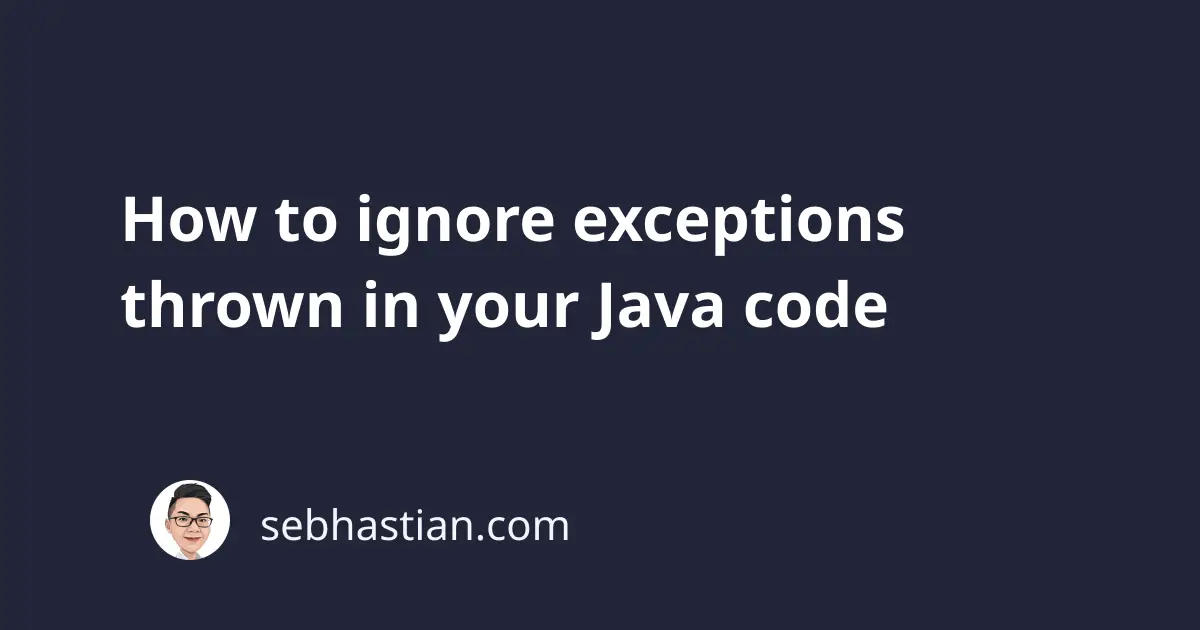
Sometimes, you may want to ignore an exception thrown by your Java program without stopping the program execution.
To ignore an exception in Java, you need to add the try...catch
block to the code that can throw an exception, but you don’t need to write anything inside the catch
block.
Let’s see an example of how to do this.
Suppose you have a checkAge()
method that checks whether the age
variable in your code is greater than 18
as follows:
static void checkAge(int age) throws Exception {
if (age < 18) {
throw new Exception( "Age must be greater than 18" );
}
}
In your main()
method, you can surround the call to the checkAge()
method with a try...catch
block.
The catch
block parameter should be named as ignored
to let Java know that this exception is ignored:
public static void main(String[] args) {
try {
checkAge(15);
} catch (Exception ignored) { }
System.out.println("Ignoring the exception");
System.out.println("Continue code as normal");
}
When the exception happens and the catch
block above is executed, nothing will be done by Java.
The exception is ignored and the next line of code below the catch
block will be executed as if nothing has happened.
The output:
Ignoring the exception
Continue code as normal
Process finished with exit code 0
When you have multiple exceptions to ignore, you need to put the try...catch
block in each of the exceptions as shown below:
try {
checkAge(12);
} catch (Exception ignored) { }
try {
checkAge(15);
} catch (Exception ignored) { }
try {
checkAge(16);
} catch (Exception ignored) { }
The code above gets redundant pretty fast. You can optimize the code by creating a wrapper method that has the try...catch
block ready for your runnable function.
You need to create a functional interface that serves as the type of the method you want to ignore.
Consider the ignoreExc()
function below:
static void ignoreExc(Runnable r) {
try {
r.run();
} catch (Exception ignored) { }
}
@FunctionalInterface
interface Runnable {
void run() throws Exception;
}
The call to run()
method inside the ignoreExc()
function will execute the Runnable
you passed into the method.
Finally, you only need to use the ignoreExc()
function to call your actual method with a lambda expression as follows:
public static void main(String[] args) {
ignoreExc(() -> checkAge(16));
ignoreExc(() -> checkAge(17));
ignoreExc(() -> checkAge(18));
System.out.println("Ignoring the exception");
System.out.println("Continue code as normal");
}
The checkAge()
method exceptions will be ignored because there’s nothing in the catch
block of the ignoreExc()
function.
With the ignoreExc()
function, you’ve reduced the need to wrap your code with the try...catch
block each time you want to ignore an exception.
Here’s the full code for ignoring exceptions in Java:
class Main {
public static void main(String[] args) {
ignoreExc(() -> checkAge(16));
ignoreExc(() -> checkAge(17));
ignoreExc(() -> checkAge(18));
System.out.println("Ignoring the exception");
System.out.println("Continue code as normal");
}
static void checkAge(int age) throws Exception {
if (age < 18) {
throw new Exception("Age must be greater than 18");
}
}
static void ignoreExc(Runnable r) {
try {
r.run();
} catch (Exception ignored) { }
}
@FunctionalInterface
interface Runnable {
void run() throws Exception;
}
}
Now you’ve learned how to ignore exceptions thrown by a Java program.
Feel free to use the code in this tutorial for your project. 👍