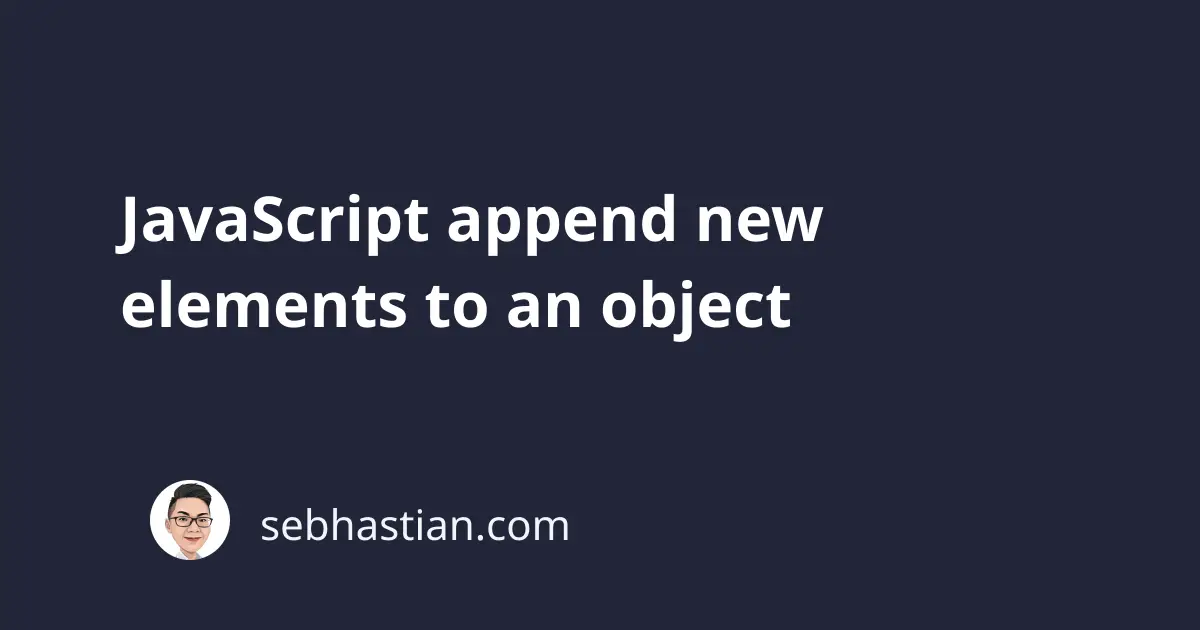
The way to append (or add) new elements to your JavaScript object depends on whether you are adding a new element to a parent Node object of a DOM tree or to a regular JavaScript object. This tutorial will show you how to append to both objects.
First, let’s see how you can append elements to a parent Node object
Append elements to a Node object
The Node object is a representation of HTML elements that you can select using the selector methods of the document
object. For example, suppose you have the following code on your HTML page:
<div id="app"></div>
You can select the <div>
tag using the following selector methods:
document.querySelector("#app"); // # means id
// OR
document.getElementById("app");
After selecting the object, you can append new elements to it using the append()
method. You can append any valid Document element (HTML tags) or even just a text as follows:
const header = document.createElement("h1"); // creates a <h1> element
header.textContent = "Hello World"; // add the text
document.getElementById("app").append(header);
You’ll see the <h1>
tag “Hello World” appended to the <div>
just like the code below:
<div id="app">
<h1>Hello World</h1>
</div>
The append()
method always adds new element next to the last child of the parent element, so appending two elements will cause the second one appended after the first:
const header = document.createElement("h1");
header.textContent = "Hello World";
const paragraph = document.createElement("p");
paragraph.textContent = "Nice weather today";
document.getElementById("app").append(header, paragraph);
The code above will generate the following HTML structure:
<div id="app">
<h1>Hello World</h1>
<p>Nice weather today</p>
</div>
The append()
method can accept many document elements as its argument.
And that’s how you append new elements to a Node object. Let’s see how you can append elements to a regular JavaScript object next.
Append to JavaScript object
When you need to append new elements to your JavaScript object variable, you can use either the Object.assign()
method or the spread operator. Let me show you an example of using both.
First, the Object.assign()
method will copy all properties that you defined in an object to another object (also known as source
and target
objects).
To use Object.assign()
, you need to pass one target
object and one or more source
objects:
Object.assign(target, source);
Knowing this, you can easily append new elements or properties to an object as follows:
let myObj = {
1: { name: "John" },
2: { name: "Susan" },
};
let newObj = Object.assign(myObj, { 3: { name: "Lucas" } });
console.log(newObj);
// { 1: { name: "John" }, 2: { name: "Susan" }, 3: { name: "Lucas" } }
Alternatively, you can also use the spread operator to combine two or more objects as follows:
let myObj = {
1: { name: "John" },
2: { name: "Susan" },
};
let newObj = { ...myObj, 3: { name: "Lucas" } };
console.log(newObj);
// { 1: { name: "John" }, 2: { name: "Susan" }, 3: { name: "Lucas" } }
And that’s how you can append new elements to objects using JavaScript.