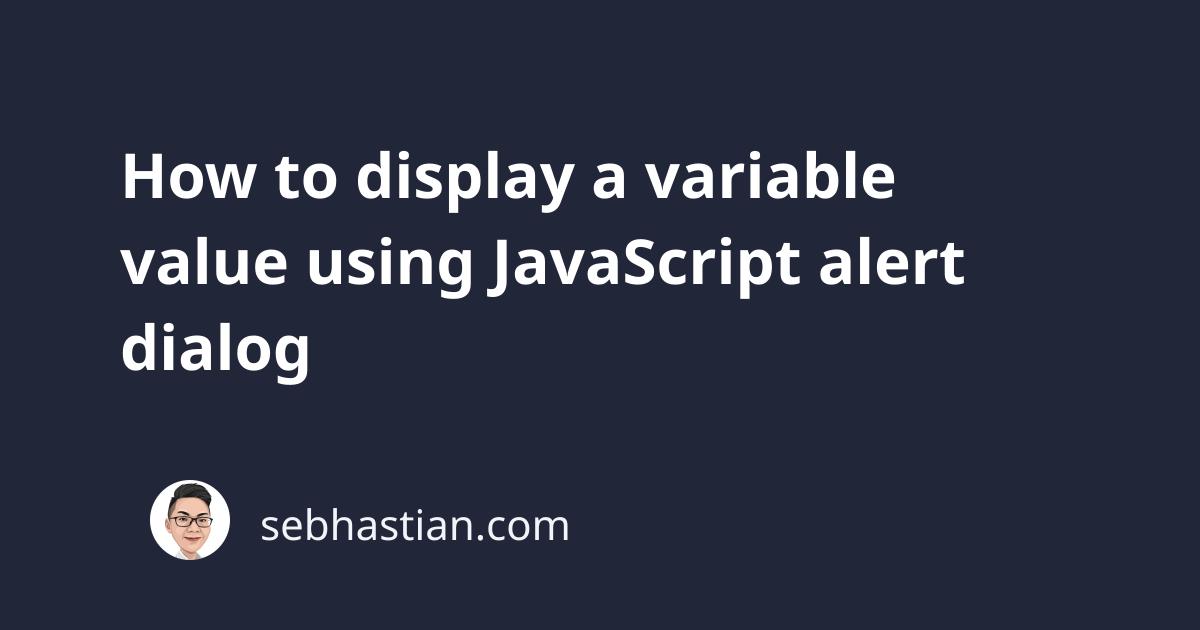
JavaScript provides you with the alert()
method that allows you to create an alert box, where you can display some information together with an OK button to remove the alert.
You can test the alert()
method by simply opening your browser’s console and type in alert()
as in the screenshot below:
The alert()
method is also useful for testing your JavaScript code and see if a certain variable holds the right value. For example, you may have the isMember
variable that contains true
if the user has logged in to your website and false
when the user is not.
You can use the alert()
method to print the value of the variable as follows:
const isMember = false;
alert(isMember);
The result would be as follows:
Be aware that the alert()
method can’t print an array of objects as follows:
const users = [
{
name: "Nathan",
},
{
name: "Daisy",
},
{
name: "Abby",
},
];
alert(users);
The above code will cause the alert box to display [Object object]
as in the screenshot below:
To prevent this issue, you need to transform the array of objects into a JSON string first using the JSON.stringify()
method:
const users = [
{
name: "Nathan",
},
{
name: "Daisy",
},
{
name: "Abby",
},
];
alert(JSON.stringify(users));
Now the alert box will display the values without any trouble:
And that’s how you can use the alert()
method to display variable values.
You can use the alert()
method to display multiple variables too, just like a normal string. It’s recommended for you to use template string to ember the variables in your alert box as follows:
const email = "[email protected]";
const username = "nathan";
const isMember = true;
alert(`Your values: \n
email: ${email} \n
username: ${username} \n
isMember: ${isMember} \n
You can use this alert as your template for displaying values`);
The result in the browser will be similar to this:
Feel free to use the alert()
call above as you require it 😉