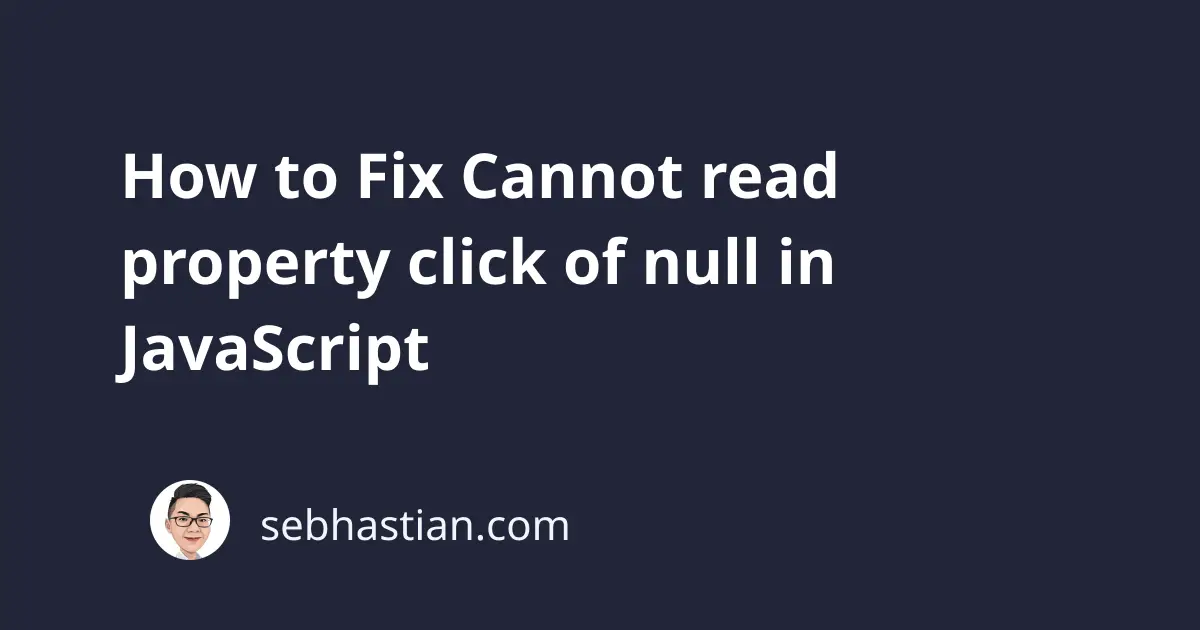
The TypeError: Cannot read property click of null occurs when you try to call the click()
method from the null object. Most likely, you’re trying to get a button element using JavaScript selector methods, but the element isn’t found in your HTML document, so the method returns null
.
When you call the click()
method from the value returned by the selector method, you get this error.
To show you an example, imagine you have an HTML document with the following contents:
<body>
<h1 id="header">Hello!</h1>
<button id="home-btn">Click Me!</button>
</body>
Here, you can see that the HTML document has a <button>
element with an id
attribute called ‘home-btn’.
Next, you tried to get the button using the getElementById()
method, and call the click()
method using JavaScript as shown below:
let btn = document.getElementById('btn');
btn.click();
Notice that the value passed to the getElementById()
method is ‘btn’, but the button in the document has an id value of ‘home-btn’. This causes JavaScript unable to find the button and return null
instead.
When you call the click()
button from the returned value, which is null
, the error happens:
Uncaught TypeError: Cannot read properties of null (reading 'click')
Uncaught TypeError: Cannot read property click of null
Both error messages above mean the same thing, the button isn’t found, and the null
object doesn’t have the click()
method, so JavaScript doesn’t know what to do.
To prevent this error, you need to make sure that you’re not calling the click()
method from a null
object.
In this case, the solution is quite clear: you need to specify the right id
value to the getElementById()
method:
let btn = document.getElementById('home-btn');
btn.click();
To prevent this error, you can also add the optional chaining ?.
operator when calling the click()
method. Here’s how you do that:
let btn = document.getElementById('home-btn');
btn?.click();
The optional chaining ensures that the btn
object is not null
or undefined
before calling the click()
method. This causes JavaScript to do nothing when the btn
object is null
, preventing the error from happening.
And that’s how you fix the TypeError: Cannot read property click of null in your code. See you in other tutorials!