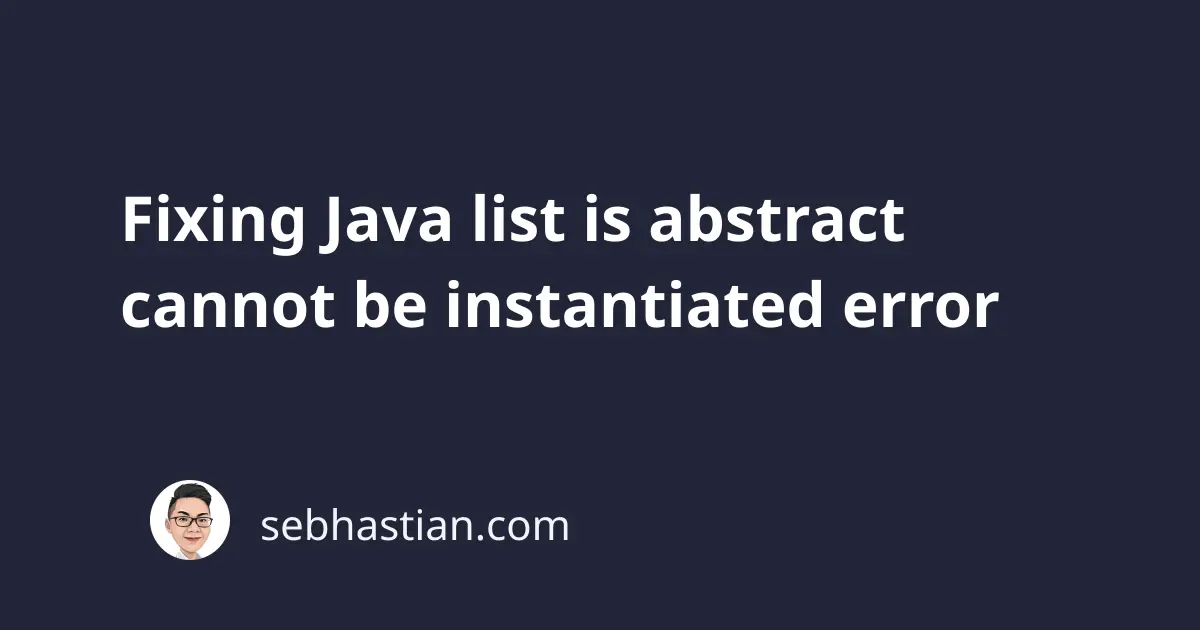
The List
type in Java is an Interface
type and not a Class
type.
If you try to create an instance of List
type, Java will throw an error as follows:
List myList = new List();
// ERROR: 'List' is abstract; cannot be instantiated
This is because all Interface
types in Java are abstract references used to group related methods and variables together.
When you need to create an instance of List
type, you need to instantiate from one of the classes that implement the type.
In Java, there are several classes that implement the List
type. They are:
ArrayList
LinkedList
Stack
Vector
CopyOnWriteArrayList
Below is an example of how to create a List
type instance. Note that you can instantiate the object using any class that implements the List
interface:
List myList = new ArrayList();
List secondList = new LinkedList();
List thirdList = new Stack();
The classes that implement a List
type are parameterized type classes.
These classes allow you to specify the type argument for the instances created from them.
For example, you can create a List
that only stores String
values:
List<String> myList = new ArrayList();
myList.add("Nathan");
myList.add("Hello");
Without specifying the type argument using <String>
as in the example above, the instance can store values of any type:
List myList = new ArrayList();
myList.add(1);
myList.add("String");
myList.add(true);
In Java, initializing an instance without specifying its type is known as raw types instantiation.
Generally, it’s not recommended to create raw type instances because you lose the type safety feature for these instances.
It’s better to define a List
with an explicit type, such as a List<Integer>
or List<String>
.
And that’s how you instantiate a List
type instance in Java. 😉