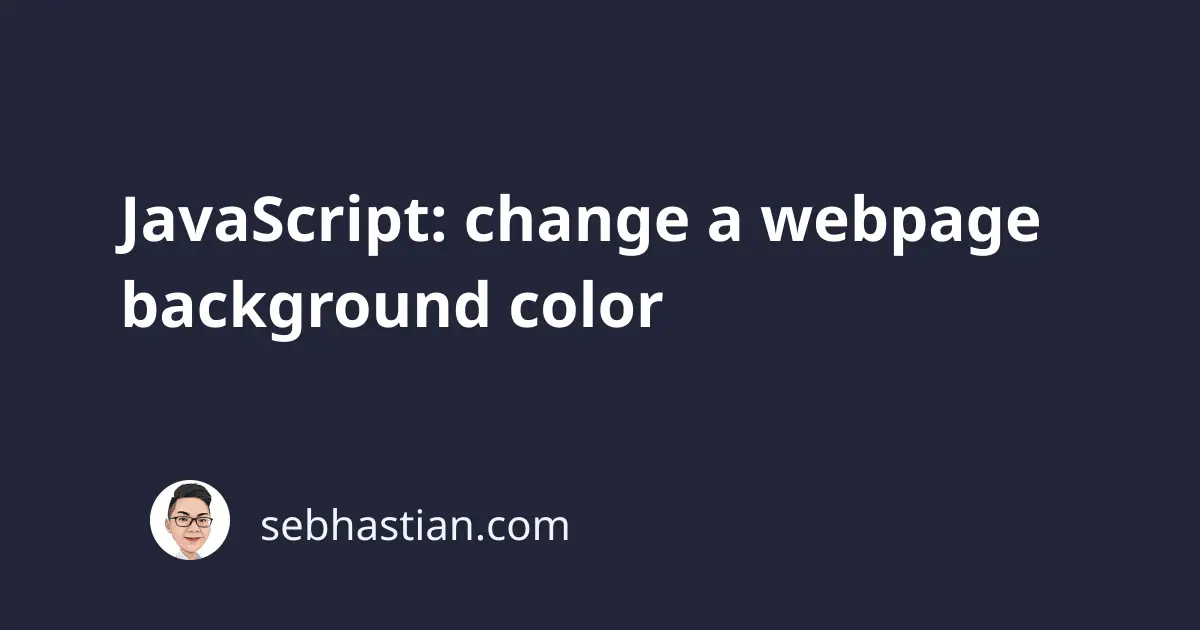
When you want to change a webpage background color using JavaScript, you need to manipulate the document object model (DOM) property by calling it inside your <script>
tag.
The property you need to manipulate for changing the background color of the whole page is document.body.style.background
:
document.body.style.background = "red";
You can test the code out by changing the color of Google’s homepage. First, open your browser’s developer console. Then in the Console tab next to Elements, write the following code:
document.body.style.background = "orange";
The color of Google’s homepage will change to Orange, just like in the screenshot below:
Back when I first learned how to code, I used to do this to impress my non-coder friends. But inside your project, you probably want to trigger the color change when the visitors do something. For example, you can change the background color when a <button>
is clicked:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript change background color</title>
<script>
// The function below will change the background color
function changeBackgroundRed() {
document.body.style.background = "red";
}
</script>
</head>
<body>
<h1>Click the button to change the background:</h1>
<button onclick="changeBackgroundRed();">Red</button>
</body>
</html>
Or you can also immediately change the background after the page is loaded by listening to the DOMContentLoaded
event as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript change background color</title>
<script>
// The function below will change the background color
function changeBackgroundRed() {
document.body.style.background = "red";
}
// call the function when the whole DOM content is loaded
document.addEventListener("DOMContentLoaded", changeBackgroundRed());
</script>
</head>
<body>
<h1>Change the background on load</h1>
</body>
</html>
The DOMContentLoaded
event is triggered when your whole HTML page has been loaded, even though some external JavaScript, CSS, and image resources are not yet fully loaded.
If you want to wait until the page and the resources are fully loaded, you can listen for the load
event instead.
Changing background color of a specific element
The document.body
code means you are selecting the <body>
tag of your HTML page. You can also change only a part of your page by selecting only that specific element.
The DOM provides several ways for you to select specific element:
getElementById()
- get a single element with matchingid
attributegetElementsByClassName()
- get elements with matchingclass
attributegetElementsByName()
- get elements with matchingname
attributegetElementsByTagName()
- get elements with matching tag name like"li"
for<li>
,"body"
for<body>
But the most recommended approach is to use getElementById()
because it’s the most specific of these methods. Out of the four methods, only getElementById()
will retrieve a single element.
The following example shows how you can change the background of <div>
element with id="user"
attribute:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>JavaScript change background color</title>
<script>
// change the background color of div user
function changeBackgroundUser() {
document.getElementById("user").style.background = "red";
}
</script>
</head>
<body>
<div id="user">
<h1>Change specific DIV background color</h1>
</div>
<div id="guest">
<h2>This element's parent DIV background won't be changed</h2>
</div>
<div id="stranger">
<h2>Neither this one</h2>
</div>
<button onclick="changeBackgroundUser();">Red</button>
</body>
</html>
Finally, you can put the backgroundColor
as a parameter to the changeBackground()
function so that you can have buttons that change the background to different colors:
<script>
// change the background color using the passed parameter
function changeBackground(bgColor) {
document.body.style.background = bgColor;
}
</script>
<button onclick="changeBackground('red');">Red</button>
<button onclick="changeBackground('green');">Green</button>
<button onclick="changeBackground('blue');">Blue</button>
And that’s how you change the background color of a webpage with JavaScript.