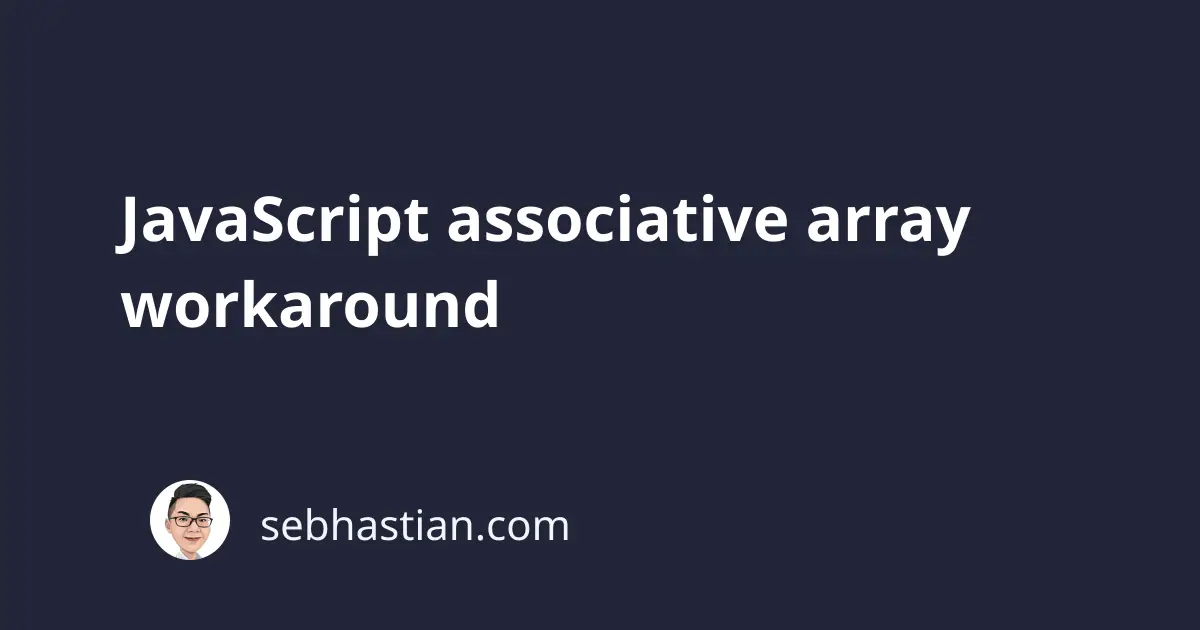
An associative array is an array that holds values in a key-value pair. A normal array uses numbered indices so that you can access its elements.
The following example shows how you can access the value stored in an array through its index:
let arr = ["Nathan", "Jack"];
console.log(arr[0]); // "Nathan"
console.log(arr[1]); // "Jack"
An associative array allows you to define a named key for each value so that instead of using a number as the index, you can use a string.
In other programming languages like PHP for example, an associative array can be created by writing the index name before the value as follows:
// This is PHP code
$age = array("first" => "Nathan", "second" => "Jack");
echo $age["first"]; // "Nathan"
echo $age["second"]; // "Jack"
Unfortunately, JavaScript doesn’t support creating associative arrays.
If you really need to create an array with named indices, you need to create a workaround by using JavaScript object type as follows:
let obj = { first: "Nathan", second: "Jack" };
console.log(obj["first"]); // "Nathan"
console.log(obj["second"]); // "Jack"
As you can see in the example above, the workaround for creating an associative array is simply by initializing a regular object.
You then access the object properties by using the objectName[propertyName]
syntax.
Since JavaScript object doesn’t have the .length
property, so you need to create a workaround to know the size of your associative array by using the Object.keys()
method.
The Object.keys()
method returns an array of a given object’s property name. You can then call the .length
property on the returned array. Here’s an example:
let obj = { first: "Nathan", second: "Jack" };
let size = Object.keys(obj).length;
console.log(size); // 2
Finally, you can use the for..in
loop statement to iterate through the properties of the object as follows:
let obj = { first: "Nathan", second: "Jack" };
for (const property in obj) {
console.log(`${property}: ${obj[property]}`);
}
The output of the code above will be as follows:
> "first: Nathan"
> "second: Jack"
And that’s how you can use JavaScript object data type as a workaround for creating an associative array.
I would recommend you to stick with the regular JavaScript array with numbered indices instead of creating a fake associative array with the object type.
But if you really have to use the associative array as the model type of your data, then this is the easiest way to create one.