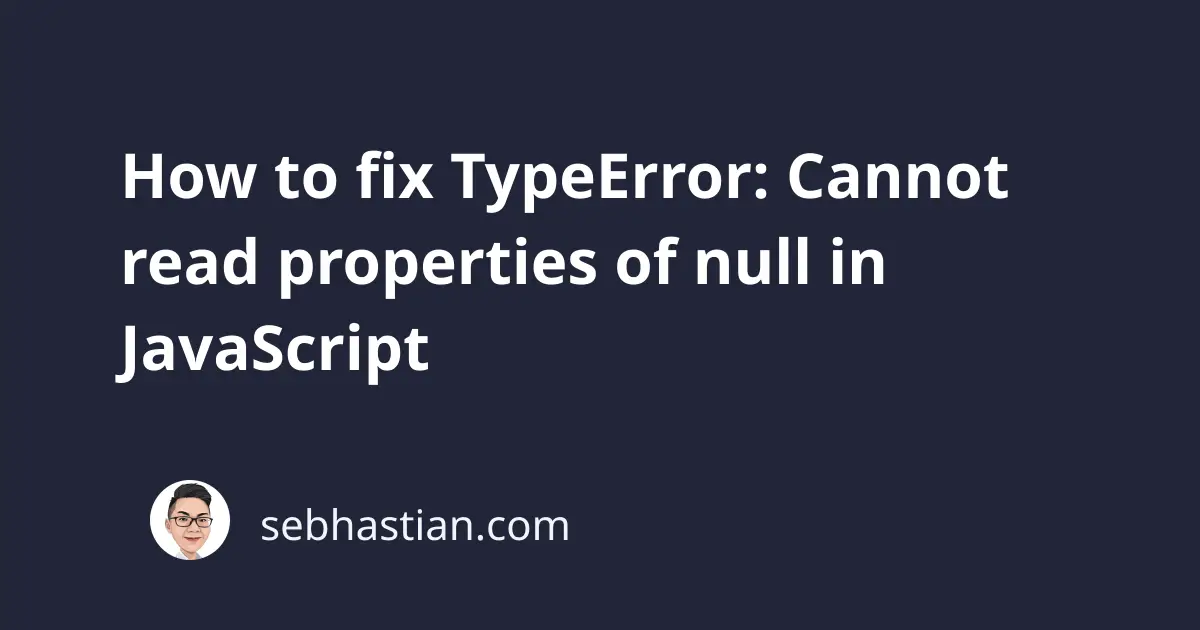
When running JavaScript code, you might encounter an error that says:
Uncaught TypeError: Cannot read properties of null (reading 'x')
This error occurs when you try to read a property of an object, but the object itself is actually null
so the property doesn’t exist.
Let me show you an example that causes this error and how I fix it.
How to reproduce this error
Suppose you write some JavaScript code to select an element from the DOM and read its value using the .value
property.
You might write code as follows:
let inputEl = document.getElementById("text-input");
let inputVal = inputEl.value;
The code above tries to fetch an element that has an id of text-input
. When the element isn’t found, then JavaScript will return null
.
In the second line, we tried to read the value
property of the inputEl
object. If the inputEl
object is null
, then the error occurs:
Uncaught TypeError: Cannot read properties of null (reading 'value')
Variations of this error might occur depending on what property you’re trying to access.
For example, you can also try to call the addEventListener()
method on a button object as follows:
let btnEl = document.getElementById("my-button");
console.log(btnEl); // null
btnEl.addEventListener("click", () => alert("You clicked a button!"));
When the getElementById()
method can’t find the button, it returns null
, so calling the addEventListener()
method generates the error:
Uncaught TypeError: Cannot read properties of null (reading 'addEventListener')
As you can see, calling a property from an element that doesn’t exist will cause this error.
How to fix this error
To resolve this error, you need to make sure that you’re not accessing properties of a null
object.
You can do so by adding an if
statement before accessing the property. For example:
let inputEl = document.getElementById("text-input");
if (inputEl) {
let inputVal = inputEl.value;
}
The if
statement above will check if the variable inputEl
is not null before running the code block.
This way, the value
property from the inputEl
object won’t be called when the inputEl
is null.
The same goes for the button element. As an alternative, you can also use the optional chaining operator ?.
as follows:
let btnEl = document.getElementById("my-button");
btnEl?.addEventListener("click", () => alert("You clicked a button!"));
This way, the addEventListener()
method is only called when the btnEl
variable isn’t null.
Sometimes, you also get this error even when the element exists on your page. If this is the case, then you need to make sure that you run the JavaScript code after the page content has been loaded.
Make sure that you place your <script>
tag before the closing </body>
tag as shown below:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Nathan Sebhastian</title>
</head>
<body>
<button id="btn">Sign up</button>
<!-- ✅ Script is placed before the closing body tag -->
<script>
let btnEl = document.getElementById("my-button");
btnEl?.addEventListener("click", () => alert("You clicked a button!"));
</script>
</body>
</html>
It doesn’t matter whether you have an inline or external script. You need to place it after the HTML elements.
Another way to ensure the script is executed after the content has been loaded is to listen for the DOMContentLoaded
event from the document
object as shown below:
document.addEventListener('DOMContentLoaded', () => {
let btnEl = document.getElementById("my-button");
btnEl?.addEventListener("click", () => alert("You clicked a button!"));
});
By wrapping your JavaScript code inside the addEventListener()
method for the DOMContentLoaded
event, you make sure that the code only gets executed after the content is loaded.
And that’s how you fix the TypeError: Cannot read properties of null when running JavaScript code.
I hope this tutorial helps. See you in other tutorials! 👋