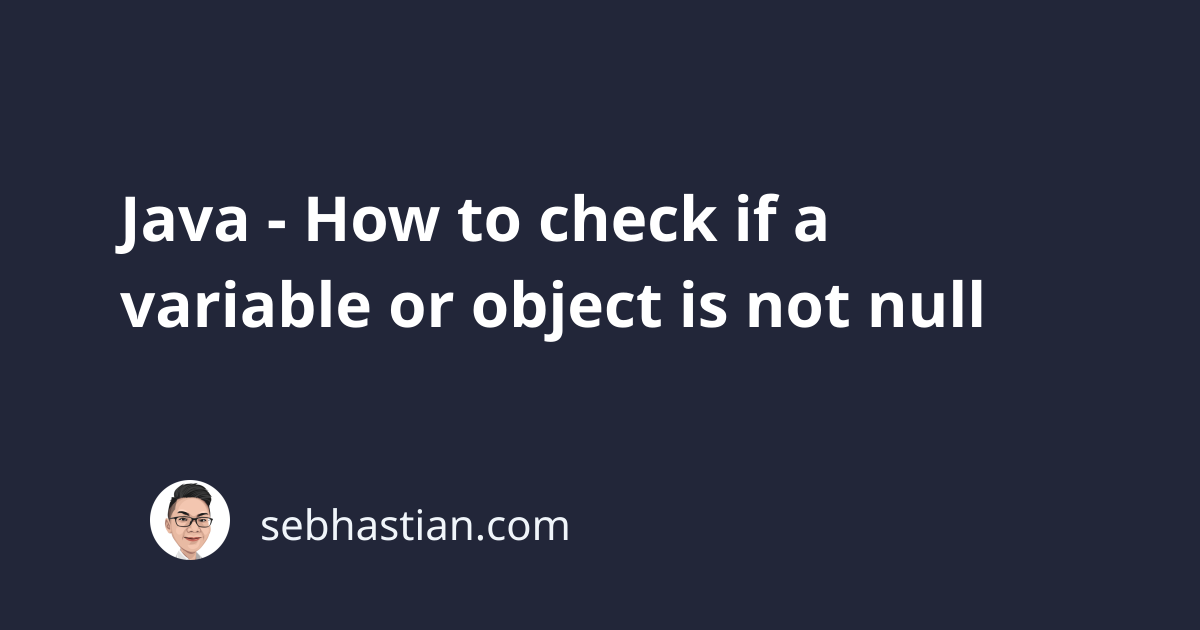
Java will throw the NullPointerException
error when you call a method on an object with the value of null
.
This frequently happens when you call methods from an object returned from a function as follows:
class Main {
public static void main(String[] myArgs) {
String response = getNull();
System.out.println(response.length()); // NullPointerException
}
private static String getNull() {
return null;
}
}
The call to the response.length()
in the code above is done on a String
variable with a null
value, causing Java to throw the NullPointerException
error:
Exception in thread "main" java.lang.NullPointerException
at Main.main(Main.java:4)
Process finished with exit code 1
To avoid the error and make the code run without any error, you can perform an if
check on the returned value response
and see if the value is null.
You can do so using the not equal operator (!=
) like this:
String response = getNull();
if(response != null){
System.out.println(response.length());
}
When the value of response
is not null
, then Java will run the println()
method inside the if
block above.
You can also use the Objects.nonNull()
method to check whether a variable is not null
.
You need to import the Objects
class and use the nonNull()
static method as follows:
import java.util.Objects;
// Inside Main class:
String response = getNull();
if(Objects.nonNull(response)){
System.out.println(response.length());
}
Finally, you can also add an else
condition to define code that will be executed when the result is null:
String response = getNull();
if(response != null){
System.out.println(response.length());
} else {
System.out.println("response is NULL!");
}
The full code for is not null check is as shown below:
class Main {
public static void main(String[] myArgs) {
String response = getNull();
if(response != null){
System.out.println(response.length());
} else {
System.out.println("response is NULL!");
}
}
private static String getNull() {
return null;
}
}
To summarize, you can check whether a variable or object is not null
in two ways:
- Using the not equal operator (
variable != null
) - Using the
Objects
classnonNull()
method
Checking for null
values before executing further operations will help you to avoid the NullPointerException
error.
And that’s how you check if a variable or object is not null
in Java. I hope this tutorial has been useful for you 🙏