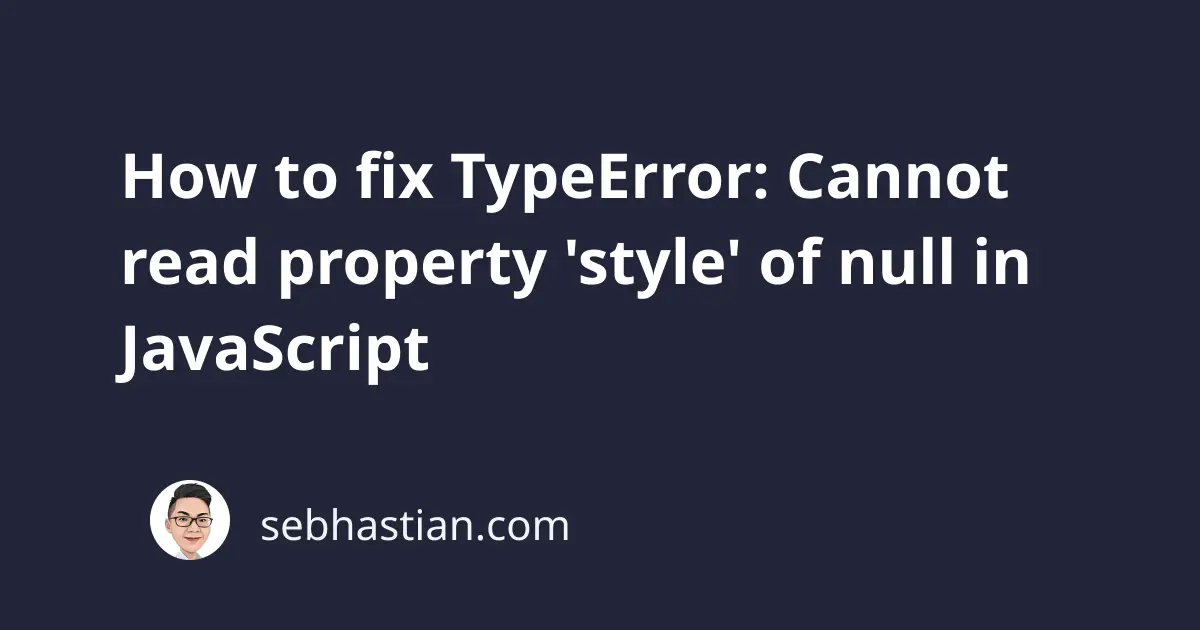
When you run JavaScript code, you might encounter the following error:
Uncaught TypeError: Cannot read property 'style' of null at x
This error occurs when you select an element that doesn’t exist in the Document Object Model (DOM).
To resolve this error, you need to check that the element exists and you are selecting the element after it has been loaded in the DOM.
This tutorial will show you an example that causes the error and how I fix it
How to reproduce the error
Suppose you have the following HTML element in your code:
<!DOCTYPE html>
<html>
<head></head>
<div id="green">
<h1>hello world!</h1>
</div>
<script>
document.getElementById("GREEN").style.color = "blue";
</script>
</html>
In the <script>
tag, you called the getElementById()
method and access the style
property of the returned element, changing the color
property to blue
.
But when you run the code, you get this error:
Uncaught TypeError: Cannot read property 'style' of null
at <anonymous>:2:37
This error occurs because the getElementById()
method returns null
when the element with a matching id
attribute isn’t found.
In this case, the id
attribute is green
with small letters instead of GREEN
which has capital letters.
To resolve this error, you need to change the argument when calling the getElementById()
method:
document.getElementById("green").style.color = "blue";
Or you can also assign the result of getElementById()
to a variable first and check if the result is not null
using an if
statement.
This way, you only access the .style.color
property when the element does exist:
let myElement = document.getElementById("green");
if (myElement) {
myElement.style.color = "blue";
}
When myElement
is null
, the if
block will be skipped, preventing the error.
Accessing the element before it has been loaded
Another cause for this error is when you try to access an element before it has been loaded.
In the example below, notice how the <script>
tag is placed before the <body>
tag:
<!DOCTYPE html>
<html>
<head></head>
<script>
document.getElementById("green").style.color = "blue";
</script>
<div id="green">
<h1>hello world!</h1>
</div>
</html>
Because the HTML is parsed from top to bottom, the <script>
tag above will run before the <body>
tag has been parsed. This will cause the same error.
To handle the error, you need to position the <script>
before the closing </body>
tag.
As an alternative, you can also add an event listener to the document
object to listen for the ‘DOMContentLoaded’ event:
<!DOCTYPE html>
<html>
<head></head>
<script>
document.addEventListener("DOMContentLoaded", () => {
document.getElementById("green").style.color = "blue";
});
</script>
<div id="green">
<h1>hello world!</h1>
</div>
</html>
This way, the getElementById()
will always be called only when the entire HTML page has been parsed to the DOM.
Conclusion
The error Uncaught TypeError: Cannot read property 'style' of null
occurs when you try to access the style
property of a null
object.
This commonly happens when you call a selector method like getElementById()
but the element isn’t found.
To fix this error, you need to ensure that the element exists in the DOM, and the JavaScript code is executed after the HTML page has been parsed to the DOM.
I hope this tutorial helps. See you in other tutorials! 👋