.backgroundImage = "url('image.png')";
document.body.style.backgroundRepeat = "no-repeat";
document.body.style.backgroundSize = "cover";
}
</script>
</head>
<body>
<h1>Click the button to change the background image:</h1>
<button onclick="changeBackgroundImage();">Change</button>
</body>
</html>
Or you can also immediately change the background image after the page is loaded by listening to the DOMContentLoaded
event as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript change background image</title>
<script>
// The function below will change the background image
function changeBackgroundImage() {
document.body.style.backgroundImage = "url('image.png')";
}
// call the function when the whole DOM content is loaded
document.addEventListener("DOMContentLoaded", changeBackgroundImage());
</script>
</head>
<body>
<h1>Change the background image on load</h1>
</body>
</html>
The DOMContentLoaded
event is triggered when your whole HTML page has been loaded, even though some external JavaScript, CSS, and image resources are not yet fully loaded.
If you want to wait until the page and the resources are fully loaded, you can listen for the load
event instead:
document.addEventListener("load", changeBackgroundImage());
Changing the background image of a specific element
The document.body
code means you are selecting the <body>
tag of your HTML page. You can also change only a part of your page by selecting only that specific element.
The browser provides you with several ways to select a specific element:
getElementById()
- get a single element with matchingid
attributegetElementsByClassName()
- get elements with matchingclass
attributegetElementsByName()
- get elements with matchingname
attributegetElementsByTagName()
- get elements with matching tag name like"li"
for<li>
,"body"
for<body>
The most recommended approach is to use getElementById()
because it’s the most specific of these methods. Out of the four methods, only getElementById()
will retrieve a single element.
The following example shows how you can change the background of <div>
element with id="user"
attribute:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript change background image</title>
<script>
// change the background image of div user
function changeBackgroundUser() {
document.getElementById("user").style.backgroundImage =
"url('image.png')";
}
</script>
</head>
<body>
<div id="user">
<h1>Change specific DIV background image</h1>
</div>
<div id="guest">
<h2>This element's parent DIV background won't be changed</h2>
</div>
<div id="stranger">
<h2>Neither this one</h2>
</div>
<button onclick="changeBackgroundUser();">Change BG image</button>
</body>
</html>
Finally, you can put the bgImage
as a parameter to the changeBackgroundImage()
function so that you can have buttons that change the background to different images:
<script>
// change the background image using the passed parameter
function changeBackgroundImage(bgImage) {
document.body.style.backgroundImage = "url(" + bgImage + ")";
}
</script>
<button onclick="changeBackgroundImage('image.png');">Use image.png</button>
<button onclick="changeBackgroundImage('pic.jpg');">Use pic.jpg</button>
<button onclick="changeBackgroundImage('user.png');">Use user.png</button>
And that’s how you change the background image of a webpage using JavaScript 😉