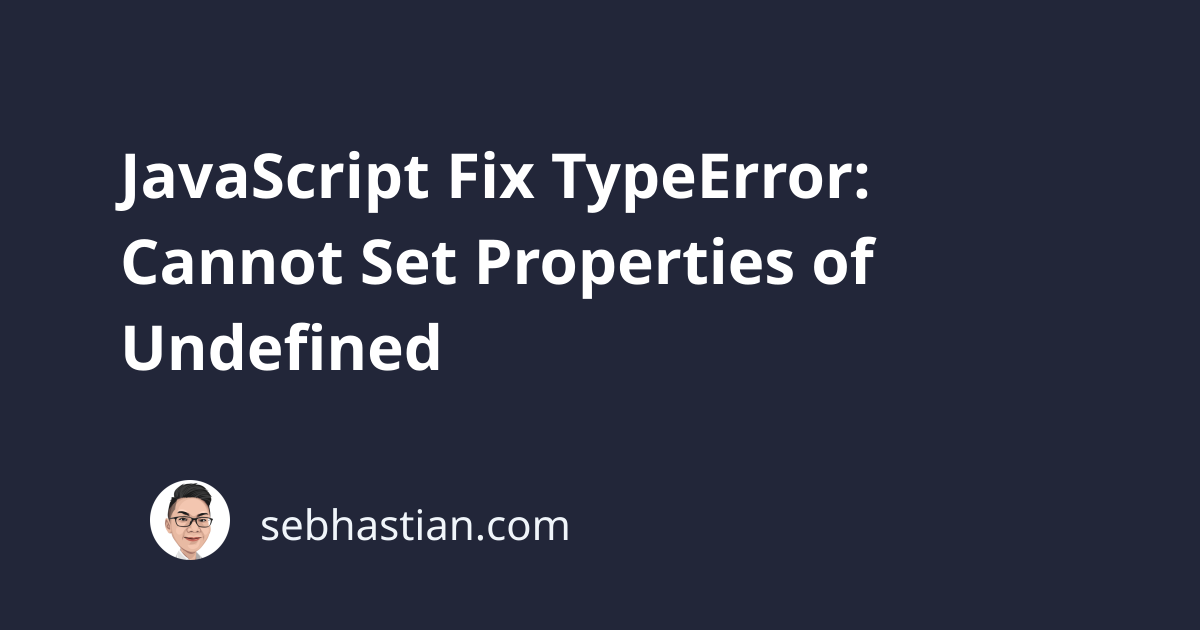
The error Uncaught TypeError: Cannot set properties of undefined (setting ‘x’) happens when you try to set a property of an undefined
value.
You probably mistake an undefined
variable for an object. For example, suppose you have a variable named user
as follows:
let user;
user.name = 'Nathan';
Here, we tried to set the property name
of the user
variable, but because the variable is never initialized, it has the value of undefined
, and we get this error as a result:
TypeError: Cannot set properties of undefined (setting 'name')
To prevent this error, you need to make sure that you’re setting a property of an object and not an undefined
value.
You can create an empty object using the curly brackets {}
literal:
let user = {}; // ✅
user.name = 'Nathan';
user['age'] = 29;
console.log(user);
Because the user
variable is now an empty object, you can set the name
property of the variable just fine.
You can use both the dot .
notation and the square brackets []
notation when setting the properties of an object.
And that’s how you fix the error. Hope this helps!